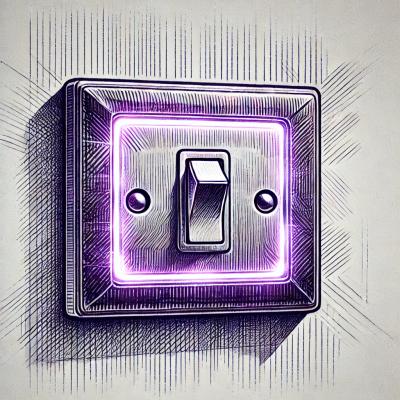
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
@types/vfile
Advanced tools
Stub TypeScript definitions entry for vfile, which provides its own types definitions
@types/vfile provides TypeScript definitions for the vfile package, which is a virtual file format used to represent files, their contents, and associated metadata. It is commonly used in the unified ecosystem for processing text and syntax trees.
Creating a VFile
This feature allows you to create a virtual file with a specified path and contents. The vfile object can then be used to manipulate the file's metadata and contents.
const vfile = require('vfile');
const file = vfile({
path: '~/example.txt',
contents: 'Hello, world!'
});
console.log(file.path); // '~/example.txt'
console.log(file.contents); // 'Hello, world!'
Reading and Writing Metadata
This feature allows you to read and write metadata associated with the virtual file. Metadata can be any arbitrary data that you want to associate with the file.
const vfile = require('vfile');
const file = vfile({
path: '~/example.txt',
contents: 'Hello, world!'
});
file.data.title = 'Example File';
console.log(file.data.title); // 'Example File'
Handling Messages
This feature allows you to attach messages to the virtual file, which can be used for warnings, errors, or other notices. Each message can have a reason and a location within the file.
const vfile = require('vfile');
const file = vfile();
file.message('This is a warning', {line: 2, column: 4});
console.log(file.messages[0].reason); // 'This is a warning'
console.log(file.messages[0].location); // { line: 2, column: 4 }
Vinyl is a virtual file format used in the Gulp ecosystem. It is similar to vfile in that it represents files and their contents, but it is more focused on stream-based processing. Vinyl files can be piped through various Gulp plugins for transformations.
File-type is a package that detects the file type of a Buffer or Uint8Array. While it does not provide a virtual file format like vfile, it is useful for determining the type of a file based on its contents, which can be a complementary functionality.
fs-extra is an extension of the Node.js 'fs' module that adds extra methods for working with the file system. It does not provide a virtual file format, but it offers many utility functions for reading, writing, and manipulating files on disk.
This is a stub types definition for vfile (https://github.com/vfile/vfile).
vfile provides its own type definitions, so you don't need @types/vfile installed!
FAQs
Stub TypeScript definitions entry for vfile, which provides its own types definitions
The npm package @types/vfile receives a total of 121,525 weekly downloads. As such, @types/vfile popularity was classified as popular.
We found that @types/vfile demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.