

This project is part of the
@thi.ng/umbrella monorepo.
About
Generic, modular, extensible API bridge, polyglot glue code and bindings code generators for hybrid JS & WebAssembly projects.
This package provides a the following:
- A small, generic and modular
WasmBridge
class as interop basis and much reduced boilerplate for hybrid JS/WebAssembly
applications. - A minimal core API for debug output, string/pointer/typedarray accessors for
8/16/32/64 bit (u)ints and 32/64 bit floats, memory allocation (optional).
Additionally, a number of support modules for DOM
manipulation,
WebGL, WebGPU, WebAudio etc. is being actively worked on.
- Include files for
C11/C++
and
Zig,
defining glue code for the JS core
API defined
by this package
- Extensible shared datatype code generators for (currently) C11, Zig &
TypeScript. The latter also generates fully type checked memory-mapped
(zero-copy) accessors of WASM-side data. In general, all languages with a
WebAssembly target are supported, however currently only bindings for these
mentioned langs are included. Other languages require custom bindings, e.g.
based on the flexible primitives provided here.
- CLI frontend/utility to invoke the code generator(s)
Data bindings & code generators
The package provides an extensible codegeneration framework to simplify the
bilateral design & exchange of data structures shared between the WASM & JS host
env. Currently, code generators for TypeScript, Zig and C11 are supplied. A CLI
wrapper is available too. See the
@thi.ng/wasm-api-dom
support package for a more thorough realworld example...
CLI generator
The package includes a small CLI
wrapper
to invoke the code generator(s) from JSON type definitions and to write the
generated source code(s) to different files:
$ npx @thi.ng/wasm-api
█ █ █ │
██ █ │
█ █ █ █ █ █ █ █ │ @thi.ng/wasm-api 0.15.0
█ █ █ █ █ █ █ █ █ │ Multi-language data bindings code generator
█ │
█ █ │
usage: wasm-api [OPTS] JSON-INPUT-FILE(S) ...
wasm-api --help
Flags:
-d, --debug enable debug output & functions
--dry-run enable dry run (don't overwrite files)
Main:
-a FILE, --analytics FILE output file path for raw codegen analytics
-c FILE, --config FILE JSON config file with codegen options
-l ID[,..], --lang ID[,..] [multiple] target language: "c11", "ts", "zig" (default: ["ts","zig"])
-o FILE, --out FILE [multiple] output file path
-s TYPE, --string TYPE Force string type implementation: "slice", "ptr"
By default, the CLI generates sources for TypeScript and Zig (in this order!).
Order is important, since the output file paths must be given in the same order
as the target languages. It's recommended to be explicit with this. An example
invocation looks like:
wasm-api \
--config codegen-opts.json \
--lang ts -o src/generated.ts \
--lang zig -o src.zig/generated.zig \
typedefs.json
The structure of the config file is as follows (all optional):
{
"global": { ... },
"c11": { ... },
"ts": { ... },
"zig": { ... },
}
More details about possible
global
,
c
and
ts
and
zig
config
options & values.
All code generators have support for custom code prologues & epilogues which can
be specified via the above options. These config options exist for both non-CLI
& CLI usage. For the latter, these custom code sections can also be loaded from
external files by specifying their file paths using @
as prefix, e.g.
{
"ts": { "pre": "@tpl/prelude.ts" },
"zig": { "pre": "@tpl/prelude.zig", "post": "@tpl/epilogue.zig" },
}
Data type definitions
Currently, the code generator supports enums, structs and unions. See API docs for
further details:
Example usage
The following example defines 1x enum, 2x structs and 1x union. Shown here are
the JSON type definitions and the resulting source codes:
⬇︎ CLICK TO EXPAND EACH CODE BLOCK ⬇︎
types.json (Type definitions)
[
{
"name": "EventType",
"type": "enum",
"tag": "u8",
"values": [
"unknown",
{ "name": "mouse", "value": 16 },
{ "name": "key", "value": 32 }
]
},
{
"name": "MouseEvent",
"type": "struct",
"doc": "Example struct",
"fields": [
{ "name": "type", "type": "EventType" },
{ "name": "pos", "type": "u16", "tag": "vec", "len": 2 }
]
},
{
"name": "KeyEvent",
"type": "struct",
"doc": "Example struct",
"fields": [
{ "name": "type", "type": "EventType" },
{ "name": "key", "type": "string" },
{ "name": "modifiers", "type": "u8", "doc": "Bitmask of modifier keys" }
]
},
{
"name": "Event",
"type": "union",
"fields": [
{ "name": "mouse", "type": "MouseEvent" },
{ "name": "key", "type": "KeyEvent" }
]
}
]
generated.ts (generated TypeScript source)
import { Pointer, WasmStringSlice, WasmTypeBase, WasmTypeConstructor } from "@thi.ng/wasm-api";
export enum EventType {
UNKNOWN,
MOUSE = 16,
KEY = 32,
}
export interface MouseEvent extends WasmTypeBase {
type: EventType;
pos: Uint16Array;
}
export const $MouseEvent: WasmTypeConstructor<MouseEvent> = (mem) => ({
get align() {
return 4;
},
get size() {
return 8;
},
instance: (base) => {
return {
get __base() {
return base;
},
get __bytes() {
return mem.u8.subarray(base, base + 8);
},
get type(): EventType {
return mem.u8[base];
},
set type(x: EventType) {
mem.u8[base] = x;
},
get pos(): Uint16Array {
const addr = (base + 4) >>> 1;
return mem.u16.subarray(addr, addr + 2);
},
};
}
});
export interface KeyEvent extends WasmTypeBase {
type: EventType;
key: WasmStringSlice;
modifiers: number;
}
export const $KeyEvent: WasmTypeConstructor<KeyEvent> = (mem) => ({
get align() {
return 4;
},
get size() {
return 16;
},
instance: (base) => {
let $key: WasmStringSlice | null = null;
return {
get __base() {
return base;
},
get __bytes() {
return mem.u8.subarray(base, base + 16);
},
get type(): EventType {
return mem.u8[base];
},
set type(x: EventType) {
mem.u8[base] = x;
},
get key(): WasmStringSlice {
return $key || ($key = new WasmStringSlice(mem, (base + 4), true));
},
get modifiers(): number {
return mem.u8[(base + 12)];
},
set modifiers(x: number) {
mem.u8[(base + 12)] = x;
},
};
}
});
export interface Event extends WasmTypeBase {
mouse: MouseEvent;
key: KeyEvent;
}
export const $Event: WasmTypeConstructor<Event> = (mem) => ({
get align() {
return 4;
},
get size() {
return 16;
},
instance: (base) => {
return {
get __base() {
return base;
},
get __bytes() {
return mem.u8.subarray(base, base + 16);
},
get mouse(): MouseEvent {
return $MouseEvent(mem).instance(base);
},
set mouse(x: MouseEvent) {
mem.u8.set(x.__bytes, base);
},
get key(): KeyEvent {
return $KeyEvent(mem).instance(base);
},
set key(x: KeyEvent) {
mem.u8.set(x.__bytes, base);
},
};
}
});
generated.zig (generated Zig source)
//! Generated by @thi.ng/wasm-api at 2022-10-26T08:36:16.827Z - DO NOT EDIT!
const std = @import("std");
pub const EventType = enum(u8) {
UNKNOWN,
MOUSE = 16,
KEY = 32,
};
/// Example struct
pub const MouseEvent = struct {
type: EventType,
pos: @Vector(2, u16),
};
/// Example struct
pub const KeyEvent = struct {
type: EventType,
key: []const u8,
/// Bitmask of modifier keys
modifiers: u8,
};
pub const Event = union {
mouse: MouseEvent,
key: KeyEvent,
};
On the TypeScript/JS side, the memory-mapped wrappers (e.g. $Event
)
can be used in combination with the WasmBridge
to obtain fully typed views
(according to the generated types) of the underlying WASM memory. Basic usage is
like:
import { WasmBridge } from "@thi.ng/wasm-api";
import { $Event, EventType } from "./generated.ts";
const bridge = new WasmBridge();
const event = $Event(bridge).instance(0x10000);
event.mouse.pos
event.mouse.pos[0] = 300;
event.mouse.type === EventType.MOUSE
IMPORTANT: Field setters are currently only supported for single values,
incl. enums, strings, structs, unions. The latter 2 will always be copied by
value (mem copy). Arrays or slices of strings do not currently provide write
access...
String handling
Most low-level languages deal with strings very differently and alas there's no
general standard. Some have UTF-8/16 support, others don't. In some languages
(incl. C & Zig), strings are stored as zero terminated, in others they aren't...
It's outside the scope of this package to provide an allround out-of-the-box
solution. The WasmBridge
provides read & write accessors to obtain JS strings
from UTF-8 encoded WASM memory. See
getString()
and
setString()
for details.
The code generators too provide a global stringType
option to
interpret the string
type of a struct field in different ways:
slice
(default): Considers strings as Zig-style slices (i.e. pointer + length)ptr
: Considers strings as C-style raw *char
pointer (without any length)
Note: If setting this global option to ptr
, it also has to be repeated for the
TypeScript code generator explicitly.
Memory allocations
If explicitly enabled on the WASM side, the WasmBridge
includes support for
malloc/free-style allocations (within the linear WASM memory) from the JS side
(Note: This is a breaking change in v0.10.0, now using a more flexible approach
& reverse logic of earlier alpha versions).
The actual allocator is implementation specific and suitable generic mechanisms
are defined for both the included Zig & C bindings. Please see for further
reference:
Note: The provided Zig mechanism supports the idiomatic (Zig) pattern of working
with multiple allocators in different parts of the application and supports
dynamic assignments/swapping of the exposed allocator. See comments in source
file and
tests
for more details...
try {
const addr = bridge.allocate(256);
const num = bridge.setString("hello WASM world!", addr, 256, true);
bridge.exports.doSomethingWithString(addr, num);
bridge.free(addr, 256);
} catch(e) {
}
Custom API modules
The
WasmBridge
can be extented via custom defined API modules. Such API extensions will consist
of a collection of JS/TS functions & variables, their related counterparts
(import definitions) for the WASM target and (optionally) some shared data types
(bindings for which can be generated by this package
too).
On the JS side, custom API modules can be easily integrated via the IWasmAPI
interface. The
following example provides a brief overview:
import { IWasmAPI, WasmBridge } from "@thi.ng/wasm-api";
export class CustomAPI implements IWasmAPI {
parent!: WasmBridge;
async init(parent: WasmBridge) {
this.parent = parent;
this.parent.logger.debug("initializing custom API");
return true;
}
getImports(): WebAssembly.Imports {
return {
fillRandom: (addr: number, num: number) => {
addr >>>= 2;
while(num-- > 0) this.parent.f32[addr++] = Math.random();
}
};
}
}
Now we can supply this custom API when creating the main WASM bridge:
export const bridge = new WasmBridge({ custom: new CustomAPI() });
In Zig (or any other language of your choice) we can then utilize this custom
API like so (Please also see
tests
& other examples in this readme):
Bindings file / lib:
//! custom.zig - extern definitions of custom JS API
/// JS external to fill a slice w/ random values
/// Note: Each API module uses a separate import object to avoid naming clashes
/// Here we declare an external binding belonging to the "custom" import group
///
/// The bridge core API uses "wasmapi" as reserved import group name
extern "custom" fn fillRandom(addr: [*]f32, num: usize) void;
Main Zig file:
// Import JS core API
const js = @import("wasmapi");
const custom = @import("custom.zig");
export fn test_randomVec4() void {
var foo = [4]f32{ 1, 2, 3, 4 };
// print original
js.printF32Array(foo[0..]);
// populate foo with random numbers
custom.fillRandom(&foo, foo.len);
// print result
js.printF32Array(foo[0..]);
}
Object indices & handles
Since only numeric values can be exchanged between the WASM module and the JS
host, any JS native objects the WASM side might want to be working with must be
managed manually in JS. For this purpose the ObjectIndex
class can be
used by API modules to handle ID generation (incl. recycling, using
@thi.ng/idgen)
and the indexing of different types of JS objects/values. Only the numeric IDs
(handles) will then need to be exchanged with the WASM module...
import { ObjectIndex } from "@thi.ng/wasm-api";
const canvases = new ObjectIndex<HTMLCanvasElement>({ name: "canvas" });
canvases.add(document.createElement("canvas"));
canvases.get(0);
canvases.get(0).id = "foo";
canvases.has(1)
canvases.get(1)
canvases.get(1, false)
canvases.find((x) => x.id == "bar")
canvases.delete(0);
Since v0.15.0, the supplied Zig core bindings lib also includes a
ManagedIndex
for similar dealings on the Zig side of the application. For example, in the
@thi.ng/wasm-api-dom
module this is used to manage Zig event listeners.
Status
ALPHA - bleeding edge / work-in-progress
Search or submit any issues for this package
Support packages
Installation
yarn add @thi.ng/wasm-api
ES module import:
<script type="module" src="https://cdn.skypack.dev/@thi.ng/wasm-api"></script>
Skypack documentation
For Node.js REPL:
# with flag only for < v16
node --experimental-repl-await
> const wasmApi = await import("@thi.ng/wasm-api");
Package sizes (gzipped, pre-treeshake): ESM: 7.06 KB
IMPORTANT: The package includes code generators for various languages which
are not required for just using the API bridge. Hence, the usual package
size in production will be MUCH smaller than what's stated here!
Dependencies
Usage examples
Several demos in this repo's
/examples
directory are using this package.
A selection:
Screenshot | Description | Live demo | Source |
---|
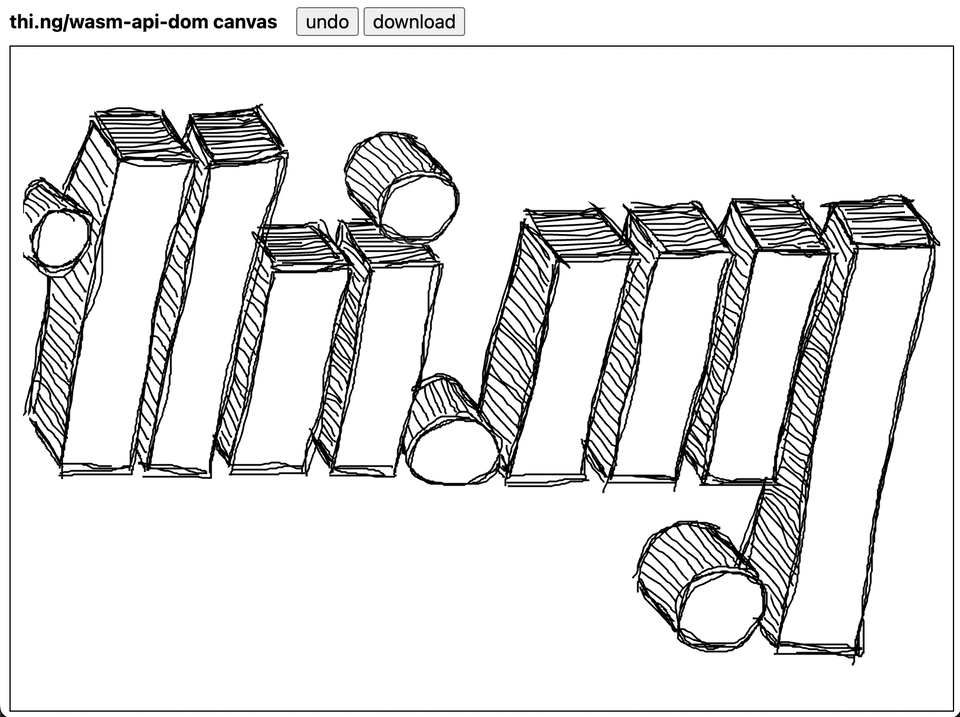 | Zig-based DOM creation & canvas drawing app | Demo | Source |
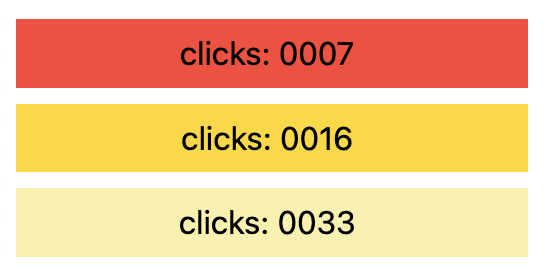 | Simple Zig/WASM click counter DOM component | Demo | Source |
API
Generated API docs
Basic usage example
import { WasmBridge, WasmExports } from "@thi.ng/wasm-api";
import { readFileSync } from "fs";
interface App extends WasmExports {
start: () => void;
}
(async () => {
const bridge = new WasmBridge<App>();
await bridge.instantiate(readFileSync("hello.wasm"));
bridge.exports.start();
})();
Zig version
Requires Zig to be installed:
//! Example Zig application (hello.zig)
/// import externals
/// see build command for configuration
const js = @import("wasmapi");
const std = @import("std");
// set custom memory allocator (here to disable)
pub const WASM_ALLOCATOR: ?std.mem.Allocator = null;
export fn start() void {
js.printStr("hello world!");
}
The WASM binary can be built using the following command (or for more complex
scenarios add the supplied .zig file(s) to your build.zig
and/or source
folder):
zig build-lib \
--pkg-begin wasmapi node_modules/@thi.ng/wasm-api/zig/wasmapi.zig --pkg-end \
-target wasm32-freestanding \
-O ReleaseSmall -dynamic --strip \
hello.zig
wasm-dis -o hello.wast hello.wasm
The resulting WASM:
(module
(type $i32_i32_=>_none (func (param i32 i32)))
(type $none_=>_none (func))
(type $i32_=>_i32 (func (param i32) (result i32)))
(import "wasmapi" "_printStr" (func $fimport$0 (param i32 i32)))
(global $global$0 (mut i32) (i32.const 1048576))
(memory $0 17)
(data (i32.const 1048576) "hello world!\00")
(export "memory" (memory $0))
(export "start" (func $0))
(export "_wasm_allocate" (func $1))
(export "_wasm_free" (func $2))
(func $0
(call $fimport$0
(i32.const 1048576)
(i32.const 12)
)
)
(func $1 (param $0 i32) (result i32)
(i32.const 0)
)
(func $2 (param $0 i32) (param $1 i32)
)
)
C11 version
Requires Emscripten to be installed:
#include <wasmapi.h>
void WASM_KEEP start() {
wasm_printStr0("hello world!");
}
Building the WASM module:
emcc -Os -Inode_modules/@thi.ng/wasm-api/include \
-sERROR_ON_UNDEFINED_SYMBOLS=0 --no-entry \
-o hello.wasm hello.c
Resulting WASM:
(module
(type $i32_=>_none (func (param i32)))
(type $none_=>_none (func))
(type $i32_=>_i32 (func (param i32) (result i32)))
(type $none_=>_i32 (func (result i32)))
(type $i32_i32_=>_none (func (param i32 i32)))
(import "wasmapi" "_printStr0" (func $fimport$0 (param i32)))
(global $global$0 (mut i32) (i32.const 5243936))
(memory $0 256 256)
(data (i32.const 1024) "hello world!")
(table $0 2 2 funcref)
(elem (i32.const 1) $0)
(export "memory" (memory $0))
(export "_wasm_allocate" (func $1))
(export "_wasm_free" (func $2))
(export "start" (func $3))
(export "__indirect_function_table" (table $0))
(export "_initialize" (func $0))
(export "__errno_location" (func $7))
(export "stackSave" (func $4))
(export "stackRestore" (func $5))
(export "stackAlloc" (func $6))
(func $0
(nop)
)
(func $1 (param $0 i32) (result i32)
(i32.const 0)
)
(func $2 (param $0 i32) (param $1 i32)
(nop)
)
(func $3
(call $fimport$0
(i32.const 1024)
)
)
(func $4 (result i32)
(global.get $global$0)
)
(func $5 (param $0 i32)
(global.set $global$0
(local.get $0)
)
)
(func $6 (param $0 i32) (result i32)
(global.set $global$0
(local.tee $0
(i32.and
(i32.sub
(global.get $global$0)
(local.get $0)
)
(i32.const -16)
)
)
)
(local.get $0)
)
(func $7 (result i32)
(i32.const 1040)
)
)
Authors
Karsten Schmidt
If this project contributes to an academic publication, please cite it as:
@misc{thing-wasm-api,
title = "@thi.ng/wasm-api",
author = "Karsten Schmidt",
note = "https://thi.ng/wasm-api",
year = 2022
}
License
© 2022 Karsten Schmidt // Apache Software License 2.0