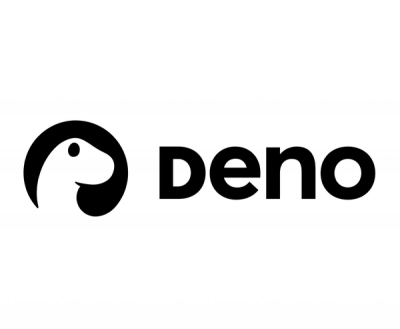
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@tensorflow/tfjs-layers
Advanced tools
@tensorflow/tfjs-layers is a high-level API for building and training machine learning models in JavaScript. It is part of the TensorFlow.js ecosystem and provides a way to create neural networks using layers, which are the building blocks of deep learning models. This package allows you to define, train, and evaluate models directly in the browser or in Node.js.
Creating a Sequential Model
This feature allows you to create a sequential model, which is a linear stack of layers. The code sample demonstrates how to add dense layers to the model.
const tf = require('@tensorflow/tfjs-layers');
const model = tf.sequential();
model.add(tf.layers.dense({units: 32, inputShape: [50]}));
model.add(tf.layers.dense({units: 10, activation: 'softmax'}));
Compiling a Model
This feature allows you to compile the model, specifying the optimizer, loss function, and metrics to be used during training. The code sample shows how to compile a model with stochastic gradient descent (SGD) optimizer and categorical cross-entropy loss.
model.compile({
optimizer: 'sgd',
loss: 'categoricalCrossentropy',
metrics: ['accuracy']
});
Training a Model
This feature allows you to train the model using the fit method. The code sample demonstrates how to train the model with random data for 10 epochs and a batch size of 32.
const xs = tf.randomNormal([100, 50]);
const ys = tf.randomUniform([100, 10]);
model.fit(xs, ys, {
epochs: 10,
batchSize: 32
}).then(history => {
console.log(history.history);
});
Evaluating a Model
This feature allows you to evaluate the model's performance on test data. The code sample shows how to evaluate the model using random test data.
const testXs = tf.randomNormal([20, 50]);
const testYs = tf.randomUniform([20, 10]);
model.evaluate(testXs, testYs).print();
Making Predictions
This feature allows you to make predictions using the trained model. The code sample demonstrates how to make a prediction with a single input sample.
const input = tf.randomNormal([1, 50]);
const prediction = model.predict(input);
prediction.print();
Keras is a high-level neural networks API, written in Python and capable of running on top of TensorFlow, CNTK, or Theano. It is designed to enable fast experimentation with deep neural networks. Keras is similar to @tensorflow/tfjs-layers in that it provides a high-level interface for building and training models, but it is primarily used in Python environments.
Brain.js is a JavaScript library for neural networks, which is also capable of running in the browser or in Node.js. It provides a simpler interface compared to @tensorflow/tfjs-layers and is more suitable for smaller-scale neural network tasks. While it offers similar functionalities, it does not have the same level of flexibility and performance as TensorFlow.js.
Synaptic is a JavaScript neural network library for node.js and the browser. It provides a wide range of neural network architectures and is designed to be highly flexible. However, it is not as optimized for performance as @tensorflow/tfjs-layers and does not integrate as seamlessly with the broader TensorFlow ecosystem.
A part of the TensorFlow.js ecosystem, TensorFlow.js Layers is a high-level API built on TensorFlow.js Core, enabling users to build, train and execute deep learning models in the browser. TensorFlow.js Layers is modeled after Keras and tf.keras and can load models saved from those libraries.
There are three ways to import TensorFlow.js Layers
tfjs-layers
has peer dependency on tfjs-core, so if you import
@tensorflow/tfjs-layers
, you also need to import
@tensorflow/tfjs-core
.Option 1 is the most convenient, but leads to a larger bundle size (we will be adding more packages to it in the future). Use option 2 if you care about bundle size.
The following example shows how to build a toy model with only one dense
layer
to perform linear regression.
import * as tf from '@tensorflow/tfjs';
// A sequential model is a container which you can add layers to.
const model = tf.sequential();
// Add a dense layer with 1 output unit.
model.add(tf.layers.dense({units: 1, inputShape: [1]}));
// Specify the loss type and optimizer for training.
model.compile({loss: 'meanSquaredError', optimizer: 'SGD'});
// Generate some synthetic data for training.
const xs = tf.tensor2d([[1], [2], [3], [4]], [4, 1]);
const ys = tf.tensor2d([[1], [3], [5], [7]], [4, 1]);
// Train the model.
await model.fit(xs, ys, {epochs: 500});
// Ater the training, perform inference.
const output = model.predict(tf.tensor2d([[5]], [1, 1]));
output.print();
You can also load a model previously trained and saved from elsewhere (e.g., from Python Keras) and use it for inference or transfer learning in the browser.
For example, in Python, save your Keras model using
tensorflowjs,
which can be installed using pip install tensorflowjs
.
import tensorflowjs as tfjs
# ... Create and train your Keras model.
# Save your Keras model in TensorFlow.js format.
tfjs.converters.save_keras_model(model, '/path/to/tfjs_artifacts/')
# Then use your favorite web server to serve the directory at a URL, say
# http://foo.bar/tfjs_artifacts/model.json
To load the model with TensorFlow.js Layers:
import * as tf from '@tensorflow/tfjs';
const model = await tf.loadLayersModel('http://foo.bar/tfjs_artifacts/model.json');
// Now the model is ready for inference, evaluation or re-training.
FAQs
TensorFlow layers API in JavaScript
We found that @tensorflow/tfjs-layers demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.