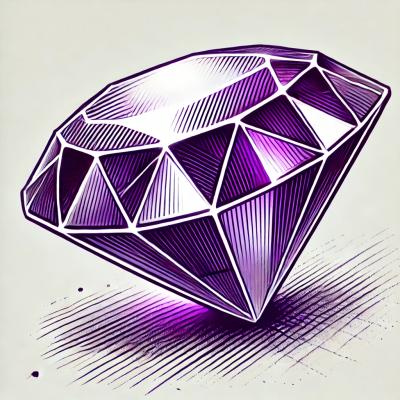
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@types/better-sqlite3
Advanced tools
TypeScript definitions for better-sqlite3
@types/better-sqlite3 provides TypeScript type definitions for the better-sqlite3 package, which is a fast and simple SQLite3 library for Node.js applications. It allows for synchronous database operations and provides a straightforward API for executing SQL queries, managing transactions, and handling prepared statements.
Executing SQL Queries
This feature allows you to execute SQL queries synchronously. The code sample demonstrates how to prepare and execute a SELECT query to fetch a user with a specific ID.
const Database = require('better-sqlite3');
const db = new Database('my-database.db');
const row = db.prepare('SELECT * FROM users WHERE id = ?').get(1);
console.log(row);
Managing Transactions
This feature allows you to manage transactions, ensuring that a series of database operations are executed atomically. The code sample demonstrates how to create and execute a transaction that inserts multiple users into the database.
const Database = require('better-sqlite3');
const db = new Database('my-database.db');
const insert = db.prepare('INSERT INTO users (name, age) VALUES (?, ?)');
const transaction = db.transaction((users) => {
for (const user of users) insert.run(user.name, user.age);
});
transaction([{ name: 'Alice', age: 30 }, { name: 'Bob', age: 25 }]);
Handling Prepared Statements
This feature allows you to handle prepared statements for executing SQL queries multiple times with different parameters. The code sample demonstrates how to prepare an INSERT statement and execute it with different values.
const Database = require('better-sqlite3');
const db = new Database('my-database.db');
const stmt = db.prepare('INSERT INTO users (name, age) VALUES (?, ?)');
stmt.run('Charlie', 35);
stmt.run('Dave', 40);
The sqlite3 package is another SQLite library for Node.js that provides both asynchronous and synchronous APIs. Unlike better-sqlite3, which is synchronous by default, sqlite3 is primarily asynchronous, making it more suitable for applications that require non-blocking database operations.
node-sqlite3 is a binding to the SQLite3 C library and provides a similar API to better-sqlite3. It supports both asynchronous and synchronous operations, but its API is more complex and less intuitive compared to better-sqlite3.
sql.js is a JavaScript library that runs SQLite in the browser using Emscripten. It is not a direct competitor to better-sqlite3, as it is designed for client-side applications, but it provides similar SQLite functionalities in a different environment.
npm install --save @types/better-sqlite3
This package contains type definitions for better-sqlite3 (https://github.com/JoshuaWise/better-sqlite3).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/better-sqlite3.
These definitions were written by Ben Davies, Mathew Rumsey, Santiago Aguilar, Alessandro Vergani, Andrew Kaiser, Mark Stewart, Florian Stamer, and Beeno Tung.
FAQs
TypeScript definitions for better-sqlite3
The npm package @types/better-sqlite3 receives a total of 133,642 weekly downloads. As such, @types/better-sqlite3 popularity was classified as popular.
We found that @types/better-sqlite3 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.