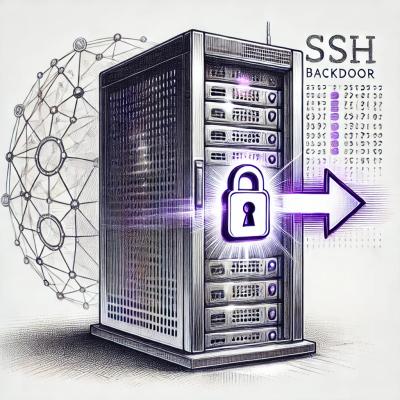
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@singlestore/ai
Advanced tools
A module that enhances the `@singlestore/client` package with AI functionality, allowing you to integrate advanced AI features like embeddings and chat completions.
A module that enhances the @singlestore/client
package with AI functionality, allowing you to integrate advanced AI features like embeddings and chat completions.
To install the @singlestore/ai
package, run the following command:
npm install @singlestore/ai
First, create an instance of the SingleStoreAI
class using your OpenAI API key.
import { AI } from "@singlestore/ai";
const ai = new SingleStoreAI({ openAIApiKey: "<OPENAI_API_KEY>" });
Generate embeddings for a given input text using the create
method.
const input = "Hi!";
const embeddings = await ai.embeddings.create(input);
console.log(embeddings);
Create a chat completion.
const prompt = "Hi, how are you?";
const chatCompletion = await ai.chatCompletions.create({
prompt,
model: "gpt-4o",
systemRole: "You are a helpful assistant",
});
console.log(chatCompletion);
Stream chat completions to handle responses in real time.
const prompt = "Hi, how are you?";
const stream = await ai.chatCompletions.create({
prompt,
model: "gpt-4o",
systemRole: "You are a helpful assistant",
stream: true,
});
const onChunk: OnChatCompletionChunk = (chunk) => {
console.log("onChunk:", chunk);
};
const chatCompletion = await ai.chatCompletions.handleStream(stream, onChunk);
console.log(chatCompletion);
Create a custom chat completion tool to handle specific tasks.
import { AI, ChatCompletionTool } from "@singlestore/ai";
import { z } from "zod";
const findCityInfoTool = new ChatCompletionTool({
name: "find_city_info",
description: "Useful for finding and displaying information about a city.",
params: z.object({ name: z.string().describe("The city name") }),
call: async (params) => {
const info = `${params.name} is known as a great city!`;
return { name: "find_city_info", params, value: JSON.stringify(info) };
},
});
const ai = new AI({
openAIApiKey: "<OPENAI_API_KEY>",
chatCompletionTools: [findCityInfoTool],
});
const chatCompletion = await ai.chatCompletions.create({ prompt: "Find info about Vancouver." });
console.log(chatCompletion);
Extend the ChatCompletions class to use a custom LLM for creating chat completions.
import { AI, type AnyChatCompletionTool, ChatCompletions } from "@singlestore/ai";
class CustomChatCompletionsManager<
TChatCompletionTool extends AnyChatCompletionTool[] | undefined,
> extends ChatCompletions<TChatCompletionTool> {
constructor() {
super();
}
getModels(): Promise<string[]> | string[] {
// Your implementation
return [];
}
async create<TStream extends boolean | undefined>(
params: CreateChatCompletionParams<TStream, TChatCompletionTool>,
): Promise<CreateChatCompletionResult<TStream>> {
// Your implementation
return {} as CreateChatCompletionResult<TStream>;
}
}
const ai = new AI({
openAIApiKey: "<OPENAI_API_KEY>",
chatCompletions: new CustomChatCompletionsManager(),
});
Create a custom embeddings class to use a custom LLM for creating embeddings.
import { AI, Embeddings } from "@singlestore/ai";
class CustomEmbeddingsManager extends Embeddings {
constructor() {
super();
}
getModels(): string[] {
// Your implementation
return [];
}
async create(input: string | string[], params?: CreateEmbeddingsParams): Promise<Embedding[]> {
// Your implementation
return [];
}
}
const ai = new AI({
openAIApiKey: "<OPENAI_API_KEY>",
embeddings: new CustomEmbeddingsManager(),
});
FAQs
A module that enhances the [`@singlestore/client`](https://github.com/singlestore-labs/singlestore/tree/main/packages/client) package with AI functionality, allowing you to integrate AI features like embeddings and chat completions.
We found that @singlestore/ai demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.