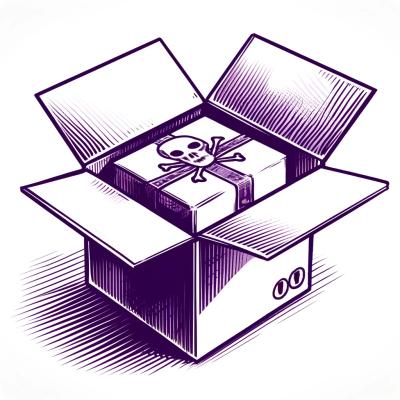
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@types/estraverse
Advanced tools
TypeScript definitions for estraverse
@types/estraverse provides TypeScript type definitions for the estraverse library, which is a tool for ECMAScript/JavaScript syntax tree traversal. It allows developers to walk through and manipulate the abstract syntax tree (AST) of JavaScript code.
Traversing the AST
This feature allows you to traverse the AST of JavaScript code. The `enter` and `leave` methods are called when entering and leaving each node in the AST, respectively.
const estraverse = require('estraverse');
const ast = { /* some AST */ };
estraverse.traverse(ast, {
enter: function (node, parent) {
console.log('Entering node:', node.type);
},
leave: function (node, parent) {
console.log('Leaving node:', node.type);
}
});
Replacing nodes in the AST
This feature allows you to replace nodes in the AST. In this example, all identifiers with the name 'oldName' are replaced with 'newName'.
const estraverse = require('estraverse');
const ast = { /* some AST */ };
const newAst = estraverse.replace(ast, {
enter: function (node) {
if (node.type === 'Identifier' && node.name === 'oldName') {
node.name = 'newName';
}
}
});
Custom node visitor keys
This feature allows you to define custom visitor keys for traversing non-standard AST nodes. In this example, a custom node type 'CustomNode' with a child node 'childNode' is defined.
const estraverse = require('estraverse');
const ast = { /* some AST */ };
const visitorKeys = {
CustomNode: ['childNode']
};
estraverse.traverse(ast, {
enter: function (node) {
console.log('Entering node:', node.type);
}
}, visitorKeys);
Esprima is a high-performance, standard-compliant ECMAScript parser that can be used to generate an AST from JavaScript code. While it focuses on parsing, it can be used in conjunction with other tools for AST traversal and manipulation.
babel-traverse is a tool from the Babel ecosystem that allows for efficient traversal and manipulation of the AST. It provides more advanced features and integrations with Babel plugins and transformations compared to estraverse.
Acorn is a small, fast, JavaScript-based JavaScript parser that generates an AST. While it is primarily focused on parsing, it can be extended with plugins to support AST traversal and manipulation.
npm install --save @types/estraverse
This package contains type definitions for estraverse (https://github.com/estools/estraverse).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/estraverse.
These definitions were written by Sanex3339, and Jason Kwok.
FAQs
TypeScript definitions for estraverse
The npm package @types/estraverse receives a total of 129,873 weekly downloads. As such, @types/estraverse popularity was classified as popular.
We found that @types/estraverse demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.