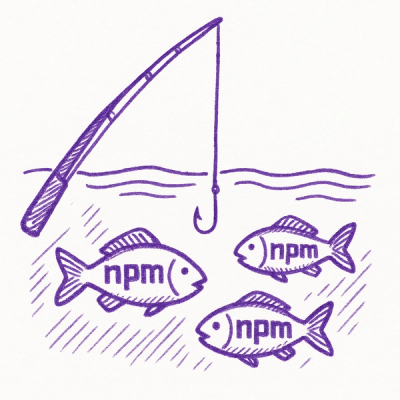
Security News
/Research
npm Phishing Email Targets Developers with Typosquatted Domain
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.
@types/stack-utils
Advanced tools
TypeScript definitions for stack-utils
@types/stack-utils provides TypeScript type definitions for the stack-utils library, which is used for parsing and manipulating stack traces in JavaScript. This can be particularly useful for debugging and error handling.
Parsing Stack Traces
This feature allows you to parse a stack trace into a more readable and structured format. The code sample demonstrates how to create a StackUtils instance and parse the stack trace of a new Error.
const StackUtils = require('stack-utils');
const stack = new StackUtils({ cwd: process.cwd(), internals: StackUtils.nodeInternals() });
const parsedStack = stack.parse(new Error().stack);
console.log(parsedStack);
Cleaning Stack Traces
This feature allows you to clean a stack trace by removing internal Node.js entries and other noise. The code sample shows how to clean the stack trace of a new Error.
const StackUtils = require('stack-utils');
const stack = new StackUtils({ cwd: process.cwd(), internals: StackUtils.nodeInternals() });
const cleanedStack = stack.clean(new Error().stack);
console.log(cleanedStack);
Customizing Stack Trace Output
This feature allows you to customize the output format of stack traces. The code sample demonstrates how to wrap call sites to format the stack trace entries in a custom way.
const StackUtils = require('stack-utils');
const stack = new StackUtils({ cwd: process.cwd(), internals: StackUtils.nodeInternals(), wrapCallSite: (callSite) => ({
getFileName: () => callSite.getFileName(),
getLineNumber: () => callSite.getLineNumber(),
getColumnNumber: () => callSite.getColumnNumber(),
toString: () => `${callSite.getFileName()}:${callSite.getLineNumber()}:${callSite.getColumnNumber()}`
}) });
const customStack = stack.clean(new Error().stack);
console.log(customStack);
error-stack-parser is a library for parsing error stack traces into a more readable format. It is similar to stack-utils in that it provides functionality for parsing stack traces, but it focuses more on browser compatibility and has a simpler API.
stacktrace-js is a library for generating, parsing, and manipulating stack traces in JavaScript. It offers more comprehensive features compared to stack-utils, including support for source maps and browser compatibility.
traceback is a library for handling stack traces in Node.js. It provides similar functionality to stack-utils, such as parsing and cleaning stack traces, but it also includes additional features like filtering and formatting options.
npm install --save @types/stack-utils
This package contains type definitions for stack-utils (https://github.com/tapjs/stack-utils#readme).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/stack-utils.
export = StackUtils;
declare class StackUtils {
static nodeInternals(): RegExp[];
constructor(options?: StackUtils.Options);
clean(stack: string | string[]): string;
capture(limit?: number, startStackFunction?: Function): StackUtils.CallSite[];
capture(startStackFunction: Function): StackUtils.CallSite[];
captureString(limit?: number, startStackFunction?: Function): string;
captureString(startStackFunction: Function): string;
at(startStackFunction?: Function): StackUtils.CallSiteLike;
parseLine(line: string): StackUtils.StackLineData | null;
}
declare namespace StackUtils {
interface Options {
internals?: RegExp[] | undefined;
ignoredPackages?: string[] | undefined;
cwd?: string | undefined;
wrapCallSite?(callSite: CallSite): CallSite;
}
interface CallSite {
getThis(): object | undefined;
getTypeName(): string;
getFunction(): Function | undefined;
getFunctionName(): string;
getMethodName(): string | null;
getFileName(): string | undefined;
getLineNumber(): number;
getColumnNumber(): number;
getEvalOrigin(): CallSite | string;
isToplevel(): boolean;
isEval(): boolean;
isNative(): boolean;
isConstructor(): boolean;
}
interface CallSiteLike extends StackData {
type?: string | undefined;
}
interface StackLineData extends StackData {
evalLine?: number | undefined;
evalColumn?: number | undefined;
evalFile?: string | undefined;
}
interface StackData {
line?: number | undefined;
column?: number | undefined;
file?: string | undefined;
constructor?: boolean | undefined;
evalOrigin?: string | undefined;
native?: boolean | undefined;
function?: string | undefined;
method?: string | undefined;
}
}
These definitions were written by BendingBender.
FAQs
TypeScript definitions for stack-utils
The npm package @types/stack-utils receives a total of 31,306,176 weekly downloads. As such, @types/stack-utils popularity was classified as popular.
We found that @types/stack-utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.
Security News
Knip hits 500 releases with v5.62.0, refining TypeScript config detection and updating plugins as monthly npm downloads approach 12M.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.