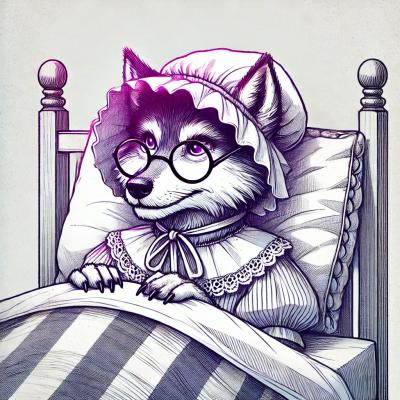
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@types/aws-sdk
Advanced tools
@types/aws-sdk provides TypeScript definitions for the AWS SDK for JavaScript, enabling developers to use AWS services with type safety and autocompletion in TypeScript projects.
S3 Operations
This code demonstrates how to use the AWS SDK to interact with Amazon S3. It retrieves an object from an S3 bucket.
const AWS = require('aws-sdk');
const s3 = new AWS.S3();
const params = {
Bucket: 'example-bucket',
Key: 'example-object'
};
s3.getObject(params, (err, data) => {
if (err) console.log(err, err.stack);
else console.log(data);
});
DynamoDB Operations
This code demonstrates how to use the AWS SDK to interact with Amazon DynamoDB. It retrieves an item from a DynamoDB table.
const AWS = require('aws-sdk');
const dynamoDB = new AWS.DynamoDB.DocumentClient();
const params = {
TableName: 'example-table',
Key: {
'example-key': 'example-value'
}
};
dynamoDB.get(params, (err, data) => {
if (err) console.log(err, err.stack);
else console.log(data);
});
Lambda Function Invocation
This code demonstrates how to use the AWS SDK to invoke an AWS Lambda function. It sends a payload to the specified Lambda function.
const AWS = require('aws-sdk');
const lambda = new AWS.Lambda();
const params = {
FunctionName: 'example-function',
Payload: JSON.stringify({ key: 'value' })
};
lambda.invoke(params, (err, data) => {
if (err) console.log(err, err.stack);
else console.log(data);
});
The aws-sdk package is the official AWS SDK for JavaScript. It provides a comprehensive set of tools for interacting with AWS services. Unlike @types/aws-sdk, it includes the actual implementation of the SDK rather than just TypeScript definitions.
AWS Amplify is a JavaScript library for frontend and mobile developers building cloud-enabled applications. It provides a higher-level abstraction over AWS services, making it easier to integrate with services like authentication, storage, and APIs. It is more opinionated and easier to use for specific use cases compared to the more general-purpose aws-sdk.
The Serverless Framework is a toolkit for deploying and operating serverless architectures. It abstracts away much of the complexity of working directly with AWS services, providing a simpler way to deploy and manage serverless applications. It is more focused on deployment and infrastructure management compared to the aws-sdk.
npm install --save @types/aws-sdk
This package contains type definitions for aws-sdk (https://github.com/aws/aws-sdk-js).
Files were exported from https://www.github.com/DefinitelyTyped/DefinitelyTyped/tree/types-2.0/aws-sdk
Additional Details
These definitions were written by midknight41 https://github.com/midknight41.
FAQs
Stub TypeScript definitions entry for aws-sdk, which provides its own types definitions
The npm package @types/aws-sdk receives a total of 146,954 weekly downloads. As such, @types/aws-sdk popularity was classified as popular.
We found that @types/aws-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.