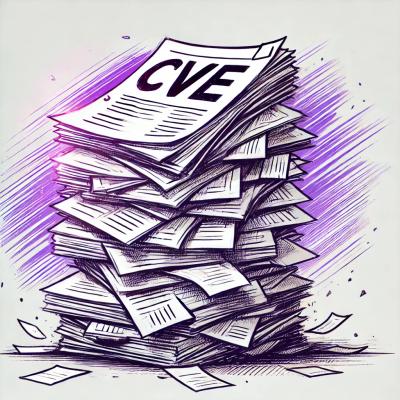
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@solid-primitives/i18n
Advanced tools
Library of primitives for providing internationalization support.
npm i @solid-primitives/i18n
# or
pnpm add @solid-primitives/i18n
# or
yarn add @solid-primitives/i18n
createI18nContext
Creates a method for internationalization support. This primitive set is largely inspired by dlv and passes all its tests.
import { I18nContext, createI18nContext, useI18n } from "@solid-primitives/i18n";
const App: Component = () => {
const [t, { add, locale, dict }] = useI18n();
const [name, setName] = createSignal("Greg");
const addLanguage = () => {
add("sw", { hello: "hej {{ name }}, hur mar du?" });
locale("sw");
};
return (
<>
<button onClick={() => locale("fr")}>fr</button>
<button onClick={() => locale("en")}>en</button>
<button onClick={() => locale("unknownLanguage")}>unknown language</button>
<button onClick={addLanguage}>add and set swedish</button>
<input value={name()} onInput={e => setName(e.target.value)} />
<hr />
<h1>{t("hello", { name: name() }, "Hello {{ name }}!")}!</h1>
<p>{locale()}</p>
<pre>
<code>{JSON.stringify(dict("sw"), null, 4)}</code>
</pre>
</>
);
};
const dict = {
fr: {
hello: "bonjour {{ name }}, comment vas-tu ?",
},
en: {
hello: "hello {{ name }}, how are you?",
},
};
const value = createI18nContext(dict, "fr");
<I18nContext.Provider value={value}>
<App />
</I18nContext.Provider>;
createChainedI18n
Creates a chained dictionary and manages the locale. Provides a proxy wrapper around translate so you can do chained calls that always returns with the current locale. IE t.hello()
import { createChainedI18n } from "@solid-primitives/i18n";
const dictionaries = {
fr: {
hello: "bonjour {{ name }}, comment vas-tu ?",
goodbye: ({ name }: { name: string }) => `au revoir ${name}`,
},
en: {
hello: "hello {{ name }}, how are you?",
goodbye: ({ name }: { name: string }) => `goodbye ${name}`,
},
};
const [t, { locale, setLocale, getDictionary }] = createChainedI18n({
dictionaries,
locale: "en", // Starting locale
});
createEffect(() => {
t.hello({ name: "Mathiew" });
});
createChainedI18nContext
Creates chained I18n state wrapped in a Context Provider to be shared with the app using the component tree.
import { createChainedI18nContext } from "@solid-primitives/i18n";
const { I18nProvider, useI18nContext } = makeChainedI18nContext({
dictionaries,
locale: "en", // Starting locale
});
export const useI18n = () => {
const context = useI18nContext();
if (!context) throw new Error("useI18n must be used within an I18nProvider");
return context;
};
const App: Component = () => {
const [t, { locale, setLocale, getDictionary }] = useI18n();
const [name, setName] = createSignal("Greg");
return (
<>
<button onClick={() => setLocale("fr")}>fr</button>
<button onClick={() => setLocale("en")}>en</button>
<button onClick={addLanguage}>add and set swedish</button>
<input value={name()} onInput={e => setName(e.target.value)} />
<hr />
<h1>{t.hello({ name: name() })}!</h1>
<p>{locale()}</p>
<p>{t.goodbye({ name: name() })}</p>
</>
);
};
<I18nContext.Provider value={value}>
<App />
</I18nContext.Provider>;
useScopedI18n
Use useScopedI18n
to create a module-specific translate.
const dict = {
en: {
login: {
username: 'User name',
password: 'Password',
login: 'Login'
},
fr: {
...
}
}
}
export const LoginView = () => {
const [t] = useScopedI18n('login');
return <>
<div>{t('username')}<input /></div>
<div>{t('password')}<input /></div>
<button>{t('login') }</button>
</>
}
You may view a working example of createI18nContext here: https://codesandbox.io/s/use-i18n-rd7jq?file=/src/index.tsx
See CHANGELOG.md
FAQs
Library of primitives for providing internationalization support.
The npm package @solid-primitives/i18n receives a total of 4,088 weekly downloads. As such, @solid-primitives/i18n popularity was classified as popular.
We found that @solid-primitives/i18n demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.