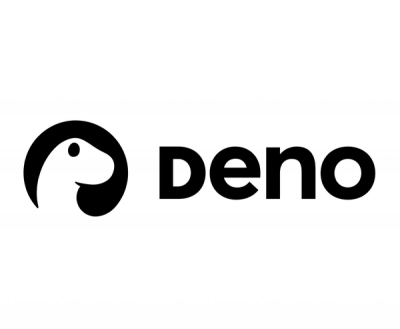
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@types/bunyan
Advanced tools
@types/bunyan provides TypeScript type definitions for the Bunyan logging library, which is a simple and fast JSON logging library for Node.js services.
Basic Logging
This feature allows you to create a basic logger and log messages at the 'info' level.
const bunyan = require('bunyan');
const log = bunyan.createLogger({name: 'myapp'});
log.info('Hello, world!');
Logging with Different Levels
This feature allows you to log messages at different levels such as trace, debug, info, warn, error, and fatal.
const bunyan = require('bunyan');
const log = bunyan.createLogger({name: 'myapp'});
log.trace('Trace message');
log.debug('Debug message');
log.info('Info message');
log.warn('Warn message');
log.error('Error message');
log.fatal('Fatal message');
Child Loggers
This feature allows you to create child loggers that inherit properties from the parent logger but can also have additional context-specific properties.
const bunyan = require('bunyan');
const log = bunyan.createLogger({name: 'myapp'});
const child = log.child({widget_type: 'foo'});
child.info('Hello from child logger');
Serializers
This feature allows you to use serializers to format log messages, such as including request and response objects in a structured way.
const bunyan = require('bunyan');
const log = bunyan.createLogger({name: 'myapp', serializers: bunyan.stdSerializers});
log.info({req: {method: 'GET', url: '/'}}, 'Request received');
Winston is another popular logging library for Node.js that supports multiple transports (e.g., console, file, HTTP) and log levels. It is more flexible in terms of output formats and transport options compared to Bunyan.
Pino is a very fast JSON logger for Node.js, similar to Bunyan. It focuses on performance and low overhead, making it suitable for high-throughput applications. Pino also provides a CLI tool for pretty-printing logs.
Log4js is a logging library inspired by the Java log4j library. It provides a variety of appenders for different output targets and supports hierarchical loggers. It is more feature-rich but can be more complex to configure compared to Bunyan.
npm install --save @types/bunyan
This package contains type definitions for node-bunyan (https://github.com/trentm/node-bunyan).
Files were exported from https://www.github.com/DefinitelyTyped/DefinitelyTyped/tree/types-2.0/bunyan
Additional Details
These definitions were written by Alex Mikhalev https://github.com/amikhalev.
FAQs
TypeScript definitions for bunyan
The npm package @types/bunyan receives a total of 1,533,645 weekly downloads. As such, @types/bunyan popularity was classified as popular.
We found that @types/bunyan demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.