@snaplet/copycat
Advanced tools
Comparing version 1.1.0-rc.1 to 1.1.0
@@ -22,4 +22,6 @@ export * from './primitives'; | ||
export * from './scramble'; | ||
export * from './hash'; | ||
export * from './oneOfString'; | ||
export { generateHashKey, setHashKey } from './hash'; | ||
export { unique } from './unique'; | ||
export { uniqueByInput } from './uniqueByInput'; | ||
//# sourceMappingURL=copycat.d.ts.map |
@@ -6,2 +6,6 @@ "use strict"; | ||
var __hasOwnProp = Object.prototype.hasOwnProperty; | ||
var __export = (target, all) => { | ||
for (var name in all) | ||
__defProp(target, name, { get: all[name], enumerable: true }); | ||
}; | ||
var __copyProps = (to, from, except, desc) => { | ||
@@ -18,2 +22,8 @@ if (from && typeof from === "object" || typeof from === "function") { | ||
var copycat_exports = {}; | ||
__export(copycat_exports, { | ||
generateHashKey: () => import_hash.generateHashKey, | ||
setHashKey: () => import_hash.setHashKey, | ||
unique: () => import_unique.unique, | ||
uniqueByInput: () => import_uniqueByInput.uniqueByInput | ||
}); | ||
module.exports = __toCommonJS(copycat_exports); | ||
@@ -41,3 +51,12 @@ __reExport(copycat_exports, require("./primitives"), module.exports); | ||
__reExport(copycat_exports, require("./scramble"), module.exports); | ||
__reExport(copycat_exports, require("./hash"), module.exports); | ||
__reExport(copycat_exports, require("./oneOfString"), module.exports); | ||
var import_hash = require("./hash"); | ||
var import_unique = require("./unique"); | ||
var import_uniqueByInput = require("./uniqueByInput"); | ||
// Annotate the CommonJS export names for ESM import in node: | ||
0 && (module.exports = { | ||
generateHashKey, | ||
setHashKey, | ||
unique, | ||
uniqueByInput | ||
}); |
@@ -1,3 +0,7 @@ | ||
export declare const setHashKey: (key: string | import("fictional").HashKey) => void; | ||
export declare const generateHashKey: (input: string) => import("fictional").HashKey; | ||
import { HashKey } from 'fictional'; | ||
export declare function getHashKey(): string | HashKey; | ||
declare function setKey(key: string | HashKey): void; | ||
export declare const setHashKey: typeof setKey; | ||
export declare const generateHashKey: (input: string) => HashKey; | ||
export {}; | ||
//# sourceMappingURL=hash.d.ts.map |
@@ -22,2 +22,3 @@ "use strict"; | ||
generateHashKey: () => generateHashKey, | ||
getHashKey: () => getHashKey, | ||
setHashKey: () => setHashKey | ||
@@ -27,2 +28,12 @@ }); | ||
var import_fictional = require("fictional"); | ||
const hashKey = { | ||
value: import_fictional.hash.generateKey("chinochinochino!") | ||
}; | ||
function getHashKey() { | ||
return hashKey.value; | ||
} | ||
function setKey(key) { | ||
hashKey.value = key; | ||
return import_fictional.hash.setKey(key); | ||
} | ||
function derive16CharacterString(input) { | ||
@@ -41,3 +52,3 @@ const base = 33; | ||
} | ||
const setHashKey = import_fictional.hash.setKey; | ||
const setHashKey = setKey; | ||
const generateHashKey = (input) => { | ||
@@ -49,3 +60,4 @@ return input.length === 16 ? import_fictional.hash.generateKey(input) : import_fictional.hash.generateKey(derive16CharacterString(input)); | ||
generateHashKey, | ||
getHashKey, | ||
setHashKey | ||
}); |
@@ -1,2 +0,41 @@ | ||
export declare const phoneNumber: (input: import("fictional").JSONSerializable) => string; | ||
import { Input } from './types'; | ||
type PhoneNumberOptions = { | ||
/** | ||
* An array of prefixes to use when generating a phone number. | ||
* Can be used to generate a fictional phone number instead of a random one. | ||
* Using fictional phone numbers might make the generation slower. And might increase likelihood of collisions. | ||
* @example | ||
* ```ts | ||
* phoneNumber(seed, { | ||
* // Generate a French phone number within fictional phone number delimited range (cf: https://en.wikipedia.org/wiki/Fictitious_telephone_number) | ||
* prefixes: ['+3319900', '+3326191', '+3335301'], | ||
* // A french phone number is 11 digits long (including the prefix) so there is no need to generate a number longer than 4 digits | ||
* min: 1000, max: 9999 | ||
* }) | ||
* ``` | ||
* @example | ||
* | ||
* ```ts | ||
* phoneNumber(seed, { | ||
* // Generate a New Jersey fictional phone number | ||
* prefixes: ['+201555'], | ||
* min: 1000, max: 9999 | ||
* }) | ||
* ``` | ||
* @default undefined | ||
*/ | ||
prefixes?: Array<string>; | ||
/** | ||
* The minimum number to generate. | ||
* @default 10000000000 | ||
*/ | ||
min?: number; | ||
/** | ||
* The maximum number to generate. | ||
* @default 999999999999999 | ||
*/ | ||
max?: number; | ||
}; | ||
export declare const phoneNumber: (input: Input, options?: PhoneNumberOptions) => string; | ||
export {}; | ||
//# sourceMappingURL=phoneNumber.d.ts.map |
@@ -25,7 +25,17 @@ "use strict"; | ||
var import_fictional = require("fictional"); | ||
const phoneNumber = (0, import_fictional.join)("", [ | ||
"+", | ||
(0, import_fictional.times)([2, 3], import_fictional.char.digit), | ||
(0, import_fictional.times)([10, 12], import_fictional.char.digit) | ||
]); | ||
const phoneNumber = (input, options = { min: 1e10, max: 999999999999999 }) => { | ||
const min = options.min ?? 1e10; | ||
const max = options.max ?? 999999999999999; | ||
if (options.prefixes) { | ||
const prefix = options.prefixes.length > 1 ? (0, import_fictional.oneOf)(input, options.prefixes) : options.prefixes[0]; | ||
const prefixLength = prefix.length; | ||
const adjustedMin = Math.max(min, 10 ** (10 - prefixLength)); | ||
const adjustedMax = Math.min(max, 10 ** (15 - prefixLength) - 1); | ||
return `${prefix}${(0, import_fictional.int)(input, { | ||
min: adjustedMin, | ||
max: adjustedMax | ||
})}`; | ||
} | ||
return `+${(0, import_fictional.int)(input, { min, max })}`; | ||
}; | ||
// Annotate the CommonJS export names for ESM import in node: | ||
@@ -32,0 +42,0 @@ 0 && (module.exports = { |
{ | ||
"name": "@snaplet/copycat", | ||
"version": "1.1.0-rc.1", | ||
"version": "1.1.0", | ||
"description": "", | ||
@@ -5,0 +5,0 @@ "main": "dist/index.js", |
@@ -353,4 +353,8 @@ # 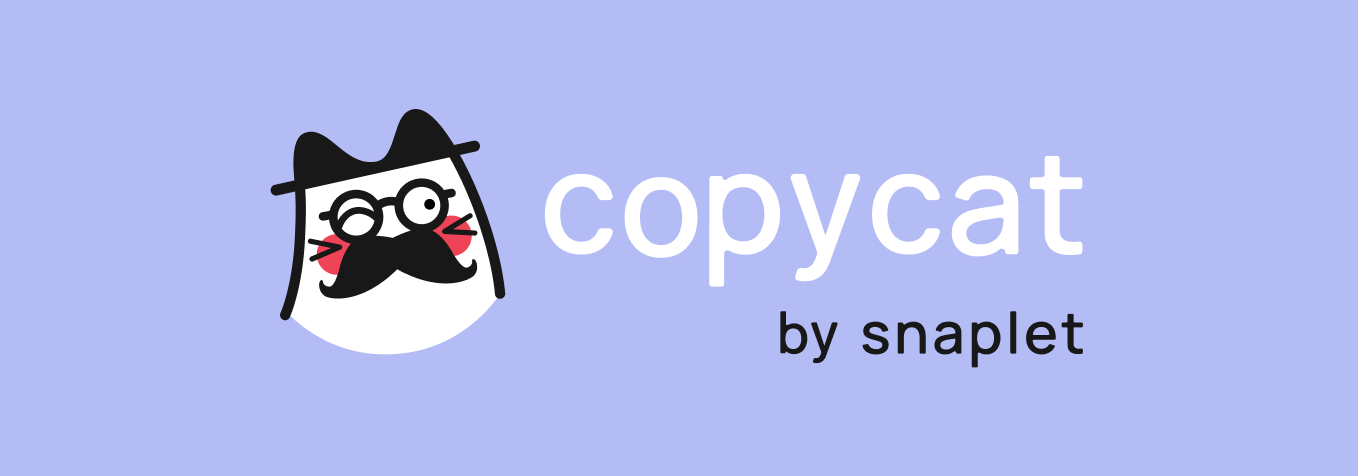 | ||
copycat.phoneNumber('foo') | ||
// => '+18312203332869' | ||
// => '+208438699696662' | ||
``` | ||
```js | ||
copycat.phoneNumber('foo', { prefixes: ['+3319900', '+3363998'], min: 1000, max: 9999 }) | ||
// => '+33639987662' | ||
``` | ||
@@ -360,2 +364,7 @@ **note** The strings _resemble_ phone numbers, but will not always be valid. For example, the country dialing code may not exist, or for a particular country, the number of digits may be incorrect. Please let us know if you need valid | ||
#### `options` | ||
- **`min=10000000000`:** Constrain generated values to be greater than or equal to `min` allow to control the minimum number of digits in the phone number | ||
- **`max=999999999999999`:** Constrain generated values to be less than or equal to `max` allow to control the maximum number of digits in the phone number | ||
- **`prefixes`:** An array of strings that should be used as prefixes for the generated phone numbers. Allowing to control the country dialing code. | ||
### `copycat.username(input)` | ||
@@ -373,2 +382,86 @@ | ||
### `copycat.unique(input, method, store, options)` | ||
The `unique` function is tailored to maintain uniqueness of values after they have undergone a specific transformation. | ||
This method is especially useful when the transformed values need to be unique, regardless of whether the input values are identical. | ||
It will do so by trying to generate a new value multiples times (up to attempts time) until it finds a unique one. | ||
**note** This method will try its best to generate unique values, but be aware of these limitations: | ||
1. The uniqueness is not guaranteed, but the probability of generating a duplicate is lower as the number of attempts increases. | ||
2. On the contrary of the other methods, the `unique` method is not stateless. It will store the generated values in the `store` object to ensure uniqueness. | ||
Meaning that the deterministic property over input is not guaranteed anymore. Now the determinism is based over a combination of: | ||
- the `input` value | ||
- the state of the `store` object | ||
- the number of `attempts` | ||
3. The `unique` method as it alter the global copycat hashKey between attemps before restoring the original one, it is not thread safe. | ||
4. If duplicates exists in the passed `input` accross calls, the method might hide those duplicates by generating a unique value for each of them. | ||
If you want to ensure duplicate value for duplicate input you should use the `uniqueByInput` method. | ||
#### `parameters` | ||
- **`input`** (_Input_): The seed input for the generation method. | ||
- **`method`** (_Function_): A deterministic function that takes `input` and returns a value of type `T`. | ||
- **`store`** (_Store<T>_): A store object to track generated values and ensure uniqueness. It must have `has(value: T): boolean` and `add(value: T): void` methods. | ||
- **`options`** (_UniqueOptions_): An optional configuration object for additional control. | ||
#### `options` | ||
- **`attempts`** (_number_): The maximum number of attempts to generate a unique value. Defaults to 10. | ||
- **`attemptsReached`** (_Function_): An optional callback function that is called when the maximum number of attempts is reached. | ||
```js | ||
// Define a method to generate a value | ||
const generateValue = (seed) => { | ||
return copycat.int(seed, { max: 3 }); | ||
}; | ||
// Create a store to track unique values | ||
const store = new Set(); | ||
// Use the unique method to generate a unique number | ||
copycat.unique('exampleSeed', generateValue, store); | ||
// => 3 | ||
copycat.unique('exampleSeed1', generateValue, store); | ||
// => 1 | ||
copycat.unique('exampleSeed', generateValue, store); | ||
// => 0 | ||
``` | ||
### `copycat.uniqueByInput(input, method, inputStore, resultStore, options)` | ||
The `uniqueByInput` function is designed to generate unique values while preserving duplicates for identical inputs. | ||
It is particularly useful in scenarios where input consistency needs to be maintained alongside the uniqueness of the transformed values. | ||
- **Preserving Input Duplication**: If the same input is provided multiple times, `uniqueByInput` ensures that the transformed value is consistently the same for each occurrence of that input. | ||
- **Uniqueness Preservation**: For new and unique inputs, `uniqueByInput` employs the `unique` method to generate distinct values, avoiding duplicates in the `resultStore`. | ||
#### `parameters` | ||
- **`input`** (_Input_): The seed input for the generation method. | ||
- **`method`** (_Function_): A deterministic function that takes `input` and returns a value of type `T`. | ||
- **`inputStore`** (_Store_): A store object to track the inputs and ensure consistent output for duplicate inputs. | ||
- **`resultStore`** (_Store_): A store object to track the generated values and ensure their uniqueness. | ||
- **`options`** (_UniqueOptions_): An optional configuration object for additional control. | ||
#### `options` | ||
- **`attempts`** (_number_): The maximum number of attempts to generate a unique value after transformation. Defaults to 10. | ||
- **`attemptsReached`** (_Function_): An optional callback function that is invoked when the maximum number of attempts is reached. | ||
```js | ||
// Define a method to generate a value | ||
const method = (seed) => { | ||
return copycat.int(seed, { max: 3 }); | ||
}; | ||
// Create stores to track unique values and inputs | ||
const resultStore = new Set(); | ||
const inputStore = new Set(); | ||
// Generate a unique number or retrieve the existing one for duplicate input | ||
copycat.uniqueByInput('exampleSeed', method, inputStore, resultStore); | ||
// => 3 | ||
copycat.uniqueByInput('exampleSeed1', method, inputStore, resultStore); | ||
// => 1 | ||
copycat.uniqueByInput('exampleSeed', method, inputStore, resultStore); | ||
// => 3 | ||
``` | ||
### `copycat.password(input)` | ||
@@ -375,0 +468,0 @@ |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
2875910
518
80378
2
679