@splinetool/react-spline
Advanced tools
Comparing version 2.2.1 to 2.2.2
{ | ||
"name": "@splinetool/react-spline", | ||
"version": "2.2.1", | ||
"version": "2.2.2", | ||
"packageManager": "yarn@3.2.0", | ||
@@ -14,2 +14,3 @@ "files": [ | ||
".": { | ||
"types": "./dist/Spline.d.ts", | ||
"import": "./dist/react-spline.es.js", | ||
@@ -36,3 +37,3 @@ "require": "./dist/react-spline.cjs.js" | ||
"devDependencies": { | ||
"@splinetool/runtime": "^0.9.46", | ||
"@splinetool/runtime": "^0.9.65", | ||
"@types/animejs": "^3.1.4", | ||
@@ -44,2 +45,3 @@ "@types/node": "^17.0.31", | ||
"animejs": "^3.2.1", | ||
"modern-normalize": "^1.1.0", | ||
"np": "^7.6.1", | ||
@@ -46,0 +48,0 @@ "react": "^18.1.0", |
140
README.md
@@ -21,3 +21,2 @@ [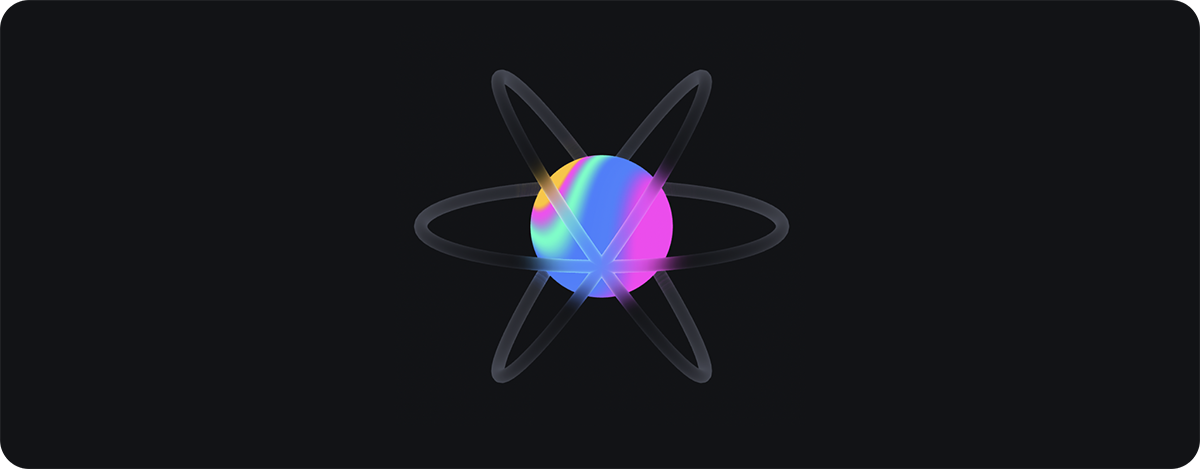](https://my.spline.design/splinereactlogocopycopy-eaa074bf6b2cc82d870c96e262a625ae/) | ||
- [Trigger Spline events from outside](#trigger-spline-events-from-outside) | ||
- [Usage with Next.js](#usage-with-nextjs) | ||
- [Lazy loading](#lazy-loading) | ||
@@ -58,3 +57,3 @@ - [API](#api) | ||
<div> | ||
<Spline scene="https://prod.spline.design/TRfTj83xgjIdHPmT/scene.spline" /> | ||
<Spline scene="https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode" /> | ||
</div> | ||
@@ -71,3 +70,3 @@ ); | ||
You can query any Spline object via `findObjectById` or `findObjectByName`. | ||
You can query any Spline object via `findObjectByName` or `findObjectById`. | ||
@@ -77,20 +76,22 @@ _(You can get the ID of the object in the `Develop` pane of the right sidebar)._ | ||
```jsx | ||
import { useRef } from 'react'; | ||
import Spline from '@splinetool/react-spline'; | ||
export default function App() { | ||
const [myObj, setMyObj] = useState(null); | ||
const cube = useRef(); | ||
function onLoad(spline) { | ||
const obj = spline.findObjectById('8E8C2DDD-18B6-4C54-861D-7ED2519DE20E'); | ||
const obj = spline.findObjectByName('Cube'); | ||
// or | ||
// const obj = spline.findObjectByName('my object'); | ||
// const obj = spline.findObjectById('8E8C2DDD-18B6-4C54-861D-7ED2519DE20E'); | ||
setMyObj(obj); | ||
// save it in a ref for later use | ||
cube.current = obj; | ||
} | ||
function moveObj() { | ||
console.log(myObj); // Spline Object => { name: 'my object', id: '8E8C2DDD-18B6-4C54-861D-7ED2519DE20E', position: {}, ... } | ||
console.log(cube.current); // Spline Object => { name: 'Cube', id: '8E8C2DDD-18B6-4C54-861D-7ED2519DE20E', position: {}, ... } | ||
// move the object in 3D space | ||
myObj.position.x += 10; | ||
cube.current.position.x += 10; | ||
} | ||
@@ -101,7 +102,7 @@ | ||
<Spline | ||
scene="https://prod.spline.design/TRfTj83xgjIdHPmT/scene.spline" | ||
scene="https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode" | ||
onLoad={onLoad} | ||
/> | ||
<button type="button" onClick={moveObj}> | ||
Move {myObj.name} | ||
Move Cube | ||
</button> | ||
@@ -122,4 +123,4 @@ </div> | ||
function onMouseDown(e) { | ||
if (e.target.id === '8E8C2DDD-18B6-4C54-861D-7ED2519DE20E') { | ||
// doSomething(); | ||
if (e.target.name === 'Cube') { | ||
console.log('I have been clicked!'); | ||
} | ||
@@ -131,3 +132,3 @@ } | ||
<Spline | ||
scene="https://prod.spline.design/TRfTj83xgjIdHPmT/scene.spline" | ||
scene="https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode" | ||
onMouseDown={onMouseDown} | ||
@@ -151,13 +152,15 @@ /> | ||
```jsx | ||
import { useRef } from 'react'; | ||
import Spline from '@splinetool/react-spline'; | ||
export default function App() { | ||
const [spline, setSpline] = useState(); | ||
const spline = useRef(); | ||
function onLoad(spline) { | ||
setSpline(spline); | ||
function onLoad(splineApp) { | ||
// save the app in a ref for later use | ||
spline.current = splineApp; | ||
} | ||
function triggerAnimation() { | ||
spline.emitEvent('mouseHover', '8E8C2DDD-18B6-4C54-861D-7ED2519DE20E'); | ||
spline.current.emitEvent('mouseHover', 'Cube'); | ||
} | ||
@@ -168,3 +171,3 @@ | ||
<Spline | ||
scene="https://prod.spline.design/TRfTj83xgjIdHPmT/scene.spline" | ||
scene="https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode" | ||
onLoad={onLoad} | ||
@@ -183,14 +186,16 @@ /> | ||
```jsx | ||
import { useRef } from 'react'; | ||
import Spline from '@splinetool/react-spline'; | ||
export default function App() { | ||
const [objectToAnimate, setObjectToAnimate] = useState(null); | ||
const objectToAnimate = useRef(); | ||
function onLoad(spline) { | ||
const obj = spline.findObjectById('8E8C2DDD-18B6-4C54-861D-7ED2519DE20E'); | ||
setObjectToAnimate(obj); | ||
const obj = spline.findObjectByName('Cube'); | ||
// save the object in a ref for later use | ||
objectToAnimate.current = obj; | ||
} | ||
function triggerAnimation() { | ||
objectToAnimate.emitEvent('mouseHover'); | ||
objectToAnimate.current.emitEvent('mouseHover'); | ||
} | ||
@@ -201,3 +206,3 @@ | ||
<Spline | ||
scene="https://prod.spline.design/TRfTj83xgjIdHPmT/scene.spline" | ||
scene="https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode" | ||
onLoad={onLoad} | ||
@@ -215,69 +220,2 @@ /> | ||
### Usage with Next.js | ||
Because react-spline only works on client-side, it needs to be registered as a client-side only component or be [lazy loaded](#lazy-loading). | ||
You can use [next/dynamic](https://nextjs.org/docs/advanced-features/dynamic-import) to import it as client-side only component: | ||
```jsx | ||
import dynamic from 'next/dynamic'; | ||
const Spline = dynamic(() => import('@splinetool/react-spline'), { | ||
ssr: false, | ||
}); | ||
export default function App() { | ||
return ( | ||
<div> | ||
<Spline scene="https://prod.spline.design/TRfTj83xgjIdHPmT/scene.spline" /> | ||
</div> | ||
); | ||
} | ||
``` | ||
However, if you need to use the `ref` prop, you will need to create a wrapped component and import it dynamically: | ||
1. Create a wrapped component. | ||
```jsx | ||
import Spline from '@splinetool/react-spline'; | ||
export function WrappedSpline({ splineRef, ...props }) { | ||
return <Spline ref={splineRef} {...props} />; | ||
} | ||
``` | ||
2. Use [next/dynamic](https://nextjs.org/docs/advanced-features/dynamic-import) to import client-side component. | ||
```jsx | ||
import dynamic from 'next/dynamic'; | ||
const WrappedSpline = dynamic(() => import('./WrappedSpline'), { | ||
ssr: false, | ||
}); | ||
const Spline = forwardRef((props, ref) => { | ||
return <WrappedSpline {...props} splineRef={ref} />; | ||
}); | ||
``` | ||
```jsx | ||
export default function App() { | ||
const ref = useRef(); | ||
useEffect(() => { | ||
// you can access splineRef.current here | ||
}, []); | ||
return ( | ||
<div> | ||
<Spline | ||
scene="https://prod.spline.design/TRfTj83xgjIdHPmT/scene.spline" | ||
ref={ref} | ||
/> | ||
</div> | ||
); | ||
} | ||
``` | ||
### Lazy loading | ||
@@ -296,3 +234,3 @@ | ||
<Suspense fallback={<div>Loading...</div>}> | ||
<Spline scene="https://prod.spline.design/TRfTj83xgjIdHPmT/scene.spline" /> | ||
<Spline scene="https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode" /> | ||
</Suspense> | ||
@@ -316,5 +254,5 @@ </div> | ||
| `className?` | `string` | CSS classes | | ||
| `style?` | `string` | CSS style | | ||
| `style?` | `object` | CSS style | | ||
| `id?` | `string` | Canvas id | | ||
| `ref?` | `React.Ref<HTMLDivElement>` | A ref pointing to the container `div`. | | ||
| `ref?` | `React.Ref<HTMLDivElement>` | A ref pointing to canvas element. | | ||
| `onLoad?` | `(spline: Application) => void` | Gets called once the scene has loaded. The `spline` parameter is an instance of the [Spline Application](#spline-app-methods) | | ||
@@ -335,9 +273,9 @@ | `onWheel?` | `(e: SplineEvent) => void` | Gets called on the [`wheel`](https://developer.mozilla.org/en-US/docs/Web/API/Element/wheel_event) event on the canvas | | ||
| Name | Type | Description | | ||
| ------------------ | ---------------------------------------------------- | --------------------------------------------------------------------------------------------------------------------------- | | ||
| `emitEvent` | `(eventName: SplineEventName, uuid: string) => void` | Triggers a Spline event associated to an object with provided uuid in reverse order. Starts from first state to last state. | | ||
| `emitEventReverse` | `(eventName: SplineEventName, uuid: string) => void` | Triggers a Spline event associated to an object with provided uuid in reverse order. Starts from last state to first state. | | ||
| `findObjectById` | `(uuid: string) => SPEObject` | Searches through scene's children and returns the object with that uuid. | | ||
| `findObjectByName` | `(name: string) => SPEObject` | Searches through scene's children and returns the first object with that name | | ||
| `setZoom` | `(zoom: number) => void` | Sets the initial zoom of the scene. | | ||
| Name | Type | Description | | ||
| ------------------ | ---------------------------------------------------------- | --------------------------------------------------------------------------------------------------------------------------- | | ||
| `emitEvent` | `(eventName: SplineEventName, nameOrUuid: string) => void` | Triggers a Spline event associated to an object with provided name or uuid. | | ||
| `emitEventReverse` | `(eventName: SplineEventName, nameOrUuid: string) => void` | Triggers a Spline event associated to an object with provided uuid in reverse order. Starts from last state to first state. | | ||
| `findObjectById` | `(uuid: string) => SPEObject` | Searches through scene's children and returns the object with that uuid. | | ||
| `findObjectByName` | `(name: string) => SPEObject` | Searches through scene's children and returns the first object with that name. | | ||
| `setZoom` | `(zoom: number) => void` | Sets the initial zoom of the scene. | | ||
@@ -344,0 +282,0 @@ ### Spline Events |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Network access
Supply chain riskThis module accesses the network.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No License Found
License(Experimental) License information could not be found.
Found 1 instance in 1 package
3069127
14
0
19000
13
309
11