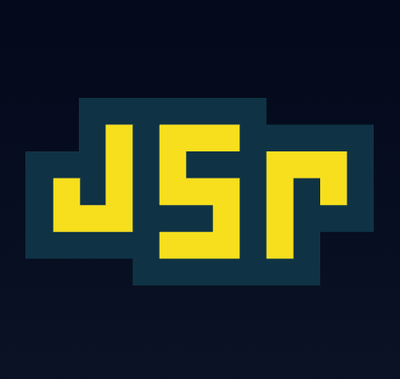
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@sindresorhus/is
Advanced tools
The @sindresorhus/is npm package is a type-checking library that provides a variety of functions to assert the type of a given value. It can be used to check primitive types, built-in objects, and to perform environment checks.
Primitive type checking
Check if a value is a string.
const is = require('@sindresorhus/is');
console.log(is.string('Hello World')); // true
Built-in object checking
Check if a value is a Promise.
const is = require('@sindresorhus/is');
console.log(is.promise(new Promise(resolve => resolve()))); // true
Environment checking
Check if the current environment is Node.js.
const is = require('@sindresorhus/is');
console.log(is.nodejs); // true or false depending on the environment
Array checking
Check if a value is an Array.
const is = require('@sindresorhus/is');
console.log(is.array([1, 2, 3])); // true
Object checking
Check if a value is an Object.
const is = require('@sindresorhus/is');
console.log(is.object({foo: 'bar'})); // true
Type-check offers a more granular and customizable approach to type checking, allowing users to define custom type expressions and check values against them. It is more flexible but also more complex than @sindresorhus/is.
Check-types is a library that provides a set of predicates for type checking. It is similar to @sindresorhus/is but offers a slightly different API and additional functions for assertions and throwing errors.
Type check values:
is.string('🦄') //=> true
$ npm install @sindresorhus/is
const is = require('@sindresorhus/is');
is('🦄');
//=> 'string'
is(new Map());
//=> 'Map'
is.number(6);
//=> true
Returns the type of value
.
Primitives are lowercase and object types are camelcase.
Example:
'undefined'
'null'
'string'
'symbol'
'Array'
'Function'
'Object'
Note: It will throw if you try to feed it object-wrapped primitives, as that's a bad practice. For example new String('foo')
.
All the below methods accept a value and returns a boolean for whether the value is of the desired type.
Keep in mind that functions are objects too.
Returns true
for any object with a .then()
and .catch()
method. Prefer this one over .nativePromise()
as you usually want to allow userland promise implementations too.
Returns true
for any object that implements its own .next()
and .throw()
methods and has a function definition for Symbol.iterator
.
JavaScript primitives are as follows: null
, undefined
, string
, number
, boolean
, symbol
.
An object is plain if it's created by either {}
, new Object()
, or Object.create(null)
.
Returns true
for instances created by a ES2015 class.
Check if value
(number) is in the given range
. The range is an array of two values, lower bound and upper bound, in no specific order.
is.inRange(3, [0, 5]);
is.inRange(3, [5, 0]);
is.inRange(0, [-2, 2]);
Check if value
(number) is in the range of 0
to upperBound
.
is.inRange(3, 10);
Returns true
if value
is a DOM Element.
Check if value
is Infinity
or -Infinity
.
Returns true
if value
is falsy or an empty string, array, object, map, or set.
Returns true
if any of the input values
returns true in the predicate
:
is.any(is.string, {}, true, '🦄');
//=> true
is.any(is.boolean, 'unicorns', [], new Map());
//=> false
Returns true
if all of the input values
returns true in the predicate
:
is.all(is.object, {}, new Map(), new Set());
//=> true
is.all(is.string, '🦄', [], 'unicorns');
//=> false
There are hundreds of type checking modules on npm, unfortunately, I couldn't find any that fit my needs:
For the ones I found, pick 3 of these.
The most common mistakes I noticed in these modules was using instanceof
for type checking, forgetting that functions are objects, and omitting symbol
as a primitive.
MIT
FAQs
Type check values
The npm package @sindresorhus/is receives a total of 12,751,568 weekly downloads. As such, @sindresorhus/is popularity was classified as popular.
We found that @sindresorhus/is demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.