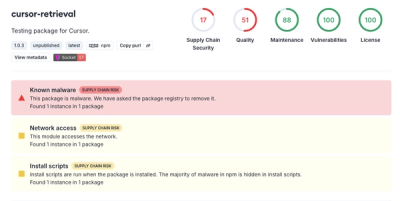
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@terminal-packages/sdk
Advanced tools
| Setup Guide | Basic Wallet Providers | MetaMask Provider | Custom Http Providers | Websockets | Basic RPC Endpoints |
Terminal is an developers platform for builing on Ethereum. Develop, test, and manage Ethereum artifacts and infrastructure in a unified workspace with Terminal. The Terminal toolkit provides developers with a broad range of utilities to construct robust and user-friendly decentralized applications or dapps.
TerminalSDK allows you to surface logs from arbitrary RPC endpoints and networks to your Terminal account. TerminalSDK is a simple drop-in wrapper around any Web3 provider with minimal configuration required on your part to get up and running.
To install, do the following:
npm:
$ npm install @terminal-packages/sdk
yarn:
$ yarn add @terminal-packages/sdk
Now that you've installed the sdk, you will need to signup for a Terminal account and generate an API key. You can make an account by going to https://terminal.co and clicking 'signup'. Once you have made an account, you can easily generate an API key by clicking on the profile icon in the top right corner and clicking 'settings'. You can then generate a new API key at the bottom of the page.
Once you've signed up and generated an API key, you will be able to wrap any external source in the TerminalSDK and surface logs. Choose which type of external source you'd like to wrap first and follow the instructions below or at the tech docs. If you are trying to get MetaMask logs, you can go ahead and skip to the bottom of the page or click here.
Now that you have wrapped your desired external source in the TerminalSDK, navigate to the logs page and check that your newly added source is surfacing logs. We recommend making a few test calls to each source in order to ensure it is working properly. If you are having trouble setting up logs for your external sources, please feel free to reach out on our Discord.
host: Any ethereum provider url of your choice
apiKey: An API key associated with your Terminal account
source: Any custom provider of your choice
*source can be any dynamic string of your choice or use one of the basic sourceTypes listed below
View changelog
View develop-docs
Hooking this custom provider up is super easy and once hooked up it will push all JSON-RPC calls to your logs in your Terminal account.
Due to the rewrite of web3 for 2.0 the code on web3 1 vs web3 2 is very different, this means we have to go down different paths of code depending on the version you use. We made this super easy for you to tell us in the examples below. By default if you do not supply it then it will default to 2.0. Please note if your on 1.0-beta
version you do not need to supply the web3
version.
https://www.npmjs.com/package/web3
export enum SourceType {
Terminal = 'Terminal',
Truffle = 'Truffle',
Alchemy = 'Alchemy',
MetaMask = 'MetaMask',
Infura = 'Infura',
Pocket = 'Pocket',
Ledger = 'Ledger',
Web3ProviderEngine = 'Web3ProviderEngine',
WalletLink = 'WalletLink',
TruffleHDWalletProvider = 'TruffleHDWalletProvider',
Portis = 'Portis'
}
Please note if your on 1.0-beta
then Web3Versions.two
is the correct version.
export enum Web3Versions {
one = '1',
two = '2'
}
import { TerminalHttpProvider, SourceType } from '@terminal-packages/sdk';
import Web3 from 'web3';
const web3 = new Web3(
new TerminalHttpProvider({
host: 'https://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one
})
);
const sdk = require('@terminal-packages/sdk');
const Web3 = require('web3');
const web3 = new Web3(
new sdk.TerminalHttpProvider({
host: 'https://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one
})
);
import { TerminalHttpProvider, SourceType } from '@terminal-packages/sdk';
import Web3 from 'web3';
const web3 = new Web3(
new TerminalHttpProvider({
host: 'https://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: "YOUR_TERMINAL_PROJECT_ID",
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
// options is not required
options: {
// timeout is not required
timeout: 10000,
// headers is not required
headers: [{ name: 'x-custom-header' value: 'example' }],
// with credentials is not required
withCredentials: true
}
})
);
const sdk = require('@terminal-packages/sdk');
const Web3 = require('web3');
const web3 = new Web3(
new sdk.TerminalHttpProvider({
host: 'https://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: "YOUR_TERMINAL_PROJECT_ID",
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one,
// options is not required
options: {
// timeout is not required
timeout: 10000,
// headers is not required
headers: [{ name: 'x-custom-header' value: 'example' }],
// with credentials is not required
withCredentials: true
}
})
);
If you're using your own custom provider it should be following the standard HttpProvider
interface which exposes a property called host
on your provider itself. Without this we do not know the nodeUrl
which means you don't get that information when you go and view your logs. If you want to log the nodeUrl
as well you just need to expose a host
property on your provider and the sdk will grab it and start saving all logs with that nodeUrl
as that property.
import { TerminalHttpProvider, SourceType } from '@terminal-packages/sdk';
import Web3 from 'web3';
const web3 = new Web3(
new TerminalHttpProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
customHttpProvider: new YourCustomHttpProvider()
})
);
const sdk = require('@terminal-packages/sdk');
const Web3 = require('web3');
const web3 = new Web3(
new sdk.TerminalHttpProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one,
customHttpProvider: new YourCustomHttpProvider()
})
);
https://www.npmjs.com/package/ethers
export enum SourceType {
Terminal = 'Terminal',
Truffle = 'Truffle',
Alchemy = 'Alchemy',
MetaMask = 'MetaMask',
Infura = 'Infura',
Pocket = 'Pocket',
Ledger = 'Ledger',
Web3ProviderEngine = 'Web3ProviderEngine',
WalletLink = 'WalletLink',
TruffleHDWalletProvider = 'TruffleHDWalletProvider',
Portis = 'Portis'
}
Please note if your on 1.0-beta
then Web3Versions.two
is the correct version if you ever want to supply the Web3Versions
to a call.
export enum Web3Versions {
one = '1',
two = '2'
}
import { TerminalHttpProvider, SourceType } from '@terminal-packages/sdk';
import { ethers } from 'ethers';
const provider = new ethers.providers.Web3Provider(
new TerminalHttpProvider({
host: 'https://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one
})
);
const sdk = require('@terminal-packages/sdk');
const ethers = require('ethers');
const provider = new ethers.providers.Web3Provider(
new sdk.TerminalHttpProvider({
host: 'https://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one
})
);
import { TerminalHttpProvider, SourceType } from '@terminal-packages/sdk';
import { ethers } from 'ethers';
const provider = new ethers.providers.Web3Provider(
new TerminalHttpProvider({
host: 'https://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: "YOUR_TERMINAL_PROJECT_ID",
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
// options is not required
options: {
// timeout is not required
timeout: 10000,
// headers is not required
headers: [{ name: 'x-custom-header' value: 'example' }],
// with credentials is not required
withCredentials: true
}
})
);
const sdk = require('@terminal-packages/sdk');
const ethers = require('ethers');
const provider = new ethers.providers.Web3Provider(
new sdk.TerminalHttpProvider({
host: 'https://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: "YOUR_TERMINAL_PROJECT_ID",
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one,
// options is not required
options: {
// timeout is not required
timeout: 10000,
// headers is not required
headers: [{ name: 'x-custom-header' value: 'example' }],
// with credentials is not required
withCredentials: true
}
})
);
If you're using your own custom provider it should be following the standard HttpProvider
interface which exposes a property called host
on your provider itself. Without this we do not know the nodeUrl
which means you don't get that information when you go and view your logs. If you want to log the nodeUrl
as well you just need to expose a host
property on your provider and the sdk will grab it and start saving all logs with that nodeUrl
as that property.
import { TerminalHttpProvider } from '@terminal-packages/sdk';
import { ethers } from 'ethers';
const provider = new ethers.providers.Web3Provider(
new TerminalHttpProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
customHttpProvider: new YourCustomHttpProvider()
})
);
const sdk = require('@terminal-packages/sdk');
const ethers = require('ethers');
const provider = new ethers.providers.Web3Provider(
new sdk.TerminalHttpProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one,
customHttpProvider: new YourCustomHttpProvider()
})
);
Please note that if you're using MetaMask please follow the guide here.
Our provider handle any injected custom provider in the window. If you're using a 3rd party wallet which injects the custom provider in the window.ethereum
you can use that with our provider. All you have to do is pass in the window.ethereum
into the customHttpProvider
option within the TerminalHttpProvider
class. Example below shows how you would do this:
import { TerminalHttpProvider, SourceType } from '@terminal-packages/sdk';
import Web3 from 'web3';
const web3 = new Web3(
new TerminalHttpProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
customHttpProvider: window.ethereum
})
);
import { TerminalHttpProvider, SourceType } from '@terminal-packages/sdk';
import { ethers } from 'ethers';
const provider = new ethers.providers.Web3Provider(
new TerminalHttpProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
customHttpProvider: window.ethereum
})
);
Due to the rewrite of web3 for 2.0 the code on web3 1 vs web3 2 is very different, this means we have to go down different paths of code depending on the version you use. We made this super easy for you to tell us in the examples below. By default if you do not supply it then it will default to 2.0. Please note if your on 1.0-beta
version you do not need to supply the web3
version.
https://www.npmjs.com/package/web3
export enum SourceType {
Terminal = 'Terminal',
Truffle = 'Truffle',
Alchemy = 'Alchemy',
MetaMask = 'MetaMask',
Infura = 'Infura',
Pocket = 'Pocket',
Ledger = 'Ledger',
Web3ProviderEngine = 'Web3ProviderEngine',
WalletLink = 'WalletLink',
TruffleHDWalletProvider = 'TruffleHDWalletProvider',
Portis = 'Portis'
}
Please note if your on 1.0-beta
then Web3Versions.two
is the correct version if you ever want to supply the Web3Versions
to a call.
export enum Web3Versions {
one = '1',
two = '2'
}
import {
TerminalWebsocketProvider,
SourceType,
Web3Versions
} from '@terminal-packages/sdk';
import Web3 from 'web3';
const web3 = new Web3(
new TerminalWebsocketProvider({
host: 'wss://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one
})
);
const sdk = require('@terminal-packages/sdk');
const Web3 = require('web3');
const web3 = new Web3(
new sdk.TerminalWebsocketProvider({
host: 'wss://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one
})
);
import { TerminalWebsocketProvider, SourceType, Web3Versions } from '@terminal-packages/sdk';
import Web3 from 'web3';
const web3 = new Web3(
new TerminalWebsocketProvider({
host: 'wss://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: "YOUR_TERMINAL_PROJECT_ID",
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
// options not required
options: {
// timeout not required
timeout: 10000,
// headers not required
headers: [{ name: 'x-custom-header' value: 'example' }],
// protocol not required
protocol: '63',
// client config not required
clientConfig: {
maxReceivedFrameSize: 100000000,
maxReceivedMessageSize: 100000000
},
}
})
);
const sdk = require('@terminal-packages/sdk');
const Web3 = require('web3');
const web3 = new Web3(
new sdk.TerminalWebsocketProvider({
host: 'wss://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: "YOUR_TERMINAL_PROJECT_ID",
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one,
// options not required
options: {
// timeout not required
timeout: 10000,
// headers not required
headers: [{ name: 'x-custom-header' value: 'example' }],
// protocol not required
protocol: '63',
// client config not required
clientConfig: {
maxReceivedFrameSize: 100000000,
maxReceivedMessageSize: 100000000
},
}
})
);
If you're using your own custom provider we look for a property called host
on your provider itself. Without this we do not know the nodeUrl
which means you don't get that information when you go and view your logs. If you want to log the nodeUrl
as well you just need to expose a host
property on your provider and the sdk will grab it and start saving all logs with that nodeUrl
as that property.
import {
TerminalHttpProvider,
SourceType,
Web3Versions
} from '@terminal-packages/sdk';
import Web3 from 'web3';
const web3 = new Web3(
new TerminalWebsocketProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
customWebsocketProvider: new YourCustomWebsocketProvider()
})
);
const sdk = require('@terminal-packages/sdk');
const Web3 = require('web3');
const web3 = new Web3(
new sdk.TerminalWebsocketProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one,
customWebsocketProvider: new YourCustomWebsocketProvider()
})
);
https://www.npmjs.com/package/ethers
export enum SourceType {
Terminal = 'Terminal',
Truffle = 'Truffle',
Alchemy = 'Alchemy',
MetaMask = 'MetaMask',
Infura = 'Infura',
Pocket = 'Pocket',
Ledger = 'Ledger',
Web3ProviderEngine = 'Web3ProviderEngine',
WalletLink = 'WalletLink',
TruffleHDWalletProvider = 'TruffleHDWalletProvider',
Portis = 'Portis'
}
Please note if your on 1.0-beta
then Web3Versions.two
is the correct version if you ever want to supply the Web3Versions
to a call.
export enum Web3Versions {
one = '1',
two = '2'
}
import {
TerminalWebsocketProvider,
SourceType,
Web3Versions
} from '@terminal-packages/sdk';
import { ethers } from 'ethers';
const provider = new ethers.providers.Web3Provider(
new TerminalWebsocketProvider({
host: 'wss://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one
})
);
const sdk = require('@terminal-packages/sdk');
const ethers = require('ethers');
const provider = new ethers.providers.Web3Provider(
new sdk.TerminalWebsocketProvider({
host: 'wss://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one
})
);
import { TerminalWebsocketProvider, SourceType, Web3Versions } from '@terminal-packages/sdk';
import { ethers } from 'ethers';
const web3 = new ethers.providers.Web3Provider(
new TerminalWebsocketProvider({
host: 'wss://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: "YOUR_TERMINAL_PROJECT_ID",
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
// options not required
options: {
// timeout not required
timeout: 10000,
// headers not required
headers: [{ name: 'x-custom-header' value: 'example' }],
// protocol not required
protocol: '63',
// client config not required
clientConfig: {
maxReceivedFrameSize: 100000000,
maxReceivedMessageSize: 100000000
},
}
})
);
const sdk = require('@terminal-packages/sdk');
const ethers = require('ethers');
const web3 = new ethers.providers.Web3Provider(
new sdk.TerminalWebsocketProvider({
host: 'wss://yourethnodeurl.io',
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: "YOUR_TERMINAL_PROJECT_ID",
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one,
// options not required
options: {
// timeout not required
timeout: 10000,
// headers not required
headers: [{ name: 'x-custom-header' value: 'example' }],
// protocol not required
protocol: '63',
// client config not required
clientConfig: {
maxReceivedFrameSize: 100000000,
maxReceivedMessageSize: 100000000
},
}
})
);
If you're using your own custom provider we look for a property called host
on your provider itself. Without this we do not know the nodeUrl
which means you don't get that information when you go and view your logs. If you want to log the nodeUrl
as well you just need to expose a host
property on your provider and the sdk will grab it and start saving all logs with that nodeUrl
as that property.
import {
TerminalWebsocketProvider,
SourceType,
Web3Versions
} from '@terminal-packages/sdk';
import { ethers } from 'ethers';
const web3 = new ethers.providers.Web3Provider(
new TerminalWebsocketProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
customWebsocketProvider: new YourCustomWebsocketProvider()
})
);
const sdk = require('@terminal-packages/sdk');
const ethers = require('ethers');
const web3 = new ethers.providers.Web3Provider(
new sdk.TerminalWebsocketProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: sdk.SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: sdk.Web3Versions.one,
customWebsocketProvider: new YourCustomWebsocketProvider()
})
);
Please note that if you're using MetaMask please follow the guide here and they only support http provider.
Our provider handle any injected custom provider in the window. If you're using a 3rd party wallet which injects the custom provider in the window.ethereum
you can use that with our websocket provider. All you have to do is pass in the window.ethereum
into the customWebsocketProvider
option within the TerminalWebsocketProvider
class. Please note your window.ethereum
provider must support websockets for this to work. Example below shows how you would do this:
import {
TerminalWebsocketProvider,
SourceType,
Web3Versions
} from '@terminal-packages/sdk';
import Web3 from 'web3';
const web3 = new Web3(
new TerminalWebsocketProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
customWebsocketProvider: window.ethereum
})
);
import {
TerminalWebsocketProvider,
SourceType,
Web3Versions
} from '@terminal-packages/sdk';
import { ethers } from 'ethers';
const provider = new ethers.providers.Web3Provider(
new TerminalWebsocketProvider({
apiKey: 'yourApiKey',
// projectId is not required to log but we suggest
// using it for the best experience
projectId: 'YOUR_TERMINAL_PROJECT_ID',
// source can be a dynamic string as well
source: SourceType.Terminal,
// if your using web3 1 (NONE BETA) please tell us, if you're not please delete this property
web3Version: Web3Versions.one,
customWebsocketProvider: window.ethereum
})
);
export interface IMetaMaskOptions {
apiKey: string;
projectId?: string | undefined;
}
Please note that if you're only using the logging MetaMask
feature, you do not need to install the sdk
as well.
To get all the amazing logging in your dapp, all you need to do is paste this script within the <head>
tag of your website:
<!-- TERMINAL SCRIPTS -->
<script src="https://storage.googleapis.com/terminal-sdk/metamask/latest/metamask-latest.min.js"></script>
<script type="text/JavaScript">
window.terminal.sdk.metamask.startLogging({apiKey: 'YOUR_API_KEY', projectId: 'YOUR_PROJECT_ID'});
</script>
<!-- END TERMINAL SCRIPTS -->
This will expose a window.terminal.ethereum
which you can inject into any web3 instance
import Web3 from 'web3';
const web3 = new Web3(window.terminal.ethereum);
For legacy dapps which still use window.web3
injected by MM using that will start logging everything. We highly recommend not doing this as MM use 0.2.x web3 which uses callbacks. Using the above approach and using 1.x.x or 2.x.x will allow you to use promises.
If you want to use a fixed version of the MetaMask
script you can. We deploy every version of the script to the CDN store against the same version as the sdk
when that's deployed. You can hook in those fixed versions by replacing {{versionInfo}}
with your version number you want to use.
<script src="https://storage.googleapis.com/terminal-sdk/metamask/{{versionNumber}}/metamask-{{versionNumber}}.min.js"></script>
FAQs
Ethereum developer tool kit for the Terminal platform
We found that @terminal-packages/sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.