What is @stencil/core?
@stencil/core is a compiler for building fast web apps using Web Components. It combines the best features of popular frameworks into a simple build-time tool. Stencil generates standards-compliant Web Components that work in any major framework or with no framework at all.
What are @stencil/core's main functionalities?
Component Creation
Stencil allows you to create reusable web components. The example demonstrates a simple component that takes a 'name' property and renders a greeting message.
```typescript
import { Component, Prop, h } from '@stencil/core';
@Component({
tag: 'my-component',
styleUrl: 'my-component.css',
shadow: true
})
export class MyComponent {
@Prop() name: string;
render() {
return <div>Hello, {this.name}!</div>;
}
}
```
Reactive Data Binding
Stencil provides reactive data binding using the @State decorator. The example shows a counter component that updates its state and re-renders when the button is clicked.
```typescript
import { Component, State, h } from '@stencil/core';
@Component({
tag: 'counter-component',
styleUrl: 'counter-component.css',
shadow: true
})
export class CounterComponent {
@State() count: number = 0;
increment() {
this.count += 1;
}
render() {
return (
<div>
<p>Count: {this.count}</p>
<button onClick={() => this.increment()}>Increment</button>
</div>
);
}
}
```
Lazy Loading
Stencil supports lazy loading of components to improve performance. The example shows a component that will be lazy-loaded when needed.
```typescript
import { Component, h } from '@stencil/core';
@Component({
tag: 'lazy-component',
styleUrl: 'lazy-component.css',
shadow: true,
assetsDirs: ['assets']
})
export class LazyComponent {
render() {
return <div>Lazy Loaded Component</div>;
}
}
```
Other packages similar to @stencil/core
lit
Lit is a simple library for building fast, lightweight web components. It offers a similar approach to Stencil but focuses more on simplicity and performance. Unlike Stencil, Lit does not include a compiler and relies on JavaScript for defining components.
svelte
Svelte is a radical new approach to building user interfaces. It shifts much of the work to compile time, resulting in highly optimized JavaScript. Svelte components are compiled to highly efficient imperative code that directly manipulates the DOM, similar to Stencil's approach.
vue
Vue.js is a progressive JavaScript framework for building user interfaces. While not specifically focused on web components, Vue can be used to create reusable components and offers a rich ecosystem. Vue's approach is more framework-oriented compared to Stencil's compiler-based approach.
Stencil
A compiler for generating Web Components using technologies like TypeScript and JSX, built by the Ionic team.
Getting Started
Start a new project by following our quick Getting Started guide.
We would love to hear from you!
If you have any feedback or run into issues using Stencil, please file an issue on this repository.
Examples
A Stencil component looks a lot like a class-based React component, with the addition of TypeScript decorators:
import { Component, Prop, h } from '@stencil/core';
@Component({
tag: 'my-component',
styleUrl: 'my-component.css',
shadow: true,
})
export class MyComponent {
@Prop() first: string;
@Prop() last: string;
render() {
return (
<div>
Hello, my name is {this.first} {this.last}
</div>
);
}
}
The component above can be used like any other HTML element:
<my-component first="Stencil" last="JS"></my-component>
Since Stencil generates web components, they work in any major framework or with no framework at all.
In many cases, Stencil can be used as a drop in replacement for traditional frontend framework, though using it as such is certainly not required.
Contributing
Thanks for your interest in contributing!
Please take a moment to read up on our guidelines for contributing.
Please note that this project is released with a Contributor Code of Conduct. By participating in this project you agree to abide by its terms.
Thanks
Stencil's internal testing suite is supported by the BrowserStack Open-Source Program
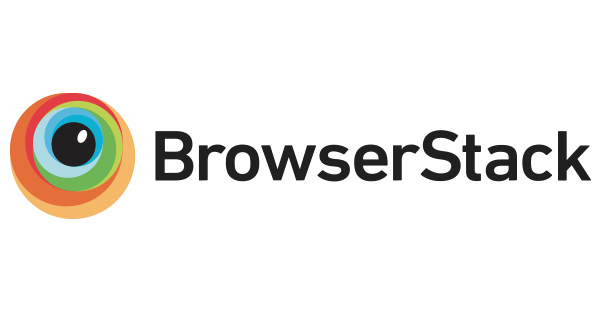