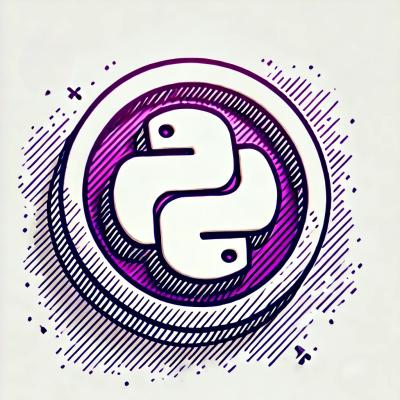
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
@tawasal/react
Advanced tools
check our Documentation 👉 platform.tawasal.ae
This library provides a set of React hooks to integrate with the Tawasal SuperApp API, allowing you to interact with various functionalities such as fetching user data, handling clipboard operations, scanning QR codes, and more.
npm i @tawasal/react
useTawasal
Fetches user information, user photo, and user link.
import React from 'react';
import { useTawasal } from '@tawasal/react';
const UserProfile = () => {
const { user, avatar, userLink, error, isLoading } = useTawasal();
if (isLoading) return <div>Loading...</div>;
if (error) return <div>Error: {error.message}</div>;
return (
<div>
{user && (
<div>
<h2>User Info</h2>
<p>Name: {user.name}</p>
<p>ID: {user.id}</p>
</div>
)}
{avatar && <img src={avatar} alt="User Photo" />}
{userLink && <p>User Link: {userLink}</p>}
</div>
);
};
export default UserProfile;
useContacts
Allows selecting contacts and fetching their details including avatar URLs.
import React, { useState } from 'react';
import { useContacts } from '@tawasal/react';
const ContactSelector = () => {
const { contacts, isLoading, error, triggerSelect } = useContacts();
return (
<div>
<button onClick={() => triggerSelect('Select a contact')}>
Select Contacts
</button>
{isLoading && <div>Loading...</div>}
{error && <div>Error: {error.message}</div>}
{contacts && (
<ul>
{contacts.map(contact => (
<li key={contact.userId}>
{contact.name} - {contact.photoUrl && <img src={contact.photoUrl} alt="Avatar" />}
</li>
))}
</ul>
)}
</div>
);
};
export default ContactSelector;
useClipboard
Reads the content of the clipboard.
import React from 'react';
import { useClipboard } from '@tawasal/react';
const ClipboardReader = () => {
const { clipboard, isLoading, error, updateClipboard } = useClipboard();
return (
<div>
<button onClick={updateClipboard}>Read Clipboard</button>
{isLoading && <div>Loading...</div>}
{error && <div>Error: {error.message}</div>}
{clipboard && <p>Clipboard Content: {clipboard}</p>}
</div>
);
};
export default ClipboardReader;
usePhoneNumber
Prompts the user to share their phone number.
import React from 'react';
import { usePhoneNumber } from '@tawasal/react';
const PhoneNumberPrompt = () => {
const { phone, isLoading, error, triggerPhonePrompt } = usePhoneNumber();
return (
<div>
<button onClick={() => triggerPhonePrompt('We need your phone number for verification')}>
Get Phone Number
</button>
{isLoading && <div>Loading...</div>}
{error && <div>Error: {error.message}</div>}
{phone && <p>Phone Number: {phone}</p>}
</div>
);
};
export default PhoneNumberPrompt;
useQR
Shows the QR code scanner and returns the scanned QR code.
import React, { useEffect } from 'react';
import { useQR } from '@tawasal/react';
const QRScanner = () => {
const {qr, isLoading, error, triggerScan, closeScan} = useQR();
useEffect(()=> {
// don't forget that your code and user can close scan. So if you recieved data you wanted
// from user, you can close scan faster then user to provide better UX.
if (!!qr) {
closeScan()
}
}, [qr])
return (
<div>
<button onClick={triggerScan}>Scan QR Code</button>
<button onClick={closeScan}>Close Scanner</button>
{isLoading && <div>Loading...</div>}
{error && <div>Error: {error.message}</div>}
{qr && <p>QR Code: {qr}</p>}
</div>
);
};
export default QRScanner;
useTawasalSDK
Provides additional Tawasal SDK functionalities such as haptic feedback, opening a destination, closing the app, and sharing content.
import React from 'react';
import { useTawasalSDK } from '@tawasal/react';
const TawasalActions = () => {
const { haptic, open, closeApp, share } = useTawasalSDK();
return (
<div>
<button onClick={() => haptic('light')}>Haptic Feedback</button>
<button onClick={() => open('destination')}>Open Destination</button>
<button onClick={closeApp}>Close App</button>
<button
onClick={() =>
share({
text: 'Check out this link!',
url: 'https://example.com',
})
}
>
Share
</button>
</div>
);
};
export default TawasalActions;
error: ESM packages need to be imported
add esm support to your next.js config
experimental: {
esmExternals: "loose"
}
Distributed under the MIT License. See LICENSE for more information.
FAQs
tawasal sdk for react development
The npm package @tawasal/react receives a total of 177 weekly downloads. As such, @tawasal/react popularity was classified as not popular.
We found that @tawasal/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.