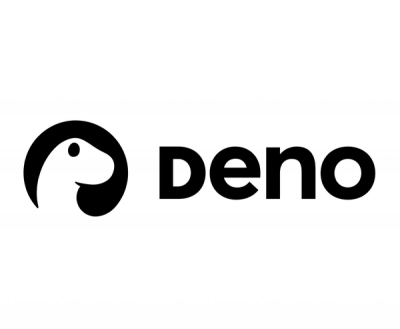
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@tensorflow/tfjs-core
Advanced tools
@tensorflow/tfjs-core is a JavaScript library for developing and training machine learning models in the browser and on Node.js. It provides a flexible and efficient way to perform numerical computations using tensors, which are the core data structure for machine learning.
Tensor Operations
This feature allows you to create and manipulate tensors, which are multi-dimensional arrays. The code sample demonstrates creating two tensors and performing an element-wise addition.
const tf = require('@tensorflow/tfjs-core');
const a = tf.tensor([1, 2, 3, 4]);
const b = tf.tensor([5, 6, 7, 8]);
const c = tf.add(a, b);
c.print();
Model Training
This feature allows you to define, compile, and train machine learning models. The code sample demonstrates creating a simple linear regression model, training it with some data, and making a prediction.
const tf = require('@tensorflow/tfjs-core');
const model = tf.sequential();
model.add(tf.layers.dense({units: 1, inputShape: [1]}));
model.compile({optimizer: 'sgd', loss: 'meanSquaredError'});
const xs = tf.tensor2d([1, 2, 3, 4], [4, 1]);
const ys = tf.tensor2d([1, 3, 5, 7], [4, 1]);
model.fit(xs, ys, {epochs: 10}).then(() => {
model.predict(tf.tensor2d([5], [1, 1])).print();
});
GPU Acceleration
This feature leverages WebGL to perform computations on the GPU, which can significantly speed up numerical operations. The code sample demonstrates a simple tensor addition, which will be accelerated if a GPU is available.
const tf = require('@tensorflow/tfjs-core');
const a = tf.tensor([1, 2, 3, 4]);
const b = tf.tensor([5, 6, 7, 8]);
const c = tf.add(a, b);
c.print();
ONNX.js is a JavaScript library for running ONNX (Open Neural Network Exchange) models in the browser and Node.js. It provides similar functionalities for running pre-trained models but focuses on the ONNX model format, which is widely used for interoperability between different machine learning frameworks.
Brain.js is a JavaScript library for neural networks, which can be used in Node.js and the browser. It provides a simpler API compared to @tensorflow/tfjs-core and is more focused on ease of use for common neural network tasks, but it may not offer the same level of flexibility and performance.
deeplearn.js is an open source hardware-accelerated JavaScript library for machine intelligence. deeplearn.js brings performant machine learning building blocks to the web, allowing you to train neural networks in a browser or run pre-trained models in inference mode.
We provide an API that closely mirrors the TensorFlow eager API. deeplearn.js was originally developed by the Google Brain PAIR team to build powerful interactive machine learning tools for the browser, but it can be used for everything from education, to model understanding, to art projects.
You can install this library via yarn/npm: yarn add deeplearn
or npm install deeplearn
Alternatively you can use a script tag. Here we load it from a CDN.
In this case it will be available as a global variable named dl
.
You can replace also specify which version to load replacing @latest
with a specific
version string (e.g. 0.5.0
).
<script src="https://cdn.jsdelivr.net/npm/deeplearn@latest"></script>
<!-- or -->
<script src="https://unpkg.com/deeplearn@latest"></script>
Let's add a scalar value to a 1D Tensor. Deeplearn js supports broadcasting the value of scalar over all the elements in the tensor.
import * as dl from 'deeplearn'; // If not loading the script as a global
const a = dl.tensor1d([1, 2, 3]);
const b = dl.scalar(2);
const result = a.add(b); // a is not modified, result is a new tensor
result.data().then(data => console.log(data)); // Float32Array([3, 4, 5]
// Alternatively you can use a blocking call to get the data.
// However this might slow your program down if called repeatedly.
console.log(result.dataSync()); // Float32Array([3, 4, 5]
See the TypeScript starter project and the ES6 starter project to get you quickly started.
Let's do the same thing in ES5 Javascript. Remember to include the script tag to load deeplearn.js
var a = dl.tensor1d([1, 2, 3]);
var b = dl.scalar(2);
var result = a.add(b); // a is not modified, result is a new tensor
// Option 1: With a Promise.
result.data().then(data => console.log(data)); // Float32Array([3, 4, 5])
// Option 2: Synchronous download of data. Blocks the UI.
console.log(result.dataSync());
To build deeplearn.js from source, we need to clone the project and prepare the dev environment:
$ git clone https://github.com/PAIR-code/deeplearnjs.git
$ cd deeplearnjs
$ yarn prep # Installs dependencies.
Generally speaking it's up to you. Yarn is fully interoperable with npm. You can either do yarn
or npm install
. However we use yarn, and if you are adding or removing dependencies you should use yarn to keep the yarn.lock
file up to date.
We recommend using Visual Studio Code for
development. Make sure to install
TSLint VSCode extension
and the npm clang-format 1.2.2
or later
with the
Clang-Format VSCode extension
for auto-formatting.
To interactively develop any of the demos (e.g. demos/nn-art/
):
$ ./scripts/watch-demo demos/nn-art
>> Starting up http-server, serving ./
>> Available on:
>> http://127.0.0.1:8080
>> Hit CTRL-C to stop the server
>> 1357589 bytes written to dist/demos/nn-art/bundle.js (0.85 seconds) at 10:34:45 AM
Then visit http://localhost:8080/demos/nn-art/
. The
watch-demo
script monitors for changes of typescript code and does
incremental compilation (~200-400ms), so users can have a fast edit-refresh
cycle when developing apps.
Before submitting a pull request, make sure the code passes all the tests and is clean of lint errors:
$ yarn test
$ yarn lint
To run a subset of tests and/or on a specific browser:
$ yarn test --browsers=Chrome --grep='multinomial'
> ...
> Chrome 62.0.3202 (Mac OS X 10.12.6): Executed 28 of 1891 (skipped 1863) SUCCESS (6.914 secs / 0.634 secs)
To run the tests once and exit the karma process (helpful on Windows):
$ yarn test --single-run
To build a standalone ES5 library that can be imported in the browser with a
<script>
tag:
$ ./scripts/build-standalone.sh # Builds standalone library.
>> Stored standalone library at dist/deeplearn(.min).js
To build an npm package:
$ ./scripts/build-npm.sh
...
Stored standalone library at dist/deeplearn(.min).js
deeplearn-VERSION.tgz
To install it locally, run npm install ./deeplearn-VERSION.tgz
.
On Windows, use bash (available through git) to use the scripts above.
Looking to contribute, and don't know where to start? Check out our "help wanted" issues.
deeplearn.js targets environments with WebGL 1.0 or WebGL 2.0. For devices
without the OES_texture_float
extension, we fall back to fixed precision
floats backed by a gl.UNSIGNED_BYTE
texture. For platforms without WebGL,
we provide CPU fallbacks.
FAQs
Hardware-accelerated JavaScript library for machine intelligence
The npm package @tensorflow/tfjs-core receives a total of 279,885 weekly downloads. As such, @tensorflow/tfjs-core popularity was classified as popular.
We found that @tensorflow/tfjs-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.