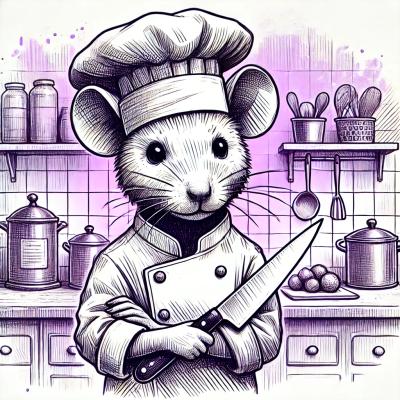
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@turf/clusters
Advanced tools
@turf/clusters is a module within the Turf.js library that provides functionality for clustering geographic points. It allows you to group points based on their proximity to each other, which is useful for various geospatial analyses such as identifying hotspots, aggregating data, and simplifying visualizations.
Cluster K-Means
This feature allows you to perform K-Means clustering on a set of points. The code sample demonstrates how to cluster a set of points into 2 clusters using the K-Means algorithm.
const turf = require('@turf/turf');
const points = turf.featureCollection([
turf.point([0, 0]),
turf.point([2, 2]),
turf.point([3, 3]),
turf.point([8, 8]),
turf.point([8, 9]),
turf.point([7, 7])
]);
const clustered = turf.clustersKmeans(points, {numberOfClusters: 2});
console.log(clustered);
Cluster DBSCAN
This feature allows you to perform DBSCAN clustering on a set of points. The code sample demonstrates how to cluster a set of points using the DBSCAN algorithm with a maximum distance of 5 units and a minimum of 2 points per cluster.
const turf = require('@turf/turf');
const points = turf.featureCollection([
turf.point([0, 0]),
turf.point([2, 2]),
turf.point([3, 3]),
turf.point([8, 8]),
turf.point([8, 9]),
turf.point([7, 7])
]);
const clustered = turf.clustersDbscan(points, 5, 2);
console.log(clustered);
Cluster Hierarchical
This feature allows you to perform hierarchical clustering on a set of points. The code sample demonstrates how to cluster a set of points using hierarchical clustering with a minimum of 2 points per cluster and a maximum distance of 5 units.
const turf = require('@turf/turf');
const points = turf.featureCollection([
turf.point([0, 0]),
turf.point([2, 2]),
turf.point([3, 3]),
turf.point([8, 8]),
turf.point([8, 9]),
turf.point([7, 7])
]);
const clustered = turf.clustersHierarchical(points, {minPoints: 2, maxDistance: 5});
console.log(clustered);
Supercluster is a fast geospatial point clustering library for browsers and Node. It is designed for visualizing large datasets on maps by clustering points into groups. Compared to @turf/clusters, Supercluster is optimized for performance and is particularly useful for rendering clusters on interactive maps.
kmeans-js is a simple implementation of the K-Means clustering algorithm in JavaScript. It is not specifically designed for geospatial data but can be used for general clustering tasks. Compared to @turf/clusters, kmeans-js is more general-purpose and lacks the geospatial-specific optimizations and features.
hdbscan is a high-performance implementation of the HDBSCAN clustering algorithm. It is designed for hierarchical density-based clustering and is suitable for large datasets. Compared to @turf/clusters, hdbscan offers more advanced clustering capabilities but requires more computational resources and is more complex to use.
Get Cluster
geojson
FeatureCollection GeoJSON Featuresfilter
any Filter used on GeoJSON properties to get Clustervar geojson = turf.featureCollection([
turf.point([0, 0], {'marker-symbol': 'circle'}),
turf.point([2, 4], {'marker-symbol': 'star'}),
turf.point([3, 6], {'marker-symbol': 'star'}),
turf.point([5, 1], {'marker-symbol': 'square'}),
turf.point([4, 2], {'marker-symbol': 'circle'})
]);
// Create a cluster using K-Means (adds `cluster` to GeoJSON properties)
var clustered = turf.clustersKmeans(geojson);
// Retrieve first cluster (0)
var cluster = turf.getCluster(clustered, {cluster: 0});
//= cluster
// Retrieve cluster based on custom properties
turf.getCluster(clustered, {'marker-symbol': 'circle'}).length;
//= 2
turf.getCluster(clustered, {'marker-symbol': 'square'}).length;
//= 1
Returns FeatureCollection Single Cluster filtered by GeoJSON Properties
Callback for clusterEach
Type: Function
cluster
FeatureCollection? The current cluster being processed.clusterValue
any? Value used to create cluster being processed.currentIndex
number? The index of the current element being processed in the array.Starts at index 0Returns void
clusterEach
geojson
FeatureCollection GeoJSON Featuresproperty
(string | number) GeoJSON property key/value used to create clusterscallback
Function a method that takes (cluster, clusterValue, currentIndex)var geojson = turf.featureCollection([
turf.point([0, 0]),
turf.point([2, 4]),
turf.point([3, 6]),
turf.point([5, 1]),
turf.point([4, 2])
]);
// Create a cluster using K-Means (adds `cluster` to GeoJSON properties)
var clustered = turf.clustersKmeans(geojson);
// Iterate over each cluster
turf.clusterEach(clustered, 'cluster', function (cluster, clusterValue, currentIndex) {
//= cluster
//= clusterValue
//= currentIndex
})
// Calculate the total number of clusters
var total = 0
turf.clusterEach(clustered, 'cluster', function () {
total++;
});
// Create an Array of all the values retrieved from the 'cluster' property
var values = []
turf.clusterEach(clustered, 'cluster', function (cluster, clusterValue) {
values.push(clusterValue);
});
Returns void
Callback for clusterReduce
The first time the callback function is called, the values provided as arguments depend on whether the reduce method has an initialValue argument.
If an initialValue is provided to the reduce method:
If an initialValue is not provided:
Type: Function
previousValue
any? The accumulated value previously returned in the last invocation
of the callback, or initialValue, if supplied.cluster
FeatureCollection? The current cluster being processed.clusterValue
any? Value used to create cluster being processed.currentIndex
number? The index of the current element being processed in the
array. Starts at index 0, if an initialValue is provided, and at index 1 otherwise.Reduce clusters in GeoJSON Features, similar to Array.reduce()
geojson
FeatureCollection GeoJSON Featuresproperty
(string | number) GeoJSON property key/value used to create clusterscallback
Function a method that takes (previousValue, cluster, clusterValue, currentIndex)initialValue
any? Value to use as the first argument to the first call of the callback.var geojson = turf.featureCollection([
turf.point([0, 0]),
turf.point([2, 4]),
turf.point([3, 6]),
turf.point([5, 1]),
turf.point([4, 2])
]);
// Create a cluster using K-Means (adds `cluster` to GeoJSON properties)
var clustered = turf.clustersKmeans(geojson);
// Iterate over each cluster and perform a calculation
var initialValue = 0
turf.clusterReduce(clustered, 'cluster', function (previousValue, cluster, clusterValue, currentIndex) {
//=previousValue
//=cluster
//=clusterValue
//=currentIndex
return previousValue++;
}, initialValue);
// Calculate the total number of clusters
var total = turf.clusterReduce(clustered, 'cluster', function (previousValue) {
return previousValue++;
}, 0);
// Create an Array of all the values retrieved from the 'cluster' property
var values = turf.clusterReduce(clustered, 'cluster', function (previousValue, cluster, clusterValue) {
return previousValue.concat(clusterValue);
}, []);
Returns any The value that results from the reduction.
This module is part of the Turfjs project, an open source module collection dedicated to geographic algorithms. It is maintained in the Turfjs/turf repository, where you can create PRs and issues.
Install this single module individually:
$ npm install @turf/clusters
Or install the all-encompassing @turf/turf module that includes all modules as functions:
$ npm install @turf/turf
FAQs
turf clusters module
The npm package @turf/clusters receives a total of 508,285 weekly downloads. As such, @turf/clusters popularity was classified as popular.
We found that @turf/clusters demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.