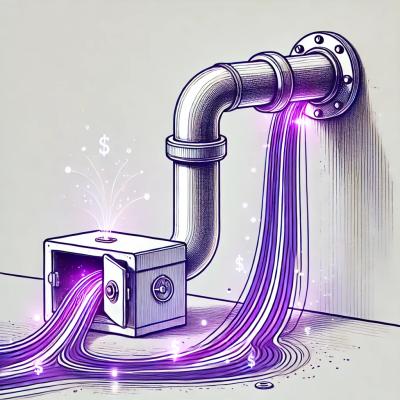
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@spectrum-web-components/menu
Advanced tools
An `<sp-menu>` is used for creating a menu list. The various elements inside a menu are given as `<sp-menu-group>`, `<sp-menu-item>`, or `<sp-menu-divider>`. Often a `<sp-menu>` element will appear in a `<sp-popover>` element so that it displays as a togg
An <sp-menu>
is used for creating a menu list. The various elements inside a menu are given as <sp-menu-group>
, <sp-menu-item>
, or <sp-menu-divider>
. Often a <sp-menu>
element will appear in a <sp-popover>
element so that it displays as a togglig menu.
yarn add @spectrum-web-components/menu
Import the side effectful registration of <sp-menu>
, <sp-menu-group>
, <sp-menu-item>
, or <sp-menu-divider>
individually as follows:
import '@spectrum-web-components/menu/sp-menu.js';
import '@spectrum-web-components/menu/sp-menu-group.js';
import '@spectrum-web-components/menu/sp-menu-item.js';
import '@spectrum-web-components/menu/sp-menu-divider.js';
When looking to leverage the Menu
, MenuGroup
, MenuItem
, or MenuDivider
base classes as a type and/or for extension purposes, do so via:
import {
Menu,
MenuGroup,
MenuItem,
MenuDivider
} from '@spectrum-web-components/menu';
<sp-menu>
<sp-menu-item>
Deselect
</sp-menu-item>
<sp-menu-item>
Select inverse
</sp-menu-item>
<sp-menu-item>
Feather...
</sp-menu-item>
<sp-menu-item>
Select and mask...
</sp-menu-item>
<sp-menu-item>
Save selection
</sp-menu-item>
<sp-menu-item disabled>
Make work path
</sp-menu-item>
</sp-menu>
Often an <sp-menu>
element will be delivered inside of an <sp-popover>
element when displaying it above other content.
<sp-popover open style="position: relative">
<sp-menu>
<sp-menu-item value="item-1">Deselect</sp-menu-item>
<sp-menu-item value="item-2">Select inverse</sp-menu-item>
<sp-menu-item value="item-3">Feather...</sp-menu-item>
<sp-menu-item value="item-4">Select and mask...</sp-menu-item>
<sp-menu-item value="item-5">Save selection</sp-menu-item>
<sp-menu-item value="item-6" disabled>Make work path</sp-menu-item>
</sp-menu>
</sp-popover>
The <sp-menu>
element can be instructed to maintain a selection via the selects
attribute. Depending on the chosen algorithm, the <sp-menu>
element will hold a value
property and manage the selected
state of its <sp-menu-item>
descendants.
When selects
is set to single
, the <sp-menu>
element will maintain one selected item after an initial selection is made.
<p>
The value of the `<sp-menu>` element is:
<span id="single-value"></span>
</p>
<sp-menu
label="Choose a shape"
selects="single"
onchange="this.previousElementSibling.querySelector('#single-value').textContent=this.value"
>
<sp-menu-item value="item-1">Square</sp-menu-item>
<sp-menu-item value="item-2">Triangle</sp-menu-item>
<sp-menu-item value="item-3">Parallelogram</sp-menu-item>
<sp-menu-item value="item-4">Star</sp-menu-item>
<sp-menu-item value="item-5">Hexagon</sp-menu-item>
<sp-menu-item value="item-6" disabled>Circle</sp-menu-item>
</sp-menu>
When selects
is set to multiple
, the <sp-menu>
element will maintain zero or more selected items.
<p>
The value of the `<sp-menu>` element is:
<span id="multiple-value">item-3,item-4</span>
</p>
<sp-menu
label="Choose some fruit"
selects="multiple"
onchange="this.previousElementSibling.querySelector('#multiple-value').textContent=this.value"
>
<sp-menu-item value="item-1">Apple</sp-menu-item>
<sp-menu-item value="item-2">Banana</sp-menu-item>
<sp-menu-item value="item-3" selected>Goji berry</sp-menu-item>
<sp-menu-item value="item-4" selected>Grapes</sp-menu-item>
<sp-menu-item value="item-5" disabled>Kumquat</sp-menu-item>
<sp-menu-item value="item-6">Orange</sp-menu-item>
</sp-menu>
When selects
is set to inherit
, the <sp-menu>
element will allow its <sp-menu-item>
children to participate in the selection of its nearest <sp-menu>
ancestor.
<p>
The value of the `<sp-menu>` element is:
<span id="inherit-value">item-3 || item-4 || item-8 || item-11</span>
</p>
<sp-menu
label="Choose some groceries"
selects="multiple"
value-separator=" || "
onchange="this.previousElementSibling.querySelector('#inherit-value').textContent=this.value"
>
<sp-menu label="Fruit" selects="inherit">
<sp-menu-item value="item-1">Apple</sp-menu-item>
<sp-menu-item value="item-2">Banana</sp-menu-item>
<sp-menu-item value="item-3" selected>Goji berry</sp-menu-item>
<sp-menu-item value="item-4" selected>Grapes</sp-menu-item>
<sp-menu-item value="item-5" disabled>Kumquat</sp-menu-item>
<sp-menu-item value="item-6">Orange</sp-menu-item>
</sp-menu>
<sp-menu label="Vegetables" selects="inherit">
<sp-menu-item value="item-7">Carrot</sp-menu-item>
<sp-menu-item value="item-8" selected>Garlic</sp-menu-item>
<sp-menu-item value="item-9" disabled>Lettuce</sp-menu-item>
<sp-menu-item value="item-10">Onion</sp-menu-item>
<sp-menu-item value="item-11" selected>Potato</sp-menu-item>
<sp-menu-item value="item-12">Tomato</sp-menu-item>
</sp-menu>
</sp-menu>
Whether <sp-menu>
carries a selection or not, when one of the <sp-menu-item>
children that it manages is activated the <sp-menu>
element will dispatch a change
event. At dispatch time, even when a selection is not held due to the absence of the selects
attribute, the value
of the <sp-menu>
will correspond to the <sp-menu-item>
that was activated. When the selects
attribute is present, this value
will be persisted beyond the lifecycle of the change
event. When selects="multiple"
the values of multiple items will be comma separated unless otherwise set via the value-separator
attribute.
Note: The change
event is only dispatched on a left mouse click or Enter/Space keypress. Right/Middle mouse clicks will not dispatch the change
event.
FAQs
An `<sp-menu>` is used for creating a menu list. The various elements inside a menu are given as `<sp-menu-group>`, `<sp-menu-item>`, or `<sp-menu-divider>`. Often a `<sp-menu>` element will appear in a `<sp-popover>` element so that it displays as a togg
We found that @spectrum-web-components/menu demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.