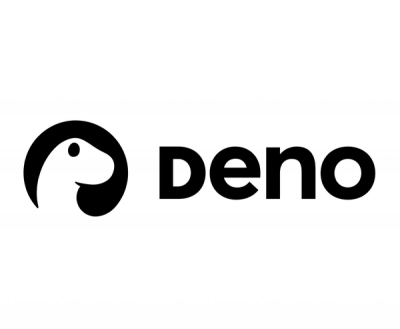
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@types/tapable
Advanced tools
The @types/tapable package provides TypeScript type definitions for the Tapable library, which is used primarily in webpack for its plugin architecture. Tapable allows you to add and apply plugins to a JavaScript project. It offers various hooks like SyncHook, AsyncParallelHook, and more, which can be used to handle synchronous and asynchronous operations.
SyncHook
SyncHook allows for the synchronous execution of functions. The functions attached via `tap` will be executed in the order they were added when `call` is invoked.
import { SyncHook } from 'tapable';
const hook = new SyncHook(['arg1']);
hook.tap('PluginName', (arg1) => {
console.log(`Value received: ${arg1}`);
});
hook.call('test');
AsyncParallelHook
AsyncParallelHook allows for the parallel execution of asynchronous functions. Functions can be added using `tapPromise` and are executed with `promise`.
import { AsyncParallelHook } from 'tapable';
const asyncHook = new AsyncParallelHook(['arg1']);
asyncHook.tapPromise('PluginName', (arg1) => {
return new Promise((resolve, reject) => {
setTimeout(() => {
console.log(`Async value: ${arg1}`);
resolve();
}, 1000);
});
});
asyncHook.promise('test').then(() => {
console.log('All async plugins have finished');
});
Mitt is a tiny functional event emitter / pubsub library. It offers similar event handling capabilities but lacks the sophisticated hook system and the distinction between synchronous and asynchronous operations found in Tapable.
EventEmitter3 is a high-performance event emitter. While it provides efficient event handling like Tapable, it does not support the hook-based plugin architecture that allows for ordered execution and lifecycle management in Tapable.
npm install --save @types/tapable
This package contains type definitions for tapable (https://github.com/webpack/tapable.git).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/tapable/v1.
These definitions were written by e-cloud, and John Reilly.
FAQs
TypeScript definitions for tapable
The npm package @types/tapable receives a total of 3,521,318 weekly downloads. As such, @types/tapable popularity was classified as popular.
We found that @types/tapable demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.