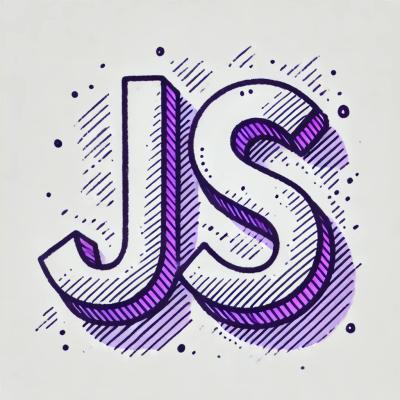
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
@smithy/util-retry
Advanced tools
@smithy/util-retry is a utility package designed to provide retry functionality for operations that may fail and need to be retried. It is part of the Smithy framework, which is used by AWS SDK for JavaScript. This package helps in implementing retry strategies, handling transient errors, and ensuring that operations are retried according to specified policies.
Standard Retry Strategy
This feature allows you to use a standard retry strategy for operations that might fail. The `StandardRetryStrategy` class provides a simple way to retry operations with a default policy.
const { StandardRetryStrategy } = require('@smithy/util-retry');
const retryStrategy = new StandardRetryStrategy();
async function fetchData() {
try {
const result = await retryStrategy.retry(() => {
// Your operation that might fail
return fetch('https://api.example.com/data');
});
console.log('Data fetched successfully:', result);
} catch (error) {
console.error('Failed to fetch data:', error);
}
}
fetchData();
Custom Retry Strategy
This feature allows you to create a custom retry strategy by extending the `StandardRetryStrategy` class. You can override the `shouldRetry` method to implement custom logic for determining whether an operation should be retried.
const { StandardRetryStrategy } = require('@smithy/util-retry');
class CustomRetryStrategy extends StandardRetryStrategy {
shouldRetry(error) {
// Custom logic to determine if the operation should be retried
return error.statusCode === 500;
}
}
const customRetryStrategy = new CustomRetryStrategy();
async function fetchData() {
try {
const result = await customRetryStrategy.retry(() => {
// Your operation that might fail
return fetch('https://api.example.com/data');
});
console.log('Data fetched successfully:', result);
} catch (error) {
console.error('Failed to fetch data:', error);
}
}
fetchData();
Exponential Backoff
This feature allows you to implement exponential backoff for retrying operations. By providing a custom `delayDecider` function, you can control the delay between retries, increasing it exponentially with each attempt.
const { StandardRetryStrategy } = require('@smithy/util-retry');
const retryStrategy = new StandardRetryStrategy({
delayDecider: (delayBase, attempts) => Math.pow(2, attempts) * delayBase
});
async function fetchData() {
try {
const result = await retryStrategy.retry(() => {
// Your operation that might fail
return fetch('https://api.example.com/data');
});
console.log('Data fetched successfully:', result);
} catch (error) {
console.error('Failed to fetch data:', error);
}
}
fetchData();
The `retry` package provides a comprehensive set of tools for retrying operations in Node.js. It allows for customizable retry strategies, including exponential backoff and custom retry logic. Compared to @smithy/util-retry, `retry` is more general-purpose and not specifically tied to the AWS SDK.
The `promise-retry` package is designed for retrying promise-based operations. It supports customizable retry strategies and integrates well with async/await syntax. Like `retry`, it is a general-purpose library and not specific to the AWS SDK, making it a versatile choice for various use cases.
The `async-retry` package provides a simple and flexible way to retry asynchronous operations. It supports custom retry strategies and integrates seamlessly with async/await. It is similar to @smithy/util-retry in terms of functionality but is not tied to the AWS SDK, making it suitable for a broader range of applications.
This package provides shared utilities for retries.
By default, each client already has a default retry strategy. The default retry count is 3, and only retryable errors will be retried.
AWS Documentation: Retry behavior.
import { S3Client } from "@aws-sdk/client-s3";
const client = new S3Client({}); // default retry strategy included.
If you want to change the number of attempts, you can provide maxAttempts
configuration during client creation.
import { S3Client } from "@aws-sdk/client-s3";
const client = new S3Client({ maxAttempts: 4 });
This is recommended because the StandardRetryStrategy
includes backoff calculation,
deciding whether an error should be retried, and a retry token counter.
If you want to change the number of attempts and use a custom delay
computation, you can use the ConfiguredRetryStrategy
from @smithy/util-retry
.
import { S3Client } from "@aws-sdk/client-s3";
import { ConfiguredRetryStrategy } from "@smithy/util-retry";
const client = new S3Client({
retryStrategy: new ConfiguredRetryStrategy(
4, // max attempts.
(attempt: number) => 100 + attempt * 1000 // backoff function.
),
});
This example sets the backoff at 100ms plus 1s per attempt.
If you provide both maxAttempts
and retryStrategy
, the retryStrategy
will
get precedence as it's more specific.
import { S3Client } from "@aws-sdk/client-s3";
import { ConfiguredRetryStrategy } from "@smithy/util-retry";
const client = new S3Client({
maxAttempts: 2, // ignored.
retryStrategy: new ConfiguredRetryStrategy(
4, // used.
(attempt: number) => 100 + attempt * 1000 // backoff function.
),
});
You can implement the RetryStrategyV2
interface.
Source: https://github.com/awslabs/smithy-typescript/blob/main/packages/types/src/retry.ts API Docs: https://docs.aws.amazon.com/AWSJavaScriptSDK/v3/latest/Package/-smithy-types/Interface/RetryStrategyV2/
FAQs
Shared retry utilities to be used in middleware packages.
The npm package @smithy/util-retry receives a total of 14,772,915 weekly downloads. As such, @smithy/util-retry popularity was classified as popular.
We found that @smithy/util-retry demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.