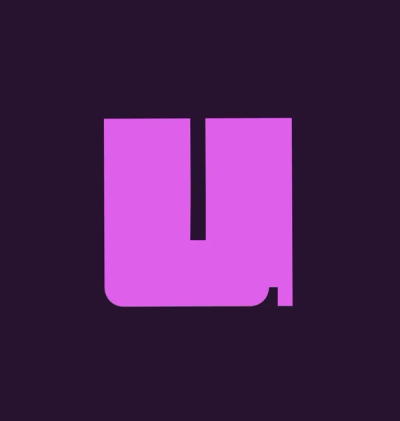
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
@tonconnect/ui
Advanced tools
⚠️ **API is work in progress right now. Don't use this package in production.**
⚠️ API is work in progress right now. Don't use this package in production.
TonConnect UI is a UI kit for TonConnect SDK. Use it to connect your app to TON wallets via TonConnect protocol.
If you use React for your dapp, take a look at TonConnect UI React kit.
If you want to use TonConnect on the server side, you should use the TonConnect SDK.
You can find more details and the protocol specification in the docs.
npm i @tonconnect/ui
import TonConnectUI from '@tonconnect/ui'
const tonConnectUI = new TonConnectUI({
manifestUrl: 'https://<YOUR_APP_URL>/tonconnect-manifest.json',
buttonRootId: '<YOUR_CONNECT_BUTTON_ANCHOR_ID>'
});
See all available options:
TonConnectUiOptionsWithManifest
TonConnectUiOptionsWithConnector
tonConnectUI.uiOptions = {
language: 'ru',
theme: THEME.DARK
};
UI element will be rerendered after assignation. You should pass only options that you want to change.
Passed options will be merged with current UI options. Note, that you have to pass object to tonConnectUI.uiOptions
to keep reactivity.
DON'T do this:
tonConnectUI.uiOptions.language = 'ru'; // WRONG, WILL NOT WORK
const walletsList = await tonConnectUI.getWallets();
/* walletsList is
{
name: string;
imageUrl: string;
tondns?: string;
aboutUrl: string;
universalLink?: string;
deepLink?: string;
bridgeUrl?: string;
jsBridgeKey?: string;
injected?: boolean; // true if this wallet is injected to the webpage
embedded?: boolean; // true if the dapp is opened inside this wallet's browser
}[]
*/
or
const walletsList = await TonConnectUI.getWallets();
"TonConnect UI connect button" (which is added at buttonRootId
) automatically handles clicks and calls connect.
But you are still able to open "connect modal" programmatically, e.g. after click on your custom connect button.
const connectedWallet = await tonConnectUI.connectWallet();
If there is an error while wallet connecting, TonConnectUIError
or TonConnectError
will be thrown depends on situation.
You can use special getters to read current connection state. Note that this getter only represents current value, so they are not reactive.
To react and handle wallet changes use onStatusChange
mathod.
const currentWallet = tonConnectUI.wallet;
const currentWalletInfo = tonConnectUI.walletInfo;
const currentAccount = tonConnectUI.account;
const currentIsConnectedStatus = tonConnectUI.connected;
const unsubscribe = tonConnectUI.onStatusChange(
walletAndwalletInfo => {
// update state/reactive variables to show updates in the ui
}
);
// call `unsubscribe()` later to save resources when you don't need to listen for updates anymore.
Call to disconnect the wallet.
await tonConnectUI.disconnect();
Wallet must be connected when you call sendTransaction
. Otherwise, an error will be thrown.
const transaction = {
validUntil: Date.now() + 1000000,
messages: [
{
address: "0:412410771DA82CBA306A55FA9E0D43C9D245E38133CB58F1457DFB8D5CD8892F",
amount: "20000000",
stateInit: "base64bocblahblahblah==" // just for instance. Replace with your transaction initState or remove
},
{
address: "0:E69F10CC84877ABF539F83F879291E5CA169451BA7BCE91A37A5CED3AB8080D3",
amount: "60000000",
payload: "base64bocblahblahblah==" // just for instance. Replace with your transaction payload or remove
}
]
}
try {
const result = await tonConnectUI.sendTransaction(transaction);
// you can use signed boc to find the transaction
const someTxData = await myAppExplorerService.getTransaction(result.boc);
alert('Transaction was sent successfully', someTxData);
} catch (e) {
console.error(e);
}
sendTransaction
will automatically render informational modals and notifications. You can change its behaviour:
const result = await tonConnectUI.sendTransaction(defaultTx, {
modals: ['before', 'success', 'error'],
notifications: ['before', 'success', 'error']
});
Default configuration is:
const defaultBehaviour = {
modals: ['before'],
notifications: ['before', 'success', 'error']
}
FAQs
TonConnect UI is a UI kit for TonConnect SDK. Use it to connect your app to TON wallets via TonConnect protocol.
The npm package @tonconnect/ui receives a total of 16,484 weekly downloads. As such, @tonconnect/ui popularity was classified as popular.
We found that @tonconnect/ui demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.