

This is a standalone project, maintained as part of the
@thi.ng/umbrella monorepo and
anti-framework.
About
Function memoization with configurable caching.
This package provides different function memoization implementations for
functions with 1 or more arguments and custom result caching using ES6
Map API like implementations. Unlike native ES6 Maps, the
implementations MUST support value, not just referential, equality
semantics (e.g. those provided by
@thi.ng/associative)
or
@thi.ng/cache).
The latter also support automatically pruning of memoization caches,
based on different strategies. See doc strings for further details.
Status
STABLE - used in production
Search or submit any issues for this package
Related packages
- @thi.ng/cache - In-memory cache implementations with ES6 Map-like API and different eviction strategies
Installation
yarn add @thi.ng/memoize
ES module import:
<script type="module" src="https://cdn.skypack.dev/@thi.ng/memoize"></script>
Skypack documentation
For Node.js REPL:
const memoize = await import("@thi.ng/memoize");
Package sizes (brotli'd, pre-treeshake): ESM: 247 bytes
Dependencies
Usage examples
Several projects in this repo's
/examples
directory are using this package:
Screenshot | Description | Live demo | Source |
---|
| Isolated, component-local DOM updates | Demo | Source |
| Basic rstream-gestures multi-touch demo | Demo | Source |
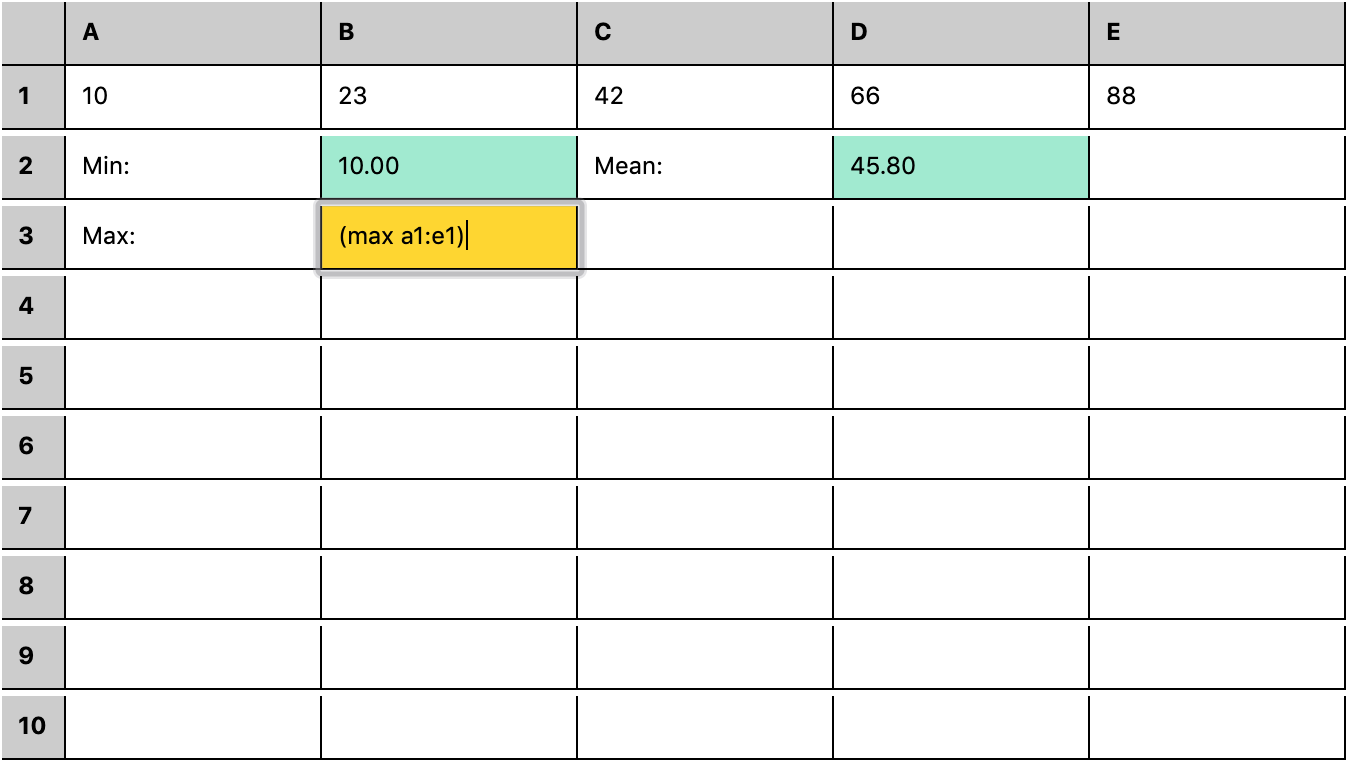 | rstream based spreadsheet w/ S-expression formula DSL | Demo | Source |
API
Generated API docs
import * as m from "@thi.ng/memoize";
import { EquivMap } from "@thi.ng/associative";
import { LRUCache } from "@thi.ng/cache";
Optimized version for single arg functions
foo = m.memoize1((x) => (console.log("exec"), x * 10));
foo(1);
foo(1);
foo = m.memoize1(
(x) => (console.log("exec"), x[0] * 10),
new EquivMap()
);
foo([1]);
foo([1]);
foo = m.memoize1(
(x) => (console.log("exec"), x[0] * 10),
new LRUCache(null, { maxlen: 3 })
);
Arbitrary args
dotProduct = m.memoize(
(x, y) => (console.log("exec"), x[0] * y[0] + x[1] * y[1]),
new EquivMap()
);
dotProduct([1,2], [3,4]);
dotProduct([1,2], [3,4]);
Via JSON.stringify()
dotProduct = m.memoizeJ(
(x, y) => (console.log("exec"), x[0] * y[0] + x[1] * y[1])
);
dotProduct([1,2], [3,4]);
dotProduct([1,2], [3,4]);
Authors
If this project contributes to an academic publication, please cite it as:
@misc{thing-memoize,
title = "@thi.ng/memoize",
author = "Karsten Schmidt",
note = "https://thi.ng/memoize",
year = 2018
}
License
© 2018 - 2023 Karsten Schmidt // Apache License 2.0