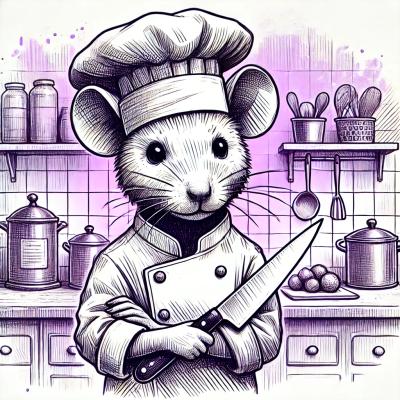
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@twreporter/errors
Advanced tools
[](https://www.npmjs.com/package/@twreporter/errors)
This package provides the helpers for handling errors in the The Reporter website.
Inspired by package errors
for Go language and the related article by Dave Cheney.
Reference:
Annotate the error with name
, message
and payload
. It will return a new error that is an instance of errors.AnnotatingError
.
See source code and JSDoc for all parameters
Example:
import errors from '@twreporter/errors'
function doSomething() {
try {
const someArguments = {
/* ... */
}
invokeSomethingWithError(...someArguments)
} catch (error) {
console.error(
errors.helpers.wrap(
error,
'ErrorType',
'failed to invoke something',
{ args: someArguments },
invokeSomethingWithError
)
)
}
}
It will return the previous error in the chain that the input error belongs to.
If there is no pointer of previous error saved in the input error, it will return null
.
It works like the Unwrap
method of errors in Go@^1.13.
See source code and JSDoc for all parameters
Example:
import errors from '@twreporter/errors'
function doSomething() {
try {
const someArguments = {
/* ... */
}
invokeSomethingWithError(...someArguments)
} catch (error) {
/* list all error.name in errors chain */
let _error = error
const chain = []
while (_error) {
chain.push(_error)
_error = errors.helpers.unwrap(_error)
}
/* ... handle the chain */
}
}
It will return the earliest error in the chain that the input error belongs to.
See source code and JSDoc for all parameters
import errors from '@twreporter/errors'
function nestedFailedTask() {
throw new Error('nested failure')
}
function invokeSomething() {
const someArguments = { bar: 'bar' }
try {
nestedFailedTask()
} catch (error) {
throw errors.helpers.wrap(
error,
'ErrorType',
'failed to invoke nested task',
{ args: someArguments }
)
}
}
function doSomething() {
const someArguments = { foo: 'foo' }
try {
invokeSomething(someArguments)
} catch (error) {
const annotatingError = errors.helpers.wrap(
error,
'ErrorType',
'failed to invoke something',
{ args: someArguments }
)
errors.helpers.cause(annotatingError) === 'nested failure' /* true */
}
}
log out:
ErrorType: failed to invoke something
at doSomething (/test.js:12:44)
payload {"args":{"foo":"foo"}}
See source code and JSDoc for all parameters
import errors from '@twreporter/errors'
function nestedFailedTask() {
throw new Error('nested failure')
}
function invokeSomething() {
const someArguments = { bar: 'bar' }
try {
nestedFailedTask()
} catch (error) {
throw errors.helpers.wrap(
error,
'ErrorType',
'failed to invoke nested task',
{ args: someArguments }
)
}
}
function doSomething() {
const someArguments = { foo: 'foo' }
try {
invokeSomething(someArguments)
} catch (error) {
const annotatingError = errors.helpers.wrap(
error,
'ErrorType',
'failed to invoke something',
{ args: someArguments }
)
const message = errors.helpers.printAll(annotatingError, {
withStack: true,
withPayload: true,
})
console.error(message)
}
}
doSomething()
log out:
Error: nested failure
at nestedFailedTask (/test.js:4:9)
ErrorType: failed to invoke nested task
at invokeSomething (/test.js:12:26)
> payload {"args":{"bar":"bar"}}
ErrorType: failed to invoke something
at doSomething (/test.js:21:44)
> payload {"args":{"foo":"foo"}}
See source code and JSDoc for all parameters
import errors from '@twreporter/errors'
function invokeSomethingWithError() {
throw new Error('nested error')
}
function doSomething() {
const someArguments = { foo: 'foo' }
try {
invokeSomethingWithError(someArguments)
} catch (error) {
const annotatingError = errors.helpers.wrap(
error,
'ErrorType',
'failed to invoke something',
{ args: someArguments }
)
const message = errors.helpers.printOne(annotatingError, {
withStack: true,
withPayload: true,
})
console.error(message)
}
}
doSomething()
log out:
ErrorType: failed to invoke something
at doSomething (/test.js:12:44)
> payload {"args":{"foo":"foo"}}
See source code and JSDoc for all parameters
import errors from '@twreporter/errors'
import axios from 'axios'
async function doSomething() {
try {
const someArguments = {
/* ... */
}
await axios.get(someArguments)
} catch (axiosError) {
throw errors.helpers.annotateAxiosError(axiosError)
}
}
name
{string} the name of this errormessage
{string} the message of this errorpayload
{Object} the context information for better debuggingstack
{string} the stack trace from where the error be constructed. it contains the name and message of the error in the V8 engineSee source code and JSDoc for all parameters
make dev
make build
make publish
FAQs
[](https://www.npmjs.com/package/@twreporter/errors)
The npm package @twreporter/errors receives a total of 36 weekly downloads. As such, @twreporter/errors popularity was classified as not popular.
We found that @twreporter/errors demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.