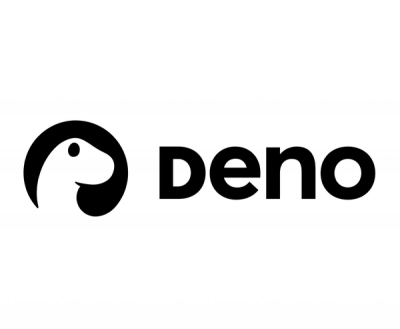
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@storybook/channel-websocket
Advanced tools
The @storybook/channel-websocket npm package is used within the Storybook ecosystem to facilitate communication between the Storybook UI and the server or between different frames of the UI using WebSockets. This package is particularly useful for enabling real-time updates and interactions in the Storybook UI, which is a tool for developing UI components in isolation for React, Vue, Angular, and more.
Establishing WebSocket connection
This code sample demonstrates how to establish a WebSocket connection using the WebSocketTransport class provided by @storybook/channel-websocket. This is essential for enabling real-time communication between the Storybook UI and the server.
import { WebSocketTransport } from '@storybook/channel-websocket';
const transport = new WebSocketTransport({ url: 'ws://localhost:9001' });
const channel = createChannel({ transport });
Sending messages
This code sample shows how to send messages over the established WebSocket connection. This is useful for triggering events or sending data from one part of the UI to another or to the server.
channel.emit('event-name', { payload: 'data' });
Receiving messages
This code sample illustrates how to listen for messages on the WebSocket channel. This allows the UI to react to data or events sent from the server or other parts of the UI.
channel.on('event-name', (data) => {
console.log('Received data:', data);
});
Socket.IO-client is a popular WebSocket library that enables real-time, bidirectional and event-based communication. It is similar to @storybook/channel-websocket but is more general-purpose and not specifically tailored for Storybook.
The 'ws' package is a simple to use, blazing fast, and thoroughly tested WebSocket client and server implementation. Unlike @storybook/channel-websocket, 'ws' provides both client and server-side capabilities, making it more versatile for different WebSocket needs.
Storybook Websocket Channel is a channel for Storybook that can be used when the Storybook Renderer should communicate with the Storybook Manager over the network.
A channel can be created using the createChannel
function.
import createChannel from '@storybook/channel-websocket';
const channel = createChannel({ url: 'ws://localhost:9001' });
For more information visit: storybook.js.org
7.0.24
file-system-cache
to 2.3.0 - #23221, thanks @JReinhold!FAQs
Unknown package
The npm package @storybook/channel-websocket receives a total of 768,937 weekly downloads. As such, @storybook/channel-websocket popularity was classified as popular.
We found that @storybook/channel-websocket demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.