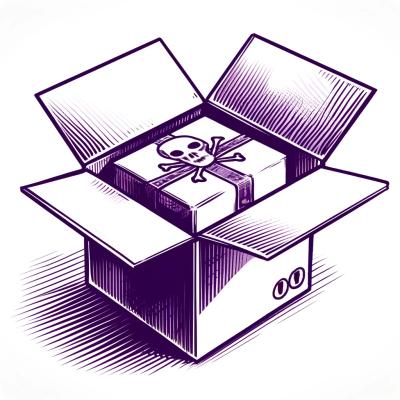
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@types/xmldom
Advanced tools
TypeScript definitions for xmldom
@types/xmldom provides TypeScript type definitions for the xmldom library, which is used to parse and serialize XML documents in a DOM-like structure.
Parsing XML
This feature allows you to parse an XML string into a DOM Document object, which can then be traversed and manipulated using standard DOM methods.
const { DOMParser } = require('xmldom');
const xmlString = '<root><child>content</child></root>';
const doc = new DOMParser().parseFromString(xmlString, 'text/xml');
console.log(doc.documentElement.nodeName); // Output: root
Serializing XML
This feature allows you to serialize a DOM Document object back into an XML string, which can be useful for saving or transmitting the XML data.
const { DOMParser, XMLSerializer } = require('xmldom');
const xmlString = '<root><child>content</child></root>';
const doc = new DOMParser().parseFromString(xmlString, 'text/xml');
const serializedXml = new XMLSerializer().serializeToString(doc);
console.log(serializedXml); // Output: <root><child>content</child></root>
Manipulating XML
This feature allows you to manipulate the XML DOM by adding, removing, or modifying elements and attributes, providing a way to dynamically change the XML structure.
const { DOMParser, XMLSerializer } = require('xmldom');
const xmlString = '<root><child>content</child></root>';
const doc = new DOMParser().parseFromString(xmlString, 'text/xml');
const newElement = doc.createElement('newChild');
newElement.textContent = 'new content';
doc.documentElement.appendChild(newElement);
const serializedXml = new XMLSerializer().serializeToString(doc);
console.log(serializedXml); // Output: <root><child>content</child><newChild>new content</newChild></root>
xml2js is a popular library for converting XML to JavaScript objects and vice versa. Unlike xmldom, which provides a DOM-like interface, xml2js focuses on converting XML to JSON and back, making it easier to work with XML data in a more JavaScript-friendly format.
fast-xml-parser is a high-performance XML parser that converts XML to JSON and vice versa. It is known for its speed and efficiency, making it a good choice for performance-critical applications. Unlike xmldom, it does not provide a DOM-like interface but focuses on fast parsing and serialization.
libxmljs is a library that provides a comprehensive set of XML parsing and manipulation features, including XPath support. It is based on the libxml2 library and offers a more feature-rich and performant alternative to xmldom, especially for complex XML processing tasks.
npm install --save @types/xmldom
This package contains type definitions for xmldom (https://github.com/xmldom/xmldom).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/xmldom.
/// <reference lib="dom" />
declare namespace xmldom {
var DOMParser: DOMParserStatic;
var XMLSerializer: XMLSerializerStatic;
var DOMImplementation: DOMImplementationStatic;
interface DOMImplementationStatic {
new(): DOMImplementation;
}
interface DOMParserStatic {
new(): DOMParser;
new(options: Options): DOMParser;
}
interface XMLSerializerStatic {
new(): XMLSerializer;
}
interface DOMParser {
parseFromString(xmlsource: string, mimeType?: string): Document;
}
interface XMLSerializer {
serializeToString(node: Node): string;
}
interface Options {
locator?: any;
errorHandler?: ErrorHandlerFunction | ErrorHandlerObject | undefined;
}
interface ErrorHandlerFunction {
(level: string, msg: any): any;
}
interface ErrorHandlerObject {
warning?: ((msg: any) => any) | undefined;
error?: ((msg: any) => any) | undefined;
fatalError?: ((msg: any) => any) | undefined;
}
}
export = xmldom;
FAQs
TypeScript definitions for xmldom
The npm package @types/xmldom receives a total of 217,396 weekly downloads. As such, @types/xmldom popularity was classified as popular.
We found that @types/xmldom demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.