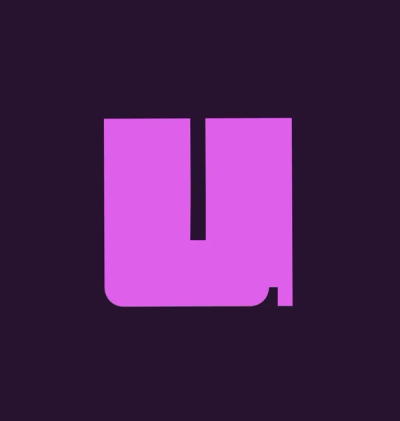
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
@solid-primitives/mouse
Advanced tools
A collection of Solid Primitives, that capture current mouse cursor position, and help to deal with common related usecases.
A collection of primitives, capturing current mouse cursor position, and helping to deal with common usecases:
createMousePosition
- Listens to the global mouse events, providing a reactive up-to-date position of the cursor on the page.createMouseToElement
- Provides an auto-updating position relative to a provided element. It can be used with existing position signals or left to get the current cursor position itself.createMouseInElement
- An alternative to createMouseToElement
, that listens to mouse (and touch) events only inside the element. Provides information of position and if the element is being hovered.createMouseOnScreen
- Answers the question: Is the cursor on screen?npm install @solid-primitives/mouse
# or
yarn add @solid-primitives/mouse
createMousePosition
Listens to the global mouse events, providing a reactive up-to-date position of the cursor on the page.
import { createMousePosition } from "@solid-primitives/mouse";
const [{ x, y, sourceType }, clear] = createMousePosition({ touch: false });
// listening to touch events is enabled by default
// to clear all event listeners
clear();
function createMousePosition(options: MouseOptions = {}): [
getters: {
x: Accessor<number>;
y: Accessor<number>;
sourceType: Accessor<MouseSourceType>;
},
clear: ClearListeners
];
interface MouseOptions {
/**
* Listen to `touchmove` events
* @default true
*/
touch?: boolean;
/**
* Initial values
* @default { x:0, y:0 }
*/
initialValue?: Position;
/**
* If enabled, position will be updated on touchmove event.
* @default true
*/
followTouch?: boolean;
}
interface Position {
x: number;
y: number;
}
type MouseSourceType = "mouse" | "touch" | null;
createMouseToElement
Provides an autoupdating position relative to a provided element. It can be used with existing position signals, or left to get the current cursor position itself.
import { createMouseToElement } from "@solid-primitives/mouse";
const [{ x, y, top, left, width, height, isInside }, manualUpdate] = createMouseToElement(
() => myRef
);
// If position argument is left undefined, it will use
// createMousePosition internally to track the cursor position.
// But if you are already tracking the mouse position yourself, or with createMousePosition.
// You can pass it to createMouseToElement to avoid additional performance payload.
const [mouse] = createMousePosition();
const [{ x, y, isInside }] = createMouseToElement(el, mouse);
// This also works when you are applying some transformations to the position, or debouncing it.
const myPos = createMemo(() => {
/* do sth with the mouse position */
});
const [{ x, y, isInside }] = createMouseToElement(el, myPos);
function createMouseToElement(
element: MaybeAccessor<Element>,
pos?: Accessor<Position> | { x: MaybeAccessor<number>; y: MaybeAccessor<number> },
options: PositionToElementOptions = {}
): [
getters: {
x: Accessor<number>;
y: Accessor<number>;
top: Accessor<number>;
left: Accessor<number>;
width: Accessor<number>;
height: Accessor<number>;
isInside: Accessor<boolean>;
},
update: Fn
];
interface Position {
x: number;
y: number;
}
interface PositionToElementOptions extends MouseOptions {
initialValue?: {
top?: number;
left?: number;
width?: number;
height?: number;
x?: number;
y?: number;
};
}
createMouseInElement
An alternative to createMouseToElement
, that listens to mouse (and touch) events only inside the element. Provides information of position and if is the element being currently hovered.
import { createMouseInElement } from "@solid-primitives/mouse";
const [{ x, y, sourceType, isInside }, clear] = createMouseInElement(() => myRef, {
followTouch: false
});
// Same way as createMousePosition:
// the "touch", and "foullowTouch" settings are enabled by default
// to clear all event listeners
clear();
function createMouseInElement(
element: MaybeAccessor<HTMLElement>,
options: MouseOptions = {}
): [
getters: {
x: Accessor<number>;
y: Accessor<number>;
sourceType: Accessor<MouseSourceType>;
isInside: Accessor<boolean>;
},
clear: ClearListeners
];
type MouseSourceType = "mouse" | "touch" | null;
createMouseOnScreen
Answers the question: Is the cursor on screen?
import { createMouseOnScreen } from "@solid-primitives/mouse";
const [isMouseOnScreen, clear] = createMouseOnScreen(true);
// to clear all event listeners
clear();
function createMouseOnScreen(
initialValue?: boolean
): [onScreen: Accessor<boolean>, clear: ClearListeners];
function createMouseOnScreen(
options?: MouseOnScreenOptions
): [onScreen: Accessor<boolean>, clear: ClearListeners];
interface MouseOnScreenOptions {
/**
* Listen to touch events
* @default true
*/
touch?: boolean;
/**
* Initial value
* @default false
*/
initialValue?: boolean;
}
https://codesandbox.io/s/solid-primitives-mouse-p10s5?file=/index.tsx
1.0.0
Release as a Stage-2 primitive.
1.0.1
Updated util and event-listener dependencies.
1.0.2
Upgraded to Solid 1.3
FAQs
A collection of Solid Primitives, that capture current mouse cursor position, and help to deal with common related usecases.
The npm package @solid-primitives/mouse receives a total of 2,393 weekly downloads. As such, @solid-primitives/mouse popularity was classified as popular.
We found that @solid-primitives/mouse demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.