

[!NOTE]
This is one of 199 standalone projects, maintained as part
of the @thi.ng/umbrella monorepo
and anti-framework.
🚀 Please help me to work full-time on these projects by sponsoring me on
GitHub. Thank you! ❤️
About
Function memoization with configurable caching.
This package provides different function memoization implementations for
functions with arbitrary arguments and custom result caching using ES6
Map API like implementations. Unlike native ES6 Maps, the
implementations MUST support value, not just referential, equality
semantics (e.g. those provided by
@thi.ng/associative)
or
@thi.ng/cache).
The latter also support automatically pruning of memoization caches,
based on different strategies. See doc strings for further details.
Available memoization functions
Status
STABLE - used in production
Search or submit any issues for this package
Related packages
- @thi.ng/cache - In-memory cache implementations with ES6 Map-like API and different eviction strategies
Installation
yarn add @thi.ng/memoize
ESM import:
import * as mem from "@thi.ng/memoize";
Browser ESM import:
<script type="module" src="https://esm.run/@thi.ng/memoize"></script>
JSDelivr documentation
For Node.js REPL:
const mem = await import("@thi.ng/memoize");
Package sizes (brotli'd, pre-treeshake): ESM: 507 bytes
Dependencies
Note: @thi.ng/api is in most cases a type-only import (not used at runtime)
Usage examples
Three projects in this repo's
/examples
directory are using this package:
Screenshot | Description | Live demo | Source |
---|
| Isolated, component-local DOM updates | Demo | Source |
| Basic rstream-gestures multi-touch demo | Demo | Source |
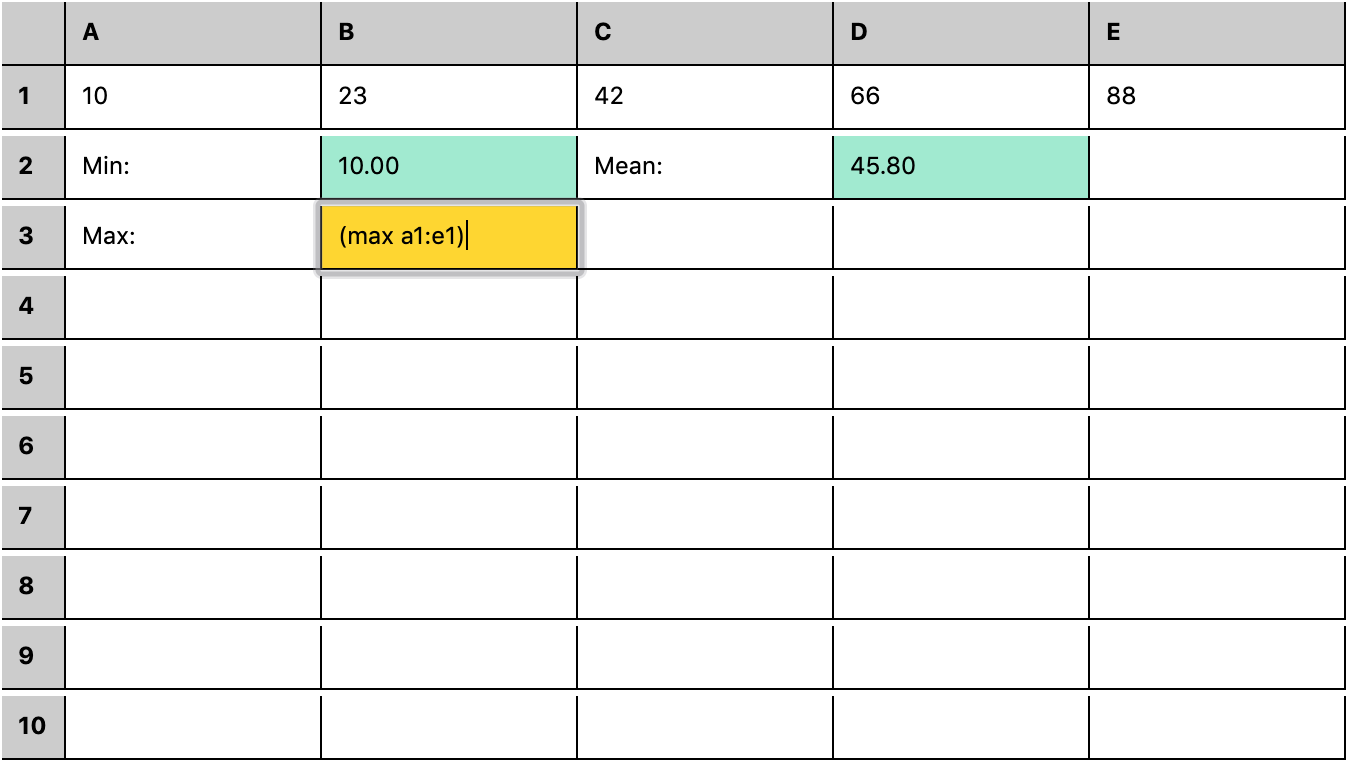 | rstream based spreadsheet w/ S-expression formula DSL | Demo | Source |
API
Generated API docs
import * as m from "@thi.ng/memoize";
import { EquivMap } from "@thi.ng/associative";
import { LRUCache } from "@thi.ng/cache";
Optimized version for single arg functions
import { memoize1 } from "@thi.ng/memoize";
foo = memoize1((x) => (console.log("exec"), x * 10));
foo(1);
foo(1);
import { EquivMap } from "@thi.ng/associative";
foo = memoize1(
(x) => (console.log("exec"), x[0] * 10),
new EquivMap()
);
foo([1]);
foo([1]);
import { LRUCache } from "@thi.ng/cache";
foo = memoize1(
(x) => (console.log("exec"), x[0] * 10),
new LRUCache(null, { maxlen: 3 })
);
Arbitrary args
import { memoize } from "@thi.ng/memoize";
import { EquivMap } from "@thi.ng/associative";
const dotProduct = memoize(
(x, y) => (console.log("exec"), x[0] * y[0] + x[1] * y[1]),
new EquivMap()
);
dotProduct([1,2], [3,4]);
dotProduct([1,2], [3,4]);
Via JSON.stringify()
import { memoizeJ } from "@thi.ng/memoize";
const dotProduct = memoizeJ(
(x, y) => (console.log("exec"), x[0] * y[0] + x[1] * y[1])
);
dotProduct([1, 2], [3, 4]);
dotProduct([1, 2], [3, 4]);
Authors
If this project contributes to an academic publication, please cite it as:
@misc{thing-memoize,
title = "@thi.ng/memoize",
author = "Karsten Schmidt",
note = "https://thi.ng/memoize",
year = 2018
}
License
© 2018 - 2024 Karsten Schmidt // Apache License 2.0