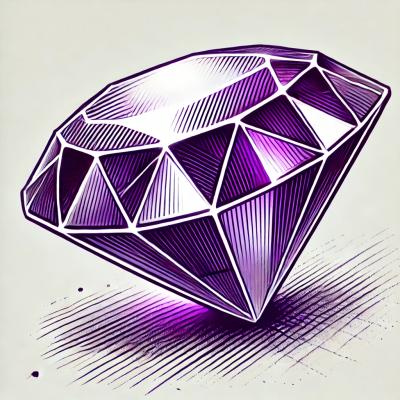
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@tapjs/test
Advanced tools
@tapjs/test is a testing framework for Node.js that provides a simple and powerful way to write and run tests. It is part of the TAP (Test Anything Protocol) ecosystem and is designed to be easy to use while offering a wide range of features for testing JavaScript code.
Basic Test
This feature allows you to write basic tests using the @tapjs/test framework. The example demonstrates a simple test that checks if 1 + 1 equals 2.
const t = require('@tapjs/test');
t.test('basic test', t => {
t.equal(1 + 1, 2, '1 + 1 should equal 2');
t.end();
});
Asynchronous Test
This feature supports asynchronous tests. The example shows how to write a test that waits for a promise to resolve and then checks the result.
const t = require('@tapjs/test');
t.test('async test', async t => {
const result = await Promise.resolve(42);
t.equal(result, 42, 'result should be 42');
t.end();
});
Nested Tests
This feature allows you to create nested tests. The example demonstrates a parent test containing a child test.
const t = require('@tapjs/test');
t.test('parent test', t => {
t.test('child test', t => {
t.ok(true, 'this is a child test');
t.end();
});
t.end();
});
Assertions
This feature provides various assertion methods. The example shows how to use different assertions like ok, equal, and notEqual.
const t = require('@tapjs/test');
t.test('assertions', t => {
t.ok(true, 'true is truthy');
t.equal(3, 3, '3 equals 3');
t.notEqual(3, 4, '3 does not equal 4');
t.end();
});
Mocha is a feature-rich JavaScript test framework running on Node.js and in the browser, making asynchronous testing simple and fun. It provides a variety of interfaces for writing tests, including BDD, TDD, and exports-style. Compared to @tapjs/test, Mocha offers more flexibility in terms of test interfaces and is widely used in the JavaScript community.
Jest is a delightful JavaScript testing framework with a focus on simplicity. It works out of the box for most JavaScript projects and provides a rich API for writing tests. Jest includes features like snapshot testing, a built-in mocking library, and code coverage reports. Compared to @tapjs/test, Jest is more opinionated and comes with more built-in features, making it a popular choice for React applications.
AVA is a test runner for Node.js with a concise API, detailed error output, and support for new language features. It runs tests concurrently, which can lead to faster test execution. Compared to @tapjs/test, AVA emphasizes simplicity and performance, making it a good choice for projects that require fast and straightforward testing.
@tapjs/test
The plugin-ified Test
class in node-tap.
FAQs
the pluggable Test class for node-tap
The npm package @tapjs/test receives a total of 59,056 weekly downloads. As such, @tapjs/test popularity was classified as popular.
We found that @tapjs/test demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.