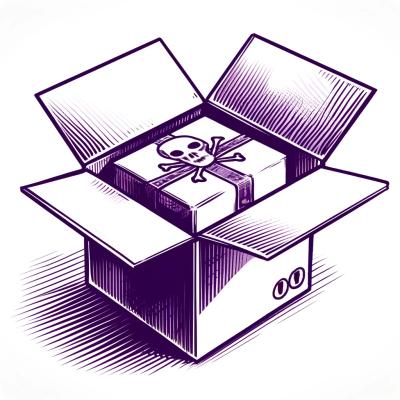
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@types/adm-zip
Advanced tools
TypeScript definitions for adm-zip
@types/adm-zip provides TypeScript type definitions for the adm-zip library, which is used for handling ZIP archives. This package allows developers to work with ZIP files in a type-safe manner, ensuring better code quality and development experience.
Creating a ZIP Archive
This feature allows you to create a new ZIP archive and add files to it. The code sample demonstrates how to add a local file to a ZIP archive and then write the archive to disk.
const AdmZip = require('adm-zip');
const zip = new AdmZip();
zip.addLocalFile('file.txt');
zip.writeZip('archive.zip');
Extracting a ZIP Archive
This feature allows you to extract all files from a ZIP archive to a specified directory. The code sample shows how to extract the contents of 'archive.zip' to the 'output_folder' directory.
const AdmZip = require('adm-zip');
const zip = new AdmZip('archive.zip');
zip.extractAllTo('output_folder', true);
Reading ZIP Archive Contents
This feature allows you to read the contents of a ZIP archive. The code sample demonstrates how to list all the entries (files and directories) in 'archive.zip'.
const AdmZip = require('adm-zip');
const zip = new AdmZip('archive.zip');
const zipEntries = zip.getEntries();
zipEntries.forEach((entry) => {
console.log(entry.entryName);
});
Adding Files and Folders to ZIP Archive
This feature allows you to add entire folders to a ZIP archive. The code sample shows how to add a folder located at 'folder_path' to a new ZIP archive and then write it to disk.
const AdmZip = require('adm-zip');
const zip = new AdmZip();
zip.addLocalFolder('folder_path');
zip.writeZip('archive.zip');
yazl is a ZIP file creation library with a focus on performance and streaming. Unlike adm-zip, yazl is designed to work with streams, making it more suitable for scenarios where memory usage is a concern.
jszip is a popular library for creating, reading, and editing ZIP files. It is well-documented and widely used in the JavaScript community. Compared to adm-zip, jszip offers a more modern API and better support for asynchronous operations.
node-stream-zip is a library for working with ZIP archives using streams. It is optimized for performance and low memory usage, making it a good alternative to adm-zip for handling large ZIP files.
npm install --save @types/adm-zip
This package contains type definitions for adm-zip (https://github.com/cthackers/adm-zip).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/adm-zip.
These definitions were written by John Vilk, Abner Oliveira, BendingBender, and Lei Nelissen.
FAQs
TypeScript definitions for adm-zip
The npm package @types/adm-zip receives a total of 527,822 weekly downloads. As such, @types/adm-zip popularity was classified as popular.
We found that @types/adm-zip demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.