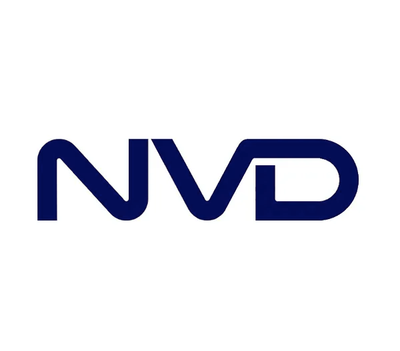
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
@types/cls-hooked
Advanced tools
TypeScript definitions for cls-hooked
@types/cls-hooked provides TypeScript type definitions for the cls-hooked package, which is used for context propagation across asynchronous calls in Node.js. It allows you to create a context that can be accessed throughout the lifecycle of a request, even across asynchronous boundaries.
Creating a Namespace
This feature allows you to create a new namespace. A namespace is a context that can be used to store and retrieve data across asynchronous calls.
const cls = require('cls-hooked');
const namespace = cls.createNamespace('myNamespace');
Setting and Getting Context
Within a namespace, you can set and get values that will be available throughout the lifecycle of the asynchronous operations.
namespace.run(() => {
namespace.set('key', 'value');
console.log(namespace.get('key')); // Outputs: value
});
Binding Functions to a Namespace
You can bind functions to a namespace so that they have access to the context set within that namespace.
const boundFunction = namespace.bind(() => {
console.log(namespace.get('key'));
});
namespace.run(() => {
namespace.set('key', 'value');
boundFunction(); // Outputs: value
});
The async_hooks module provides an API to track asynchronous resources in Node.js. It is a lower-level API compared to cls-hooked and requires more manual management of context propagation.
This package is similar to cls-hooked and provides a way to maintain context across asynchronous calls. However, it is older and less maintained compared to cls-hooked.
zone.js is a library for managing asynchronous context propagation in JavaScript. It is more commonly used in Angular applications but can be used in Node.js as well. It provides a more comprehensive solution for managing asynchronous contexts.
npm install --save @types/cls-hooked
This package contains type definitions for cls-hooked ( https://github.com/jeff-lewis/cls-hooked ).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/cls-hooked
Additional Details
These definitions were written by Leo Liang https://github.com/aleung.
FAQs
TypeScript definitions for cls-hooked
The npm package @types/cls-hooked receives a total of 894,264 weekly downloads. As such, @types/cls-hooked popularity was classified as popular.
We found that @types/cls-hooked demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.