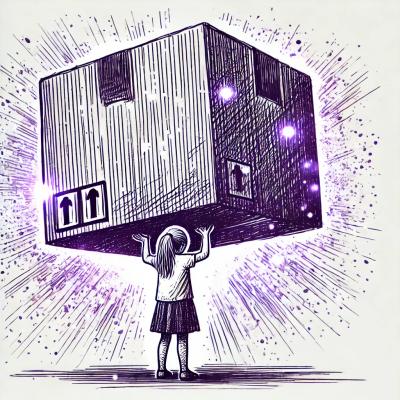
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
@swagger-api/apidom-ns-json-schema-draft-7
Advanced tools
JSON Schema Draft 7 namespace for ApiDOM.
@swagger-api/apidom-ns-json-schema-draft-7 is an npm package that provides tools for working with JSON Schema Draft-7. It is part of the ApiDOM ecosystem, which aims to provide a unified way to work with different API description formats.
Schema Validation
This feature allows you to validate JSON data against a JSON Schema Draft-7 schema. The code sample demonstrates how to validate an object with a required 'name' property.
const { validate } = require('@swagger-api/apidom-ns-json-schema-draft-7');
const schema = { type: 'object', properties: { name: { type: 'string' } }, required: ['name'] };
const data = { name: 'John Doe' };
const result = validate(schema, data);
console.log(result);
Schema Compilation
This feature allows you to compile a JSON Schema Draft-7 schema into a validation function. The code sample demonstrates how to compile a schema and use the resulting function to validate data.
const { compile } = require('@swagger-api/apidom-ns-json-schema-draft-7');
const schema = { type: 'object', properties: { age: { type: 'integer' } }, required: ['age'] };
const validate = compile(schema);
const data = { age: 30 };
const result = validate(data);
console.log(result);
Schema Resolution
This feature allows you to resolve JSON Schema Draft-7 references. The code sample demonstrates how to resolve a schema reference to its actual schema.
const { resolve } = require('@swagger-api/apidom-ns-json-schema-draft-7');
const schema = { $ref: 'http://example.com/schema.json' };
resolve(schema).then(resolvedSchema => console.log(resolvedSchema));
Ajv is a popular JSON Schema validator that supports JSON Schema Draft-7 and other versions. It is known for its high performance and extensive features, including custom keywords and formats. Compared to @swagger-api/apidom-ns-json-schema-draft-7, Ajv offers more flexibility and is widely used in the community.
The jsonschema package is a simple and easy-to-use JSON Schema validator for JavaScript. It supports JSON Schema Draft-7 and is suitable for basic validation needs. Compared to @swagger-api/apidom-ns-json-schema-draft-7, jsonschema is more lightweight but may lack some advanced features.
Jsen is a fast JSON Schema validator that supports JSON Schema Draft-4 and Draft-6. It focuses on performance and simplicity. While it does not support Draft-7, it is still a viable option for projects using older schema versions. Compared to @swagger-api/apidom-ns-json-schema-draft-7, Jsen is faster but less feature-rich.
@swagger-api/apidom-ns-json-schema-draft-7
contains ApiDOM namespace specific to JSON Schema Draft 7 specification.
You can install this package via npm CLI by running the following command:
$ npm install @swagger-api/apidom-ns-json-schema-draft-7
JSON Schema Draft 7 namespace consists of number of elements implemented on top of primitive ones.
import { createNamespace } from '@swagger-api/apidom-core';
import jsonShemaDraft7Namespace from '@swagger-api/apidom-ns-json-schema-draft-7';
const namespace = createNamespace(jsonShemaDraft7Namespace);
const objectElement = new namespace.elements.Object();
const jsonSchemaElement = new namespace.elements.JSONSchemaDraft7();
When namespace instance is created in this way, it will extend the base namespace with the namespace provided as an argument.
Elements from the namespace can also be used directly by importing them.
import { JSONSchemaElement, JSONReferenceElement, LinkDescriptionElement } from '@swagger-api/apidom-ns-json-schema-draft-7';
const jsonSchemaElement = new JSONSchemaElement();
const jsonReferenceElement = new JSONReferenceElement();
const linkDescriptionElement = new LinkDescriptionElement();
This package exposes predicates for all higher order elements that are part of this namespace.
import { isJSONSchemaElement, JSONSchemaElement } from '@swagger-api/apidom-ns-json-schema-draft-7';
const jsonSchemaElement = new JSONSchemaElement();
isJSONSchemaElement(jsonSchemaElement); // => true
Traversing ApiDOM in this namespace is possible by using visit
function from apidom
package.
This package comes with its own keyMap and nodeTypeGetter.
To learn more about these visit
configuration options please refer to @swagger-api/apidom-ast documentation.
import { visit } from '@swagger-api/apidom-core';
import { JSONSchemaElement, keyMap, getNodeType } from '@swagger-api/apidom-ns-json-schema-draft-7';
const element = new JSONSchemaElement();
const visitor = {
JSONSchemaDraft7Element(jsonSchemaElement) {
console.dir(jsonSchemaElement);
},
};
visit(element, visitor, { keyMap, nodeTypeGetter: getNodeType });
Refractor is a special layer inside the namespace that can transform either JavaScript structures or generic ApiDOM structures into structures built from elements of this namespace.
Refracting JavaScript structures:
import { LinkDescriptionElement } from '@swagger-api/apidom-ns-json-schema-draft-7';
const object = {
anchor: 'nodes/{thisNodeId}',
anchorPointer: '#/relative/json/pointer',
};
LinkDescriptionElement.refract(object); // => LinkDescriptionElement({ anchor, anchorPointer })
Refracting generic ApiDOM structures:
import { ObjectElement } from '@swagger-api/apidom-core';
import { LinkDescriptionElement } from '@swagger-api/apidom-ns-json-schema-draft-7';
const objectElement = new ObjectElement({
anchor: 'nodes/{thisNodeId}',
anchorPointer: '#/relative/json/pointer',
});
LinkDescriptionElement.refract(objectElement); // => LinkDescriptionElement({ anchor = 'nodes/{thisNodeId}', anchorPointer = '#/relative/json/pointer' })
Refractors can accept plugins as a second argument of refract static method.
import { ObjectElement } from '@swagger-api/apidom-core';
import { LinkDescriptionElement } from '@swagger-api/apidom-ns-json-schema-draft-7';
const objectElement = new ObjectElement({
anchor: 'nodes/{thisNodeId}',
anchorPointer: '#/relative/json/pointer',
});
const plugin = ({ predicates, namespace }) => ({
name: 'plugin',
pre() {
console.dir('runs before traversal');
},
visitor: {
LinkDescriptionElement(linkDescriptionElement) {
linkDescriptionElement.anchorPointer = '#/relative/json/pointer/x';
},
},
post() {
console.dir('runs after traversal');
},
});
MediaElement.refract(objectElement, { plugins: [plugin] }); // => LinkDescriptionElement({ anchor = 'nodes/{thisNodeId}', anchorPointer = '#/relative/json/pointer/x' })
You can define as many plugins as needed to enhance the resulting namespaced ApiDOM structure. If multiple plugins with the same visitor method are defined, they run in parallel (just like in Babel).
This plugin is specific to YAML 1.2 format, which allows defining key-value pairs with empty key, empty value, or both. If the value is not provided in YAML format, this plugin compensates for this missing value with the most appropriate semantic element type.
import { parse } from '@swagger-api/apidom-parser-adapter-yaml-1-2';
import { refractorPluginReplaceEmptyElement, JSONSchemaElement } from '@swagger-api/apidom-ns-json-schema-draft-7';
const yamlDefinition = `
$schema: 'https://json-schema.org/draft-07/schema#'
if:
`;
const apiDOM = await parse(yamlDefinition);
const jsonSchemaElement = JSONSchemaElement.refract(apiDOM.result, {
plugins: [refractorPluginReplaceEmptyElement()],
});
// =>
// (JSONSchemaDraft7Element
// (MemberElement
// (StringElement)
// (StringElement))
// (MemberElement
// (StringElement)
// (JSONSchemaDraft7Element)))
// => without the plugin the result would be as follows:
// (JSONSchemaDraft7Element
// (MemberElement
// (StringElement)
// (StringElement))
// (MemberElement
// (StringElement)
// (StringElement)))
Only fully implemented specification objects should be checked here.
1.0.0-alpha.5 (2024-05-29)
FAQs
JSON Schema Draft 7 namespace for ApiDOM.
The npm package @swagger-api/apidom-ns-json-schema-draft-7 receives a total of 370,062 weekly downloads. As such, @swagger-api/apidom-ns-json-schema-draft-7 popularity was classified as popular.
We found that @swagger-api/apidom-ns-json-schema-draft-7 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.