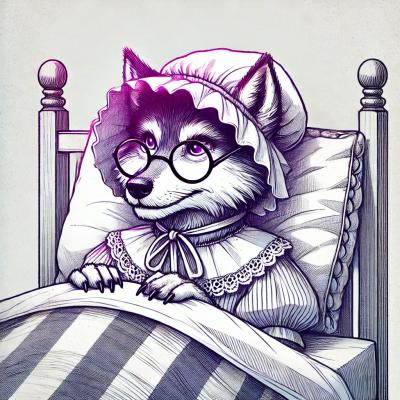
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@turf/transform-scale
Advanced tools
@turf/transform-scale is a module from the Turf.js library that allows you to scale GeoJSON geometries. This can be useful for tasks such as resizing polygons, lines, and points by a given factor, either from their centroid or a specified origin.
Scale a Polygon
This feature allows you to scale a polygon by a factor of 2. The polygon is scaled from its centroid by default.
const turf = require('@turf/turf');
const polygon = turf.polygon([[
[-70.603637, -33.399918],
[-70.614624, -33.395332],
[-70.639343, -33.392466],
[-70.659942, -33.394759],
[-70.683975, -33.404504],
[-70.697021, -33.419406],
[-70.701141, -33.434306],
[-70.700454, -33.446339],
[-70.694274, -33.458369],
[-70.682601, -33.465816],
[-70.668869, -33.472117],
[-70.646209, -33.473835],
[-70.624923, -33.472117],
[-70.609817, -33.468107],
[-70.595397, -33.458369],
[-70.587158, -33.442901],
[-70.587158, -33.426283],
[-70.590591, -33.414248],
[-70.594711, -33.406224],
[-70.603637, -33.399918]
]]);
const scaledPolygon = turf.transformScale(polygon, 2);
console.log(JSON.stringify(scaledPolygon));
Scale a LineString
This feature allows you to scale a LineString by a factor of 1.5. The line is scaled from its centroid by default.
const turf = require('@turf/turf');
const line = turf.lineString([
[-70.603637, -33.399918],
[-70.614624, -33.395332],
[-70.639343, -33.392466],
[-70.659942, -33.394759],
[-70.683975, -33.404504]
]);
const scaledLine = turf.transformScale(line, 1.5);
console.log(JSON.stringify(scaledLine));
Scale a Point
This feature allows you to scale a point by a factor of 3. The point is scaled from its centroid by default, although for points, the scaling operation is trivial.
const turf = require('@turf/turf');
const point = turf.point([-70.603637, -33.399918]);
const scaledPoint = turf.transformScale(point, 3);
console.log(JSON.stringify(scaledPoint));
JSTS is a JavaScript library of spatial predicates and functions for processing geometry. It provides a wide range of geometric operations, including scaling, but is more complex and feature-rich compared to @turf/transform-scale.
Geolib is a library to provide basic geospatial operations like distance calculation, conversion of units, and bounding boxes. While it does not focus on scaling geometries, it offers a variety of other geospatial utilities.
Martinez Polygon Clipping is a library for performing boolean operations on polygons. It can handle complex polygon operations but does not specifically focus on scaling geometries like @turf/transform-scale.
Scale GeoJSON objects from a given point by a scaling factor e.g. factor=2 would make each object 200% larger. If a FeatureCollection is provided, the origin point will be calculated based on each individual feature unless an exact
geojson
(GeoJSON | GeometryCollection) objects to be scaled
factor
number of scaling, positive values greater than 0. Numbers between 0 and 1 will shrink the geojson, numbers greater than 1 will expand it, a factor of 1 will not change the geojson.
options
Object Optional parameters (optional, default {}
)
const poly = turf.polygon([[[0,29],[3.5,29],[2.5,32],[0,29]]]);
const scaledPoly = turf.transformScale(poly, 3);
//addToMap
const addToMap = [poly, scaledPoly];
scaledPoly.properties = {stroke: '#F00', 'stroke-width': 4};
Returns (GeoJSON | GeometryCollection) scaled GeoJSON
This module is part of the Turfjs project, an open source module collection dedicated to geographic algorithms. It is maintained in the Turfjs/turf repository, where you can create PRs and issues.
Install this single module individually:
$ npm install @turf/transform-scale
Or install the all-encompassing @turf/turf module that includes all modules as functions:
$ npm install @turf/turf
FAQs
turf transform-scale module
The npm package @turf/transform-scale receives a total of 263,041 weekly downloads. As such, @turf/transform-scale popularity was classified as popular.
We found that @turf/transform-scale demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.