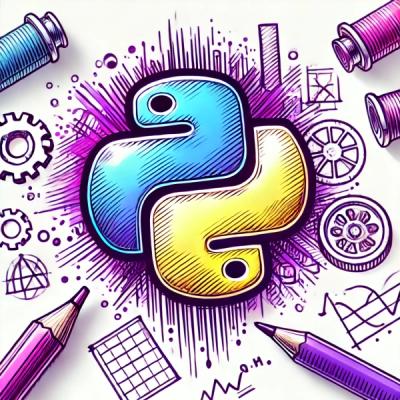
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
@therms/future-queue
Advanced tools
Schedule messages to be processed in the future.
This is not meant to be a full-featured queue service, but rather a simple service that can be used to schedule messages to be processed in the future.
This service is useful when you want to schedule a message to be processed in the future. For example, you may want to send a reminder email to a user in 3 days. You can use this service to schedule the message to be sent in 3 days.
When the message is ready to be processed an implementation use-case would be to send the message to a queue service such as RabbitMQ or Kafka where your application queue service handles the message.
This implementation was tested to gain an idea of performance.
1 CPU, 2GB RAM Digital Ocean
10k
messages with 0.1kb
data array processed in ~17 seconds.2 CPU, 4GB RAM Digital Ocean
10k
messages with 5kb
data array processed in ~27 seconds.
const queue = new FutureQueue(
{
queueName: 'test-queue',
},
{
mongo: {
uri: global.__MONGO_URI__,
},
}
)
await queue.connectionReady
const fiveMinFromNow = new Date().setMinutes(fiveMinFromNow.getMinutes() + 5)
await queue.add(
{
data: { test: 'test' },
key: 'test-key',
},
{
readyTime: fiveMinFromNow,
},
)
await queue.add(
[
{
data: { test: 'test' },
key: 'test-key-2',
},
{
data: { test: 'test' },
key: 'test-key-3',
},
],
{
readyTime: fiveMinFromNow,
},
)
A message can be set with an optional expireTime
which will remove the message
from the queue if it is not processed before the expireTime
.
await queue.add(
{
data: { test: 'test' },
key: 'test-key',
},
{
expireTime: tenMinFromNow,
readyTime: fiveMinFromNow,
},
)
You can process messages that are ready by providing a handler to the constructor. This allows you to have instances that are designated for processing messages (ie: a background server or thread) and instances that are designated for adding and interacting with messages in the queue.
const queue = new FutureQueue(
{
queueName: 'test-queue',
},
{
mongo: {
uri: global.__MONGO_URI__,
},
},
{
onMessageReady: async (message: ReadyQueueMessage) => {/*...*/}
}
)
Messages will be processed in the order they are ready. If a message is not
processed within the message expireTime
it will be removed from the queue and
will not be processed.
You can get, cancel or update messages by their key. You must provide the key when adding a message to the queue.
await queue.add(
{
data: { test: 'test' },
key: 'test-key',
},
{
readyTime: fiveMinFromNow,
},
)
const message = await queue.getByKey('test-key')
// Cancel the message (effectively removing it from the queue)
await queue.cancelByKey('test-key')
// Update the message data or expire/ready time
await queue.updateByKey(
'test-key',
{
expireTime: new Date(),
readyTime: new Date(),
data: { test: 'new data' }
},
{
throwIfNotFound: false
}
)
FAQs
A MongoDB queue service for Node.js with scheduling and retrying
We found that @therms/future-queue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.