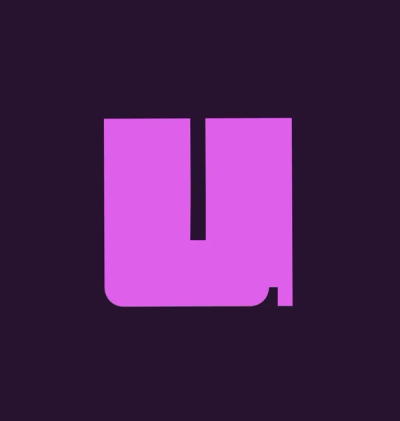
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
@solid-primitives/mouse
Advanced tools
A collection of Solid Primitives, that capture current mouse cursor position, and help to deal with common related usecases.
A collection of primitives, capturing current mouse cursor position, and helping to deal with common usecases:
createMousePosition
- Listens to the mouse events, providing a reactive up-to-date position of the cursor on the page.createPositionToElement
- Provides an auto-updating position relative to a provided element.makeMousePositionListener
- Attaches event listeners to provided target, listening for changes to the mouse/touch position.makeMouseInsideListener
- Attaches event listeners to provided target, listening for mouse/touch entering/leaving the element.getPositionToElement
- Turn position relative to the page, into position relative to an element.getPositionInElement
- Turn position relative to the page, into position relative to an element. Clamped to the element bounds.getPositionToScreen
- Turn position relative to the page, into position relative to the screen.npm install @solid-primitives/mouse
# or
pnpm add @solid-primitives/mouse
# or
yarn add @solid-primitives/mouse
createMousePosition
Attaches event listeners to provided target, providing a reactive up-to-date position of the cursor on the page.
import { createMousePosition } from "@solid-primitives/mouse";
const pos = createMousePosition(window);
createEffect(() => {
console.log(pos.x, pos.y);
});
// target can be a reactive signal
const [el, setEl] = createSignal(ref);
const pos = createMousePosition(el);
// if using a jsx ref, pass it as a function, or wrap primitive inside onMount
let ref;
const pos = createMousePosition(() => ref);
<div ref={ref}></div>;
By default createMousePosition
is listening to touch
events as well. You can disable that behavior with touch
and followTouch
options.
// disables following touch position – only registers touch start
const pos = createMousePosition(window, { followTouch: false });
// disables listening to any touch events
const pos = createMousePosition(window, { touch: false });
useMousePosition
This primitive provides a singleton root variant that will listen to window mouse position, and reuse event listeners and signals across dependents.
const pos = useMousePosition();
createEffect(() => {
console.log(pos.x, pos.y);
});
function createMousePosition(
target?: MaybeAccessor<SVGSVGElement | HTMLElement | Window | Document>,
options?: MousePositionOptions,
): MousePositionInside;
createPositionToElement
Provides an autoupdating position relative to an element based on provided page position.
import { createPositionToElement, useMousePosition } from "@solid-primitives/mouse";
const pos = useMousePosition();
const relative = createPositionToElement(ref, () => pos);
createEffect(() => {
console.log(relative.x, relative.y);
});
// target can be a reactive signal
const [el, setEl] = createSignal(ref);
const relative = createPositionToElement(el, () => pos);
// if using a jsx ref, pass it as a function, or wrap primitive inside onMount
let ref;
const relative = createPositionToElement(() => ref);
<div ref={ref}></div>;
function createPositionToElement(
element: Element | Accessor<Element | undefined>,
pos: Accessor<Position>,
options?: PositionToElementOptions,
): PositionRelativeToElement;
makeMousePositionListener
@2.0.0
Attaches event listeners to provided target, listening for changes to the mouse/touch position.
const clear = makeMousePositionListener(el, pos => console.log(pos), { touch: false });
// remove listeners manually (will happen on cleanup)
clear();
makeMouseInsideListener
@2.0.0
Attaches event listeners to provided target, listening for mouse/touch entering/leaving the element.
const clear = makeMouseInsideListener(el, inside => console.log(inside), { touch: false });
// remove listeners manually (will happen on cleanup)
clear();
getPositionToElement
Turn position relative to the page, into position relative to an element.
const pos = getPositionToElement(pageX, pageY, element);
getPositionInElement
Turn position relative to the page, into position relative to an element. Clamped to the element bounds.
const pos = getPositionInElement(pageX, pageY, element);
getPositionToScreen
Turn position relative to the page, into position relative to the screen.
const pos = getPositionToScreen(pageX, pageY);
https://codesandbox.io/s/solid-primitives-mouse-p10s5?file=/index.tsx
See CHANGELOG.md
FAQs
A collection of Solid Primitives, that capture current mouse cursor position, and help to deal with common related usecases.
The npm package @solid-primitives/mouse receives a total of 2,393 weekly downloads. As such, @solid-primitives/mouse popularity was classified as popular.
We found that @solid-primitives/mouse demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.