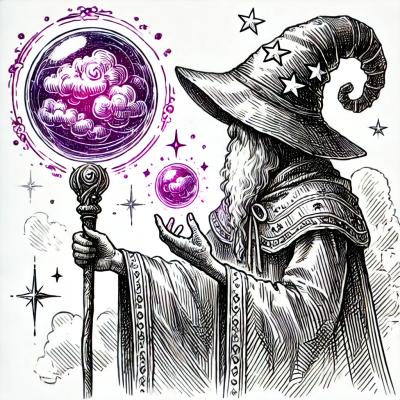
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
The @types/qs package provides TypeScript type definitions for the qs library, which is used for parsing and stringifying query strings. It allows developers to work with query strings in a type-safe manner, ensuring that the code adheres to the expected structure and types of the qs library.
Parsing query strings
This feature allows you to parse query strings into an object, making it easier to access the query parameters.
import * as qs from 'qs';
const query = '?a=c&b=d';
const parsed = qs.parse(query);
console.log(parsed); // { a: 'c', b: 'd' }
Stringifying objects
This feature enables you to convert an object into a query string, useful for creating URLs with query parameters.
import * as qs from 'qs';
const obj = { a: 'c', b: 'd' };
const stringified = qs.stringify(obj);
console.log(stringified); // 'a=c&b=d'
The query-string package offers parsing and stringifying of query strings. It provides a more modern API and supports newer JavaScript features compared to qs, but lacks some of the deep object serialization capabilities of qs.
This is a built-in Web API in modern browsers for working with query strings, similar to qs. It doesn't require installation but is not as feature-rich as qs, especially in terms of parsing options and array handling.
npm install --save @types/qs
This package contains type definitions for qs (https://github.com/ljharb/qs).
Files were exported from https://www.github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/qs
Additional Details
These definitions were written by Roman Korneev https://github.com/RWander, Leon Yu https://github.com/leonyu, Belinda Teh https://github.com/tehbelinda, Melvin Lee https://github.com/zyml.
FAQs
TypeScript definitions for qs
The npm package @types/qs receives a total of 15,479,684 weekly downloads. As such, @types/qs popularity was classified as popular.
We found that @types/qs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.