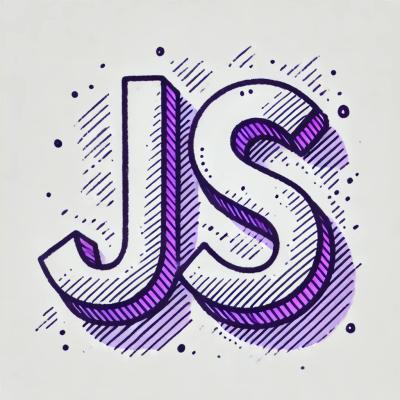
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
@types/jsforce
Advanced tools
@types/jsforce provides TypeScript definitions for the jsforce library, which is a powerful Salesforce API library for Node.js. It allows developers to interact with Salesforce services, including data manipulation, metadata management, and more, using TypeScript.
Authentication
This feature allows you to authenticate with Salesforce using a username and password. The code sample demonstrates how to establish a connection and log in to Salesforce.
const jsforce = require('jsforce');
const conn = new jsforce.Connection();
conn.login('username', 'password', function(err, userInfo) {
if (err) { return console.error(err); }
console.log('User ID: ' + userInfo.id);
console.log('Org ID: ' + userInfo.organizationId);
});
Querying Data
This feature allows you to query data from Salesforce using SOQL (Salesforce Object Query Language). The code sample demonstrates how to query Account records and log the results.
conn.query('SELECT Id, Name FROM Account', function(err, result) {
if (err) { return console.error(err); }
console.log('Total records: ' + result.totalSize);
console.log('Fetched records: ' + result.records.length);
});
CRUD Operations
This feature allows you to perform CRUD (Create, Read, Update, Delete) operations on Salesforce objects. The code sample demonstrates how to create a new Account record.
conn.sobject('Account').create({ Name: 'New Account' }, function(err, ret) {
if (err || !ret.success) { return console.error(err, ret); }
console.log('Created record id: ' + ret.id);
});
Metadata Management
This feature allows you to manage Salesforce metadata, such as custom objects and fields. The code sample demonstrates how to read metadata for a custom object.
conn.metadata.read('CustomObject', 'MyCustomObject__c', function(err, metadata) {
if (err) { return console.error(err); }
console.log('Metadata: ' + JSON.stringify(metadata));
});
node-salesforce is another Node.js module for interfacing with Salesforce. It provides similar functionalities to jsforce, such as authentication, querying, and CRUD operations. However, jsforce is generally considered more feature-rich and better maintained.
nforce is a Node.js client for Salesforce REST API. It offers functionalities like authentication, querying, and CRUD operations. Compared to jsforce, nforce is simpler and more lightweight but may lack some advanced features available in jsforce.
salesforce-connection is a lightweight library for connecting to Salesforce and performing basic operations. It is less comprehensive than jsforce but can be a good choice for simpler use cases.
npm install --save @types/jsforce
This package contains type definitions for jsforce (https://github.com/jsforce/jsforce).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/jsforce
Additional Details
These definitions were written by Dolan Miu https://github.com/dolanmiu, Kamil Ejsymont https://github.com/netes, Thomas Dvornik https://github.com/amphro, Tim Noonan https://github.com/tnoonan-salesforce, Abraham White https://github.com/whiteabelincoln, Borys Kupar https://github.com/borys-kupar.
FAQs
TypeScript definitions for jsforce
We found that @types/jsforce demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.