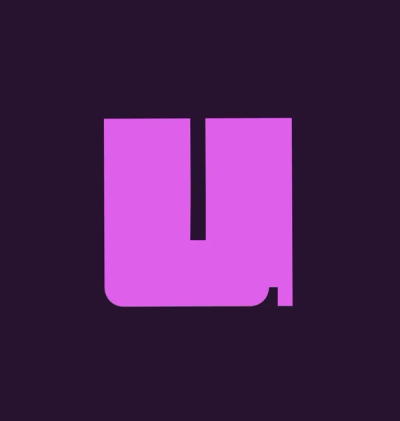
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
@tef-novum/webview-bridge
Advanced tools
JavaScript library to access to native functionality. Requires a webview with a postMessage bridge.
JavaScript library to access to native functionality. Requires a webview with a postMessage bridge.
Library size ~1.2 Kb (min + gzip)
AMD, UMD, IIFE, ES Module builds available (see package dist folder). Open an issue if you need a different build.
We recommend to manage your dependencies using npm
or yarn
and use a bundler
like webpack or parcel. Once
configured, you can use
ES imports.
Install using npm
:
npm i @tef-novum/webview-bridge
Install using yarn
:
yarn add @tef-novum/webview-bridge
Import required function and use it:
import {setWebViewTitle} from '@tef-novum/webview-bridge';
setWebViewTitle('Hello, world');
Alternatively, you can import the library directly from a CDN:
<script src="https://unpkg.com/@tef-novum/webview-bridge/dist/webview-bridge-iife.min.js"></script>
<script>
webviewBridge.setWebViewTitle('Hello, world');
</script>
Returns true if WebView Bridge is available. Use this function to implement fallbacks in case the bridge is not available.
isWebViewBridgeAvailable: () => boolean;
import {isWebViewBridgeAvailable, nativeAlert} from '@tef-novum/webview-bridge';
if (isWebViewBridgeAvailable()) {
nativeAlert('Hello'); // use bridge
} else {
myCustomAlert('Hello'); // use alternative implementation
}
Show native picker UI in order to let the user select a contact.
filter
property is ignored by iOS devicesrequestContact: ({filter?: 'phone' | 'email'}) => Promise<{
name?: string;
email?: string;
phoneNumber?: string;
address?: {
street?: string;
city?: string;
country?: string;
postalCode?: string;
};
}>;
All fields in response object are optional
import {requestContact} from '@tef-novum/webview-bridge';
requestContact({filter: 'phone'}).then((contact) => {
console.log(contact);
}).catch(err => {
console.error(err);
};
Inserts an event in calendar
createCalendarEvent: ({
beginTime: number,
endTime: number,
title: string
}) => Promise<void>;
beginTime
and endTime
are timestamps with millisecond precision
import {createCalendarEvent} from '@tef-novum/webview-bridge';
createCalendarEvent({
beginTime: new Date(2019, 10, 06).getTime(),
endTime: new Date(2019, 10, 07).getTime(),
title: "Peter's birthday",
}).then(() => {
console.log('event created');
}).catch(err => {
console.error(err);
};
Invokes the native sharing mechanism of the device.
type ShareOptions =
| {
text: string;
}
| {
url: string;
fileName: string;
text?: string;
};
share: (options: ShareOptions) => Promise<void>;
url
is present, text
is used as item to shareurl
param is present, it contains the URL to the shared filefileName
param is mandatory if url
is seturl
and text
are set, text
is used as Intent BODY
(if platform
allows it)import {share} from '@tef-novum/webview-bridge';
// sharing a text string
share({text: 'Hello, world!'});
// sharing a file
share({url: 'https://path/to/file', fileName: 'lolcats.png'});
Update webview title. If the bridge is not present, automatically fallbacks to a
document.title
update.
setWebViewTitle: (title: string) => Promise<void>;
import {setWebViewTitle} from '@tef-novum/webview-bridge';
setWebViewTitle('My new title');
Show a native confirm dialog.
If the bridge is not present (eg. showing the page in browser), fallbacks to a browser confirm.
nativeConfirm: ({
message: string;
title?: string;
acceptText?: string;
cancelText?: string;
}) => Promise<boolean>;
import {nativeConfirm} from '@tef-novum/webview-bridge';
nativeConfirm({
title: 'Confirm',
message: 'Send message?',
acceptText: 'Yes',
cancelText: 'No',
}).then(res => {
if (res) {
console.log('message sent');
}
});
Show a native alert dialog.
If the bridge is not present (eg. showing the page in browser), fallbacks to a browser alert.
nativeAlert: ({
message: string;
title?: string;
buttonText?: string;
}) => Promise<void>;
import {nativeAlert} from '@tef-novum/webview-bridge';
nativeAlert({
message: 'Purchase completed!',
title: 'Ok!',
}).then(res => {
console.log('alert closed');
});
Show a native message dialog. Use it to display feedback messages.
If the bridge is not present (eg. showing the page in browser), fallbacks to a browser alert.
buttonText
property is ignored in iOS.nativeMessage: ({
message: string;
duration?: number; // milliseconds
buttonText?: string; // Android only
type?: 'INFORMATIVE' | 'CRITICAL' | 'SUCCESS';
}) => Promise<void>;
Show a native "snackbar" with a configurable duration and optional close button
import {nativeMessage} from '@tef-novum/webview-bridge';
nativeMessage({
message: 'Operation finished!',
buttonText: 'Ok',
duration: 5000, // 5 seconds
}).then(res => {
console.log('alert closed');
});
Log an event to firebase
logEvent: ({
category: string; // Typically the object that was interacted with (e.g. 'Video')
action: string; // The type of interaction (e.g. 'play')
label?: string; // Useful for categorizing events (e.g. 'Fall Campaign')
value?: number; // A numeric value associated with the event (e.g. 43)
}) => Promise<void>;
import {logEvent} from '@tef-novum/webview-bridge';
logEvent({
category: 'topup-flow',
action: 'topup',
}).then(() => {
console.log('event logged');
});
Log the current screen name (or page name) to firebase
setScreenName: (screenName: string) => Promise<void>;
import {setScreenName} from '@tef-novum/webview-bridge';
setScreenName('Topup Flow').then(() => {
console.log('screen name logged');
});
If an error occurs, promise will be rejected with an error object:
{code: number, description: string}
This project is licensed under the terms of the MIT license. See the LICENSE file.
v.1.5.0 - 2019-04-05
webviewBrowserVersion
FAQs
JavaScript library to access to native functionality. Requires a webview with a postMessage bridge.
The npm package @tef-novum/webview-bridge receives a total of 876 weekly downloads. As such, @tef-novum/webview-bridge popularity was classified as not popular.
We found that @tef-novum/webview-bridge demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.