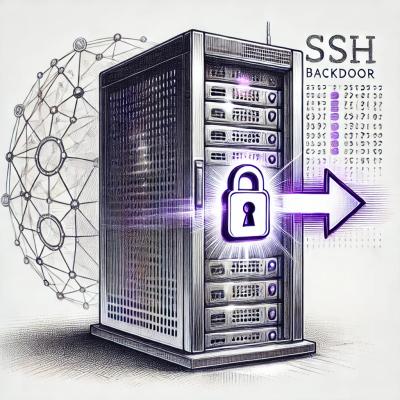
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@types/oauth2-server
Advanced tools
@types/oauth2-server provides TypeScript type definitions for the oauth2-server package, which is a complete, compliant, and well-tested module for implementing an OAuth2 server in Node.js.
Authorization Code Grant
This code demonstrates how to set up an authorization endpoint using the Authorization Code Grant type. The endpoint will handle authorization requests and return an authorization code.
const OAuth2Server = require('oauth2-server');
const Request = OAuth2Server.Request;
const Response = OAuth2Server.Response;
const oauth = new OAuth2Server({
model: require('./model')
});
app.post('/authorize', (req, res) => {
const request = new Request(req);
const response = new Response(res);
oauth.authorize(request, response)
.then((code) => {
res.json(code);
}).catch((err) => {
res.status(err.code || 500).json(err);
});
});
Token Grant
This code demonstrates how to set up a token endpoint. The endpoint will handle token requests and return an access token.
app.post('/token', (req, res) => {
const request = new Request(req);
const response = new Response(res);
oauth.token(request, response)
.then((token) => {
res.json(token);
}).catch((err) => {
res.status(err.code || 500).json(err);
});
});
Token Authentication
This code demonstrates how to set up a secure endpoint that requires token authentication. The endpoint will return secure data if the token is valid.
app.get('/secure', (req, res) => {
const request = new Request(req);
const response = new Response(res);
oauth.authenticate(request, response)
.then((token) => {
res.json({ message: 'Secure data' });
}).catch((err) => {
res.status(err.code || 500).json(err);
});
});
oauth2orize is a toolkit for implementing OAuth2 servers in Node.js. It provides a more modular approach compared to oauth2-server, allowing developers to customize the authorization and token issuance processes more granularly.
node-oauth2-server is another implementation of an OAuth2 server for Node.js. It is similar to oauth2-server but offers a different API and some additional features like support for refresh tokens and more extensive error handling.
simple-oauth2 is a library for implementing OAuth2 clients in Node.js. While it focuses on the client side of OAuth2, it can be used in conjunction with an OAuth2 server to handle token management and authentication.
npm install --save @types/oauth2-server
This package contains type definitions for Node OAuth2 Server (https://github.com/oauthjs/node-oauth2-server).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/oauth2-server
Additional Details
These definitions were written by Robbie Van Gorkom https://github.com/vangorra, Charles Irick https://github.com/cirick, Daniel Fischer https://github.com/d-fischer.
FAQs
TypeScript definitions for oauth2-server
The npm package @types/oauth2-server receives a total of 281,727 weekly downloads. As such, @types/oauth2-server popularity was classified as popular.
We found that @types/oauth2-server demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.