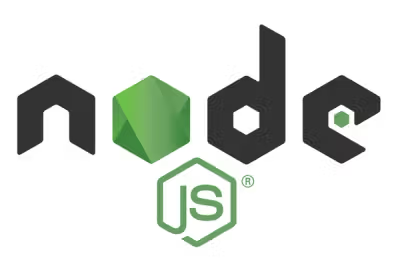
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
@swing.xyz/wallets
Advanced tools
Multi-chain wallet connection manager.
Install the package using your favorite package manager.
yarn add @swing.xyz/wallets
# OR
npm i @swing.xyz/wallets
The @swing.xyz/wallets
package is designed to work with the @swing.xyz/sdk and @swing.xyz/ui packages.
To use with @swing.xyz/sdk, you should provide the swingSDK
instance to the WalletProvider
.
import { SwingSDK } from '@swing.xyz/sdk';
import { WalletProvider } from '@swing.xyz/wallets/provider';
const swingSDK = new SwingSDK({
// Include your SwingSDK config
});
export default function App() {
return <WalletProvider swingSDK={swingSDK}>{/* The rest of your app code */}</WalletProvider>;
}
To use with @swing.xyz/ui, you should wrap the WalletProvider
in a SwingSdkProvider
. This ensures that the same swingSDK
instance is available to the WalletProvider
.
import { Swap, SwingSdkProvider, useSwingSdk } from '@swing.xyz/ui';
import { WalletProvider } from '@swing.xyz/wallets/provider';
export default function App() {
return (
<SwingSdkProvider>
<WalletProviderWrapper />
</SwingSdkProvider>
);
}
function WalletProviderWrapper() {
const swingSDK = useSwingSdk();
return (
<WalletProvider swingSDK={swingSDK}>
{/* The rest of your app code, such as the one of the @swing.xyz/ui widgets */}
<Swap />
</WalletProvider>
);
}
The @swing.xyz/wallets
package is built on top of Wagmi. It will automatically inherit any connectors you have configured in your Wagmi config.
To use with Wagmi, you need to wrap your WagmiProvider
around the WalletProvider
.
import { WagmiProvider } from 'wagmi';
import { WalletProvider } from '@swing.xyz/wallets/provider';
export default function App() {
return (
<WagmiProvider config={wagmiConfig}>
<WalletProvider swingSDK={swingSDK}>{/* The rest of your app code */}</WalletProvider>
</WagmiProvider>
);
}
Connectors are a small abstraction layer over different wallet providers.
You can add your own custom connectors by implementing the WalletConnector
interface.
// import { type WalletConnector } from '@swing.xyz/wallets/connectors';
interface WalletConnector {
/**
* Unquie identifier for the wallet
*/
id: string;
/**
* Wallet name
*/
name: string;
/**
* Wallet logo
*/
logo: string;
/**
* Type of the connector such as injected, walletconnect, etc.
*/
type: string;
/**
* Protocols supported by the wallet such as evm, ibc, solana, bitcoin, etc
*/
protocols: ProtocolType[];
/**
* Get the provider for the connector.
*/
getProvider: (chain?: Chain) => Promise<WalletProvider | undefined> | WalletProvider | undefined;
/**
* Connect to the wallet. If a chain is provided, the wallet will switch to the chain.
*/
connect: (chain?: Chain) => Promise<{ accounts: string[]; chainId: string | number }>;
/**
* Disconnect from the wallet
*/
disconnect?: () => Promise<void> | void;
/**
* Switch the current chain of the wallet
*/
switchChain?: (chain: Chain) => Promise<string[]> | Promise<void>;
}
The getConnectors
function returns an array of all the connectors.
import { getConnectors } from '@swing.xyz/wallets/connectors';
const connectors = getConnectors();
/**
* [
* {
* id: 'metamask',
* name: 'MetaMask',
* logo: 'https://metamask.io/favicon.ico',
* type: 'injected',
* protocols: ['evm'],
* }
* ]
**/
The getConnector
function returns a connector by ID.
import { getConnector } from '@swing.xyz/wallets/connectors';
const connector = getConnector('metamask');
/**
* {
* id: 'metamask',
* name: 'MetaMask',
* logo: 'https://metamask.io/favicon.ico',
* type: 'injected',
* protocols: ['evm'],
* }
**/
import type { WalletConnector } from '@swing.xyz/wallets/connectors';
import { WalletProvider } from '@swing.xyz/wallets/provider';
const customConnector: WalletConnector = {
id: 'custom',
name: 'Custom',
logo: 'custom-logo.png',
type: 'injected',
protocols: ['evm'], // Include the protocols supported by your custom wallet
getProvider: (chain) => window.ethereum,
connect: async (chain) => {
// Connect to the custom wallet
},
disconnect: async () => {
// (Optional) Disconnect from the custom wallet
},
switchChain: async (chain) => {
// (Optional) Switch the current chain of the custom wallet
},
};
export default function App() {
return <WalletProvider connectors={[customConnector]}>{/* The rest of your app code */}</WalletProvider>;
}
Connections are a representation of a wallet's connection to a chain.
// import { type WalletConnection } from '@swing.xyz/wallets/connections';
interface WalletConnection {
connector: WalletConnector;
chain: Chain;
accounts: string[];
}
The connect
function prompts the user to connect their wallet. If a chain is provided, the wallet will switch to the chain.
import { connect } from '@swing.xyz/wallets/connections';
// Connect using the wallet's current chain
const walletAddress = await connect({ connector });
// Connect to a specific chain using the chain's slug
const walletAddress = await connect({ connector, chainId: 'ethereum' });
// Connect to a specific chain using the chain's ID
const walletAddress = await connect({ connector, chainId: 1 });
The disconnect
function removes a wallet connection.
import { disconnect } from '@swing.xyz/wallets/connections';
await disconnect({ connector });
The getConnections
function returns an array of all the connections.
import { getConnections } from '@swing.xyz/wallets/connections';
const connections = getConnections();
/**
* [
* {
* accounts: ['0x123...', '0x456...'],
* chain: {
* id: 1,
* slug: 'ethereum',
* ...
* },
* connector: {
* id: 'metamask',
* ...,
* }
* }
* ]
**/
The getConnection
function returns a connection by wallet address.
import { getConnection } from '@swing.xyz/wallets/connections';
const connection = getConnection('0x123...');
/**
* {
* accounts: ['0x123...', '0x456...'],
* chain: {
* id: 1,
* slug: 'ethereum',
* ...
* },
* connector: {
* id: 'metamask',
* ...,
* }
* }
** /
The getConnectionForChain
function returns a connection for a given chain.
import { getConnectionForChain } from '@swing.xyz/wallets/connections';
const connection = getConnectionForChain('ethereum');
/**
* {
* accounts: ['0x123...', '0x456...'],
* chain: {
* id: 1,
* slug: 'ethereum',
* ...
* },
* connector: {
* id: 'metamask',
* ...,
* }
* }
**/
The useConnect
hook provides the connect() function to connect to a wallet. See the connect() function for more information.
import { useConnect } from '@swing.xyz/wallets/hooks/useConnect';
const connect = useConnect();
// Connect using the wallet's current chain
const walletAddress = await connect({ connector });
// Connect to a specific chain using the chain's slug
const walletAddress = await connect({ connector, chainId: 'ethereum' });
// Connect to a specific chain using the chain's ID
const walletAddress = await connect({ connector, chainId: 1 });
The useConnection
hook provides a function to get the current connection by wallet address.
import { useConnection } from '@swing.xyz/wallets/hooks/useConnection';
const connection = useConnection('0x123...');
The useConnections
hook provides a function to get all the connections.
import { useConnections } from '@swing.xyz/wallets/hooks/useConnections';
const connections = useConnections();
The useConnector
hook provides a function to get a connector by ID.
import { useConnector } from '@swing.xyz/wallets/hooks/useConnector';
const connector = useConnector('metamask');
The useConnectors
hook provides a function to get all the connectors.
import { useConnectors } from '@swing.xyz/wallets/hooks/useConnectors';
const connectors = useConnectors();
The following wallets are supported by default:
You can also add your own custom connectors to the WalletProvider
, and they will be available to all of the connector helpers such as the useConnectors() hook and getConnectors() functions.
If you would like your wallet to be added to our default wallet list, please open a PR adding your wallet to the src/connectors
folder.
FAQs
Unknown package
We found that @swing.xyz/wallets demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.