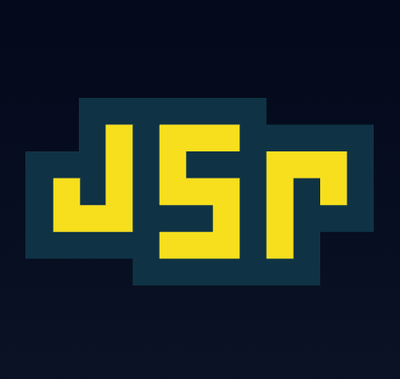
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@tanstack/react-router
Advanced tools
@tanstack/react-router is a powerful and flexible routing library for React applications. It provides a comprehensive set of features for managing navigation, route matching, and data fetching in a declarative manner.
Basic Routing
This feature allows you to define basic routes for your application. The `Router` component takes an array of route objects, each specifying a path and the component to render.
import { Router, Route } from '@tanstack/react-router';
const routes = [
{ path: '/', element: <Home /> },
{ path: '/about', element: <About /> },
];
function App() {
return (
<Router routes={routes} />
);
}
Nested Routes
Nested routes allow you to define routes within routes, enabling more complex and hierarchical navigation structures. The `children` property is used to specify nested routes.
import { Router, Route } from '@tanstack/react-router';
const routes = [
{ path: '/', element: <Home />, children: [
{ path: 'profile', element: <Profile /> },
{ path: 'settings', element: <Settings /> },
]},
];
function App() {
return (
<Router routes={routes} />
);
}
Route Parameters
Route parameters allow you to capture dynamic values from the URL. The `useParams` hook is used to access these parameters within your component.
import { Router, Route, useParams } from '@tanstack/react-router';
const routes = [
{ path: '/user/:id', element: <User /> },
];
function User() {
const { id } = useParams();
return <div>User ID: {id}</div>;
}
function App() {
return (
<Router routes={routes} />
);
}
Data Fetching
This feature allows you to fetch data before rendering a route. The `loader` function is used to fetch data, and the `useLoaderData` hook is used to access the fetched data within your component.
import { Router, Route, useLoaderData } from '@tanstack/react-router';
const routes = [
{ path: '/data', element: <DataComponent />, loader: fetchData },
];
async function fetchData() {
const response = await fetch('/api/data');
return response.json();
}
function DataComponent() {
const data = useLoaderData();
return <div>Data: {JSON.stringify(data)}</div>;
}
function App() {
return (
<Router routes={routes} />
);
}
react-router is one of the most popular routing libraries for React. It provides a similar set of features for managing navigation and route matching. However, @tanstack/react-router offers a more modern API and additional features like data fetching.
Next.js is a React framework that includes built-in routing capabilities. It offers file-based routing, server-side rendering, and static site generation. While it provides a more opinionated approach, it is less flexible than @tanstack/react-router for custom routing needs.
reach-router is a smaller, simpler alternative to react-router. It focuses on accessibility and simplicity. While it covers basic routing needs, it lacks some of the advanced features and flexibility provided by @tanstack/react-router.
FAQs
Modern and scalable routing for React applications
We found that @tanstack/react-router demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.