What is @tweenjs/tween.js?
@tweenjs/tween.js is a simple but powerful tweening engine for JavaScript. It allows you to create smooth animations by interpolating between values over time. This can be used for animating properties of objects, such as position, scale, rotation, and more.
What are @tweenjs/tween.js's main functionalities?
Basic Tweening
This code demonstrates basic tweening where an object's properties (x and y coordinates) are animated from one state to another over a duration of 1000 milliseconds.
const TWEEN = require('@tweenjs/tween.js');
let coords = { x: 0, y: 0 };
let tween = new TWEEN.Tween(coords)
.to({ x: 100, y: 100 }, 1000)
.onUpdate(() => {
console.log(coords);
})
.start();
function animate(time) {
requestAnimationFrame(animate);
TWEEN.update(time);
}
requestAnimationFrame(animate);
Easing Functions
This code demonstrates the use of easing functions to create more natural motion. The Quadratic.Out easing function is used to slow down the animation towards the end.
const TWEEN = require('@tweenjs/tween.js');
let coords = { x: 0, y: 0 };
let tween = new TWEEN.Tween(coords)
.to({ x: 100, y: 100 }, 1000)
.easing(TWEEN.Easing.Quadratic.Out)
.onUpdate(() => {
console.log(coords);
})
.start();
function animate(time) {
requestAnimationFrame(animate);
TWEEN.update(time);
}
requestAnimationFrame(animate);
Chaining Tweens
This code demonstrates chaining tweens, where one tween starts after another finishes. The x coordinate is animated first, followed by the y coordinate.
const TWEEN = require('@tweenjs/tween.js');
let coords = { x: 0, y: 0 };
let tween1 = new TWEEN.Tween(coords)
.to({ x: 100 }, 1000);
let tween2 = new TWEEN.Tween(coords)
.to({ y: 100 }, 1000);
tween1.chain(tween2);
tween1.start();
function animate(time) {
requestAnimationFrame(animate);
TWEEN.update(time);
}
requestAnimationFrame(animate);
Other packages similar to @tweenjs/tween.js
gsap
GSAP (GreenSock Animation Platform) is a robust and high-performance JavaScript animation library. It offers more features and flexibility compared to @tweenjs/tween.js, including timeline management, advanced easing, and support for SVG animations.
animejs
Anime.js is a lightweight JavaScript animation library with a simple API. It supports various types of animations, including CSS properties, SVG, DOM attributes, and JavaScript objects. It is more feature-rich and versatile compared to @tweenjs/tween.js.
popmotion
Popmotion is a functional, flexible JavaScript animation library. It provides a range of tools for creating animations and interactions, including physics-based animations. It offers more advanced features and flexibility compared to @tweenjs/tween.js.
tween.js
JavaScript tweening engine for easy animations, incorporating optimised Robert Penner's equations.

Update Note In v18 the script you should include has moved from src/Tween.js
to dist/tween.umd.js
. See the installation section below.
const box = document.createElement('div');
box.style.setProperty('background-color', '#008800');
box.style.setProperty('width', '100px');
box.style.setProperty('height', '100px');
document.body.appendChild(box);
function animate(time) {
requestAnimationFrame(animate);
TWEEN.update(time);
}
requestAnimationFrame(animate);
const coords = { x: 0, y: 0 };
const tween = new TWEEN.Tween(coords)
.to({ x: 300, y: 200 }, 1000)
.easing(TWEEN.Easing.Quadratic.Out)
.onUpdate(() => {
box.style.setProperty('transform', `translate(${coords.x}px, ${coords.y}px)`);
})
.start();
Test it with CodePen
Installation
Currently npm is required to build the project.
git clone https://github.com/tweenjs/tween.js
cd tween.js
npm i .
npm run build
This will create some builds in the dist
directory. There are currently four different builds of the library:
- UMD : tween.umd.js
- AMD : tween.amd.js
- CommonJS : tween.cjs.js
- ES6 Module : tween.es.js
You are now able to copy tween.umd.js into your project, then include it with
a script tag. This will add TWEEN to the global scope.
<script src="js/tween.umd.js"></script>
With require('@tweenjs/tween.js')
You can add tween.js as an npm dependency:
npm i @tweenjs/tween.js@^18
If you are using Node, Webpack, or Browserify, then you can now use the following to include tween.js:
const TWEEN = require('@tweenjs/tween.js');
Features
- Does one thing and one thing only: tween properties
- Doesn't take care of CSS units (e.g. appending
px
) - Doesn't interpolate colours
- Easing functions are reusable outside of Tween
- Can also use custom easing functions
Documentation
Examples
Tests
You need to install npm
first--this comes with node.js, so install that one first. Then, cd to tween.js
's directory and run:
npm install
if running the tests for the first time, to install additional dependencies for running tests, and then run
npm test
every time you want to run the tests.
If you want to add any feature or change existing features, you must run the tests to make sure you didn't break anything else. If you send a pull request (PR) to add something new and it doesn't have tests, or the tests don't pass, the PR won't be accepted. See contributing for more information.
People
Maintainers: mikebolt, sole.
All contributors.
Projects using tween.js
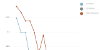