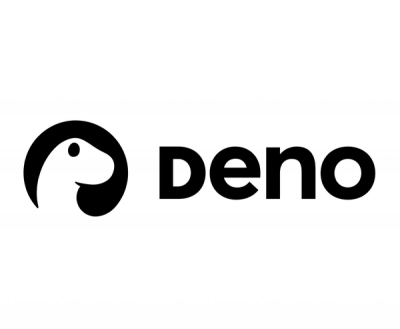
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@splinetool/runtime
Advanced tools
Spline is a collaborative design platform for creating production-ready interactive experiences in multiple dimensions. © 2024 Spline, Inc.
@splinetool/runtime is an npm package that allows developers to integrate and manipulate 3D scenes created with Spline, a 3D design tool, directly within their web applications. It provides a runtime environment to load, render, and interact with 3D models and scenes.
Loading a Spline Scene
This feature allows you to load a Spline scene into your web application. You can specify the path to your Spline file, and the scene will be loaded and ready for rendering.
const Spline = require('@splinetool/runtime');
const scene = new Spline.Scene();
scene.load('path/to/your/spline-file.splinecode');
Rendering the Scene
This feature enables you to render the loaded Spline scene onto a specified HTML canvas element. The renderer takes care of displaying the 3D content in the browser.
const canvas = document.getElementById('myCanvas');
const renderer = new Spline.Renderer({ canvas });
renderer.render(scene);
Interacting with the Scene
This feature allows you to add interactivity to your Spline scene. You can listen for events such as clicks and respond accordingly, making your 3D content interactive.
scene.on('click', (event) => {
console.log('Object clicked:', event.object);
});
Three.js is a popular JavaScript library for creating and displaying 3D graphics in the browser. It provides a wide range of features for rendering 3D scenes, including support for WebGL, shaders, and various geometries. Compared to @splinetool/runtime, Three.js offers more low-level control and flexibility but requires more effort to set up and manage 3D scenes.
Babylon.js is a powerful, open-source 3D engine that allows developers to create stunning 3D experiences in the browser. It offers a comprehensive set of features, including a physics engine, particle systems, and support for various 3D formats. Babylon.js is similar to Three.js in terms of capabilities but provides a more extensive set of tools and utilities for building complex 3D applications.
A-Frame is a web framework for building virtual reality (VR) experiences. It is built on top of Three.js and provides an easy-to-use, declarative syntax for creating 3D and VR content. A-Frame is particularly well-suited for VR applications and offers a higher-level abstraction compared to Three.js and Babylon.js, making it easier to get started with 3D development.
runtime allows you to run Spline scenes in javascript.
yarn add @splinetool/runtime
or
npm install @splinetool/runtime
To use runtime, first you have to go to the Spline editor, click on the Export button, select "Code" and then "Vanilla JS".
You can copy the URL there and pass it to the .load()
function:
import { Application } from '@splinetool/runtime';
// make sure you have a canvas in the body
const canvas = document.getElementById('canvas3d');
// start the application and load the scene
const spline = new Application(canvas);
spline.load('https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode');
You should be able to see the scene you exported in your canvas.
NOTE: If you are experiencing CORS issues, you can download the .splinecode file and self-host it; this will fix any CORS issue. To download, go to Spline's code export panel and click on the download icon visible in the prod.spline textarea.
:warning: Only .splinecode files should be loaded through this API.
.spline
files are meant to be used in the editor.
You can query any Spline object via findObjectByName
or findObjectById
.
(You can get the ID of the object in the Develop
pane of the right sidebar).
import { Application } from '@splinetool/runtime';
const canvas = document.getElementById('canvas3d');
const spline = new Application(canvas);
spline
.load('https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode')
.then(() => {
const obj = spline.findObjectByName('Cube');
// or
// const obj = spline.findObjectById('7AF5EBC0-09BB-4720-B045-F478F8053AA4');
console.log(obj); // Spline Object => { name: 'Cube', id: '7AF5EBC0-09BB-4720-B045-F478F8053AA4', position: {}, ... }
// move the object in 3D space
obj.position.x += 10;
});
You can listen to any Spline Event you set in the Events panel of the editor by attaching a listener to the Spline instance.
import { Application } from '@splinetool/runtime';
const canvas = document.getElementById('canvas3d');
const spline = new Application(canvas);
spline
.load('https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode')
.then(() => {
spline.addEventListener('mouseDown', (e) => {
if (e.target.name === 'Cube') {
console.log('I have been clicked!');
}
});
});
You can find a list of all of the Spline Event listeners in the API section.
You can trigger any animation Event you set in the Events panel in the Spline Editor.
You can use the emitEvent
function, passing the event type and the name or ID of your object.
(You can get the ID of the object in the Develop
pane of the right sidebar).
import { Application } from '@splinetool/runtime';
const canvas = document.getElementById('canvas3d');
const spline = new Application(canvas);
spline
.load('https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode')
.then(() => {
spline.emitEvent('mouseHover', 'Cube');
});
Or you can query the spline object first, and then trigger the event:
import { Application } from '@splinetool/runtime';
const canvas = document.getElementById('canvas3d');
const spline = new Application(canvas);
spline
.load('https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode')
.then(() => {
const obj = spline.findObjectByName('Cube');
objectToAnimate.emitEvent('mouseHover');
});
You can find a list of all of the Spline Events you can pass to the emitEvent
function in the Spline Events section.
You might want to start the loading of .splinecode
file before your code is loaded. It's possible using a HTML preload Link tag. Doing so will only save a little time by ensuring the spline file loading starts before your scripts are done loading. Since internally the .splinecode
file will be loaded through a fetch
call, you can do it like this :
<html>
<head>
<!--
add a preload link tag
with the scene your want to preload
at the end of your <head>
It needs to use the fetch preload type
-->
<link rel="preload" href="https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode" as="fetch"
</head>
/*
When loading the Application, use the third
param of the load function to make sure the browser
will use the preloaded file and not make another request
*/
spline.load(
'https://prod.spline.design/6Wq1Q7YGyM-iab9i/scene.splinecode',
undefined,
{
credentials: 'include',
mode: 'no-cors',
}
);
If you setup variables in your Spline scene from the editor, you can change them from code either while loading the scene, of after it's loaded. Note that if in Spline editor you have multiple variables with the same name, only the first one will be updated, so make sure to give unique names to the variables you want to update. Also note that the values you pass to your variables will be casted into their original type (number, boolean or string).
const spline = new Application(canvas);
// Create an object describing the variables you want to update during load
const myVariables = { myName: 'John', mySize: 350 };
// And pass them as second parameter for the load function
spline.load('.../scene.splinecode', myVariables);
// Later you can update your variables again
spline.setVariables({ myName: 'Paul', mySize: 100 });
// Or change only one variable
spline.setVariable('myName', 'Ringo');
You can call all these different methods on the Spline Application
instance.
Name | Type | Description |
---|---|---|
addEventListener | (eventName: SplineEventName, cb: (e: SplineEvent) => void) => void | Add an event listener for Spline events |
removeEventListener | (eventName: SplineEventName, cb: (e: SplineEvent) => void) => void | Remove an event listener for Spline events |
emitEvent | (eventName: SplineEventName, nameOrUuid: string) => void | Triggers a Spline event associated to an object with provided name or uuid in reverse order. Starts from first state to last state. |
emitEventReverse | (eventName: SplineEventName, nameOrUuid: string) => void | Triggers a Spline event associated to an object with provided name or uuid in reverse order. Starts from last state to first state. |
findObjectById | (uuid: string) => SPEObject | Searches through scene's children and returns the object with that uuid. |
findObjectByName | (name: string) => SPEObject | Searches through scene's children and returns the first object with that name. |
setZoom | (zoom: number) => void | Sets the camera zoom, expects a number value > 0 where 1 is base zoom. |
setSize | (width: number, height: number) => void | Sets the size of the application and canvas. When called, Spline will stop automatic size updates. |
setVariables | (variables: Record<string, string | number | boolean>) => void | Updates values for passed variables by name. |
setVariable | (name: string, value: string | number | boolean) => void | Updates value for passed variable by name. |
getVariables | () => Record<string, string | number | boolean> | Returns a record mapping variable names to their respective current values. |
getVariable | (name: string, value: string | number | boolean) => void | Get current value for a specific variable from its name |
stop | () => void | Stop/Pause all rendering controls and events. |
play | () => void | Play/Resume rendering, controls and events. |
setBackgroundColor | (color:string) => void | Manually sets the scene/canvas background color with a css color value. |
getAllObjects | () => SPEObject[] | Returns a flat list of all the objects present in the scene. |
getSplineEvents | () => Object[] | Returns an array listing all the Spline events used in the scene. |
These are all the Spline event types that you can pass to the addEventListener
, emitEvent
and emitEventReverse
function.
Name | Description |
---|---|
mouseDown | Refers to the Spline Mouse Down event type |
mouseHover | Refers to the Spline Mouse Hover event type |
mouseUp | Refers to the Spline Mouse Up event type |
keyDown | Refers to the Spline Key Down event type |
keyUp | Refers to the Spline Key Up event type |
start | Refers to the Spline Start event type |
lookAt | Refers to the Spline Look At event type |
follow | Refers to the Spline Mouse Up event type |
scroll | Refers to the Spline Scroll event type |
© 2024 Spline, Inc.
FAQs
Spline is a collaborative design platform for creating production-ready interactive experiences in multiple dimensions. © 2024 Spline, Inc.
The npm package @splinetool/runtime receives a total of 139,457 weekly downloads. As such, @splinetool/runtime popularity was classified as popular.
We found that @splinetool/runtime demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.