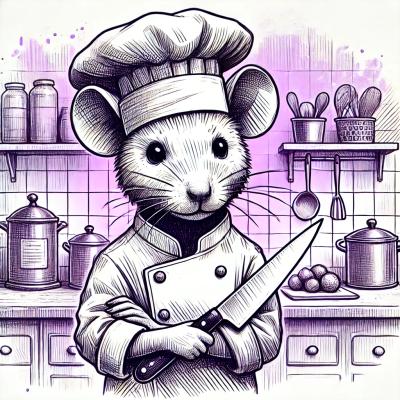
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@sonder/buzz.js
Advanced tools
Buzz.js is a simple tool to help you mock GraphQL responses in your components that use Apollo's useQuery
and useMutation
hooks.
With Buzz, there is no mocking overhead when mocking responses. Buzz will automatically generate a response with types that align to your schema, and make it easy for you to override specific peices when you need to. And everything is synchronous, so there's no need to add sleep
s to your code.
Install buzz
$ yarn add -D @sonder/buzz.js
Update your jest configuration to add a setup file to setupFilesAfterEnv
// package.json
...
"jest": {
"setupFilesAfterEnv": [
"jest/buzz.js" // 👈 add this
]
}
Load your local schema file onto the spaceship:
// jest/buzz.js
import moment from "moment";
import { loadSchemaFile, setMocks } from "@sonder/buzz.js";
beforeAll(() => {
loadSchemaFile("./schema.graphql"); // Relative path from the root of your workspace
// Optional: Mock out nonstandard scalar types in your schema
setMocks({
RFC3339DateTime: () => moment().format(),
});
});
Use it in your tests! Buzz is compatible with any DOM mocking library, including Enzyme and React Testing Library.
Let's say that we have a graphql schema that looks like this:
type HelloResponse {
id: ID!
hello: Boolean
message: String
}
type Mutation {
helloMutation: HelloResponse!
}
type Query {
hello: HelloResponse!
helloWithArgs(id: ID!): HelloResponse!
}
and a component that makes a request against this query:
const { useQuery } from '@apollo/client';
const SimpleQueryComponent = (): JSX.Element => {
const query = gql`
query TestQuery($id: ID!) {
helloWithArgs(id: $id) {
id
hello
message
}
}
`;
const { data, loading, error } = useQuery(query, {
variables: {
id: "test-input-id",
},
});
if (data) {
const { message, id } = data.helloWithArgs;
return (
<>
<div>ID: {id}</div>
{message && <div>Message: {message}</div>}
</>
);
}
if (loading) {
return <div>Loading...</div>;
} else if (error) {
return <div>Error: {error.message}</div>;
}
};
We can write our test like the following example:
import { mockUseQuery, restoreMocks } from "@sonder/buzz.js";
import { TestQuery, TestQueryVariables } from "../generated-types";
import { SimpleComponent } from "../app";
describe("SimpleComponent", () => {
/**
* 👈 important! Add this afterAll block to each describe block where mockQuery or mockMutation is used
*/
afterAll(() => {
restoreMocks();
});
it("mocks the response with no mocking overhead", () => {
// if you are using typescript, and have generated types, pass the query and variable types
mockUseQuery<TestQuery, TestQueryVariables>("TestQuery");
const { getByText } = render(<SimpleQueryComponent />);
expect(getByText(/^ID: example-id$/).textContent).not.toBeNull();
});
});
mockUseQuery
and mockUseMutation
both return validators which you can use to make assertions that your components execute the correct request
describe("SimpleComponent", () => {
it("mocks the response with no mocking overhead", () => {
const validator = mockUseQuery<TestQuery, TestQueryVariables>("TestQuery");
const { getByText } = render(<SimpleQueryComponent />);
expect(validator.getCalls().length).toEqual(1);
expect(validator.getCalls()[0].options.variables?.id).toEqual("test-id");
});
});
Need to customize the response? No problem:
describe("SimpleComponent", () => {
it("mocks the response with simple overrides", () => {
mockUseQuery<TesQuery, TestQueryVariables>("TestQuery", {
response: {
helloWithArgs: {
// Pass in a partial response to be merged with the mock
message: null,
},
},
});
const { getByText } = render(<SimpleQueryComponent />);
expect(getByText(/^Message: .*$/).textContent).not.toBeNull();
});
});
Need to mock loading or error states? Buzz can do that:
import { ApolloError } from "@apollo/client";
import { SimpleComponent } from "../app";
describe("SimpleComponent", () => {
it("displays a loading indicator", () => {
mockUseQuery<TestQuery, TestQueryVariables>("TestQuery", {
loading: true,
});
const { queryByText } = render(<SimpleQueryComponent />);
expect(queryByText(/^Loading\.\.\.$/).textContent).not.toBeNull();
expect(queryByText(/^ID: .*$/)).toBeNull();
});
it("displays an error message", () => {
mockUseQuery<TestQueryVariables>("TestQuery", {
error: new ApolloError({ errorMessage: "test-error" }),
});
const { getByTestId } = render(<SimpleQueryComponent />);
expect(queryByText(/^Error: test-error$/).textContent).not.toBeNull();
});
});
For more info, check out the API docs.
Buzz.js is only compatible with Apollo's hook functions useQuery
, and useMutation
. It is not compatible with Apollo HOCs or component integration types.
FAQs
Tools for mocking graphql queries and fragments
We found that @sonder/buzz.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.