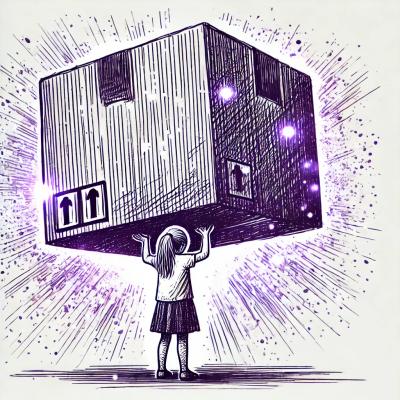
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
@typescript-eslint/eslint-plugin
Advanced tools
The @typescript-eslint/eslint-plugin package is an ESLint plugin that contains a set of ESLint rules that are specifically designed for TypeScript code. It helps in identifying and reporting on patterns found in TypeScript code, and it can be used to enforce a wide range of coding standards and conventions.
Type-aware linting
This feature allows for rules that require type information. For example, the 'strict-boolean-expressions' rule ensures that boolean expressions are clear and error-free by considering the types involved in the expression.
/* eslint @typescript-eslint/strict-boolean-expressions: 'error' */
function isTruthy(value: any): boolean {
return Boolean(value);
}
Code style enforcement
Enforces naming conventions for everything from variables to type parameters. This example enforces camelCase naming for variables.
/* eslint @typescript-eslint/naming-convention: ['error', { 'selector': 'variable', 'format': ['camelCase'] }] */
let myVariable = 1;
Accessibility checks
This feature requires functions and methods to explicitly define their return type to improve code readability and maintainability.
/* eslint @typescript-eslint/explicit-function-return-type: 'warn' */
function add(x: number, y: number) {
return x + y;
}
This package provides linting rules for React and JSX. It's similar to @typescript-eslint/eslint-plugin in that it extends ESLint's capabilities to a specific language extension, but it focuses on React rather than TypeScript.
This package is designed for linting Vue.js templates and scripts. Like @typescript-eslint/eslint-plugin, it provides additional rules that are specific to the Vue.js framework, complementing the standard ESLint rules.
This plugin provides a set of rules that help ensure proper imports, exports, and module structure. While it's not specific to TypeScript, it complements @typescript-eslint/eslint-plugin by enforcing best practices in module usage.
Make sure you have TypeScript and @typescript-eslint/parser
installed, then install the plugin:
npm i @typescript-eslint/eslint-plugin --save-dev
It is important that you use the same version number for @typescript-eslint/parser
and @typescript-eslint/eslint-plugin
.
Note: If you installed ESLint globally (using the -g
flag) then you must also install @typescript-eslint/eslint-plugin
globally.
Add @typescript-eslint/parser
to the parser
field and @typescript-eslint
to the plugins section of your .eslintrc
configuration file:
{
"parser": "@typescript-eslint/parser",
"plugins": ["@typescript-eslint"]
}
Then configure the rules you want to use under the rules section.
{
"parser": "@typescript-eslint/parser",
"plugins": ["@typescript-eslint"],
"rules": {
"@typescript-eslint/rule-name": "error"
}
}
You can also enable all the recommended rules for our plugin. Add plugin:@typescript-eslint/recommended
in extends:
{
"extends": ["plugin:@typescript-eslint/recommended"]
}
You can also use eslint:recommended (the set of rules which are recommended for all projects by the ESLint Team) with this plugin. As noted in the root README, not all eslint core rules are compatible with TypeScript, so you need to add both eslint:recommended
and plugin:@typescript-eslint/eslint-recommended
(which will adjust the one from eslint appropriately for TypeScript) to your config:
{
"extends": [
"eslint:recommended",
"plugin:@typescript-eslint/eslint-recommended",
"plugin:@typescript-eslint/recommended"
]
}
As of version 2 of this plugin, by design, none of the rules in the main recommended
config require type-checking in order to run. This means that they are more lightweight and faster to run.
Some highly valuable rules simply require type-checking in order to be implemented correctly, however, so we provide an additional config you can extend from called recommended-requiring-type-checking
. You would apply this in addition to the recommended configs previously mentioned, e.g.:
{
"extends": [
"eslint:recommended",
"plugin:@typescript-eslint/eslint-recommended",
"plugin:@typescript-eslint/recommended",
"plugin:@typescript-eslint/recommended-requiring-type-checking"
]
}
Pro Tip: For larger codebases you may want to consider splitting our linting into two separate stages: 1. fast feedback rules which operate purely based on syntax (no type-checking), 2. rules which are based on semantics (type-checking).
NOTE: If you want to use rules which require type information, you will need to specify a path to your tsconfig.json file in the "project" property of "parserOptions". If you do not do this, you will get a runtime error which explains this.
{
"parser": "@typescript-eslint/parser",
"parserOptions": {
"project": "./tsconfig.json"
},
"plugins": ["@typescript-eslint"],
"rules": {
"@typescript-eslint/restrict-plus-operands": "error"
}
}
See @typescript-eslint/parser's README.md for more information on the available "parserOptions".
Note: Make sure to use eslint --ext .js,.ts
since by default eslint
will only search for .js files.
Install eslint-config-prettier
to disable our code formatting related rules:
{
"extends": [
"plugin:@typescript-eslint/recommended",
"prettier",
"prettier/@typescript-eslint"
]
}
Note: Make sure you have eslint-config-prettier@4.0.0
or newer.
Airbnb has two configs, a base one eslint-config-airbnb-base
and one that includes rules for React eslint-config-airbnb
.
First you'll need to install the config according to the instructions in one of the links above. npx install-peerdeps --dev eslint-config-airbnb
or npx install-peerdeps --dev eslint-config-airbnb-base
should work if you are using npm 5+.
Then you should add airbnb
(or airbnb-base
) to your extends
section of .eslintrc
. You might also want to turn on plugin:@typescript-eslint/recommended
as well to enable all of the recommended rules.
{
"extends": ["airbnb-base", "plugin:@typescript-eslint/recommended"]
}
Note: You can use Airbnb's rules alongside Prettier, see Usage with Prettier
Key: :heavy_check_mark: = recommended, :wrench: = fixable, :thought_balloon: = requires type information
Name | Description | :heavy_check_mark: | :wrench: | :thought_balloon: |
---|---|---|---|---|
@typescript-eslint/adjacent-overload-signatures | Require that member overloads be consecutive | :heavy_check_mark: | ||
@typescript-eslint/array-type | Requires using either T[] or Array<T> for arrays | :wrench: | ||
@typescript-eslint/await-thenable | Disallows awaiting a value that is not a Thenable | :heavy_check_mark: | :thought_balloon: | |
@typescript-eslint/ban-ts-ignore | Bans “// @ts-ignore” comments from being used | :heavy_check_mark: | ||
@typescript-eslint/ban-types | Bans specific types from being used | :heavy_check_mark: | :wrench: | |
@typescript-eslint/brace-style | Enforce consistent brace style for blocks | :wrench: | ||
@typescript-eslint/camelcase | Enforce camelCase naming convention | :heavy_check_mark: | ||
@typescript-eslint/class-name-casing | Require PascalCased class and interface names | :heavy_check_mark: | ||
@typescript-eslint/consistent-type-assertions | Enforces consistent usage of type assertions. | :heavy_check_mark: | ||
@typescript-eslint/consistent-type-definitions | Consistent with type definition either interface or type | :wrench: | ||
@typescript-eslint/explicit-function-return-type | Require explicit return types on functions and class methods | :heavy_check_mark: | ||
@typescript-eslint/explicit-member-accessibility | Require explicit accessibility modifiers on class properties and methods | |||
@typescript-eslint/func-call-spacing | Require or disallow spacing between function identifiers and their invocations | :wrench: | ||
@typescript-eslint/generic-type-naming | Enforces naming of generic type variables | |||
@typescript-eslint/indent | Enforce consistent indentation | :wrench: | ||
@typescript-eslint/interface-name-prefix | Require that interface names should or should not prefixed with I | :heavy_check_mark: | ||
@typescript-eslint/member-delimiter-style | Require a specific member delimiter style for interfaces and type literals | :heavy_check_mark: | :wrench: | |
@typescript-eslint/member-naming | Enforces naming conventions for class members by visibility | |||
@typescript-eslint/member-ordering | Require a consistent member declaration order | |||
@typescript-eslint/no-array-constructor | Disallow generic Array constructors | :heavy_check_mark: | :wrench: | |
@typescript-eslint/no-dynamic-delete | Bans usage of the delete operator with computed key expressions | :wrench: | ||
@typescript-eslint/no-empty-function | Disallow empty functions | :heavy_check_mark: | ||
@typescript-eslint/no-empty-interface | Disallow the declaration of empty interfaces | :heavy_check_mark: | :wrench: | |
@typescript-eslint/no-explicit-any | Disallow usage of the any type | :heavy_check_mark: | :wrench: | |
@typescript-eslint/no-extra-non-null-assertion | Disallow extra non-null assertion | |||
@typescript-eslint/no-extra-parens | Disallow unnecessary parentheses | :wrench: | ||
@typescript-eslint/no-extraneous-class | Forbids the use of classes as namespaces | |||
@typescript-eslint/no-floating-promises | Requires Promise-like values to be handled appropriately. | :thought_balloon: | ||
@typescript-eslint/no-for-in-array | Disallow iterating over an array with a for-in loop | :heavy_check_mark: | :thought_balloon: | |
@typescript-eslint/no-inferrable-types | Disallows explicit type declarations for variables or parameters initialized to a number, string, or boolean | :heavy_check_mark: | :wrench: | |
@typescript-eslint/no-magic-numbers | Disallows magic numbers | |||
@typescript-eslint/no-misused-new | Enforce valid definition of new and constructor | :heavy_check_mark: | ||
@typescript-eslint/no-misused-promises | Avoid using promises in places not designed to handle them | :heavy_check_mark: | :thought_balloon: | |
@typescript-eslint/no-namespace | Disallow the use of custom TypeScript modules and namespaces | :heavy_check_mark: | ||
@typescript-eslint/no-non-null-assertion | Disallows non-null assertions using the ! postfix operator | :heavy_check_mark: | ||
@typescript-eslint/no-parameter-properties | Disallow the use of parameter properties in class constructors | |||
@typescript-eslint/no-require-imports | Disallows invocation of require() | |||
@typescript-eslint/no-this-alias | Disallow aliasing this | :heavy_check_mark: | ||
@typescript-eslint/no-type-alias | Disallow the use of type aliases | |||
@typescript-eslint/no-unnecessary-condition | Prevents conditionals where the type is always truthy or always falsy | :thought_balloon: | ||
@typescript-eslint/no-unnecessary-qualifier | Warns when a namespace qualifier is unnecessary | :wrench: | :thought_balloon: | |
@typescript-eslint/no-unnecessary-type-arguments | Warns if an explicitly specified type argument is the default for that type parameter | :wrench: | :thought_balloon: | |
@typescript-eslint/no-unnecessary-type-assertion | Warns if a type assertion does not change the type of an expression | :heavy_check_mark: | :wrench: | :thought_balloon: |
@typescript-eslint/no-untyped-public-signature | Requires that all public method arguments and return type will be explicitly typed | |||
@typescript-eslint/no-unused-expressions | Disallow unused expressions | |||
@typescript-eslint/no-unused-vars | Disallow unused variables | :heavy_check_mark: | ||
@typescript-eslint/no-unused-vars-experimental | Disallow unused variables and arguments. | :thought_balloon: | ||
@typescript-eslint/no-use-before-define | Disallow the use of variables before they are defined | :heavy_check_mark: | ||
@typescript-eslint/no-useless-constructor | Disallow unnecessary constructors | |||
@typescript-eslint/no-var-requires | Disallows the use of require statements except in import statements | :heavy_check_mark: | ||
@typescript-eslint/prefer-for-of | Prefer a ‘for-of’ loop over a standard ‘for’ loop if the index is only used to access the array being iterated | |||
@typescript-eslint/prefer-function-type | Use function types instead of interfaces with call signatures | :wrench: | ||
@typescript-eslint/prefer-includes | Enforce includes method over indexOf method | :heavy_check_mark: | :wrench: | :thought_balloon: |
@typescript-eslint/prefer-namespace-keyword | Require the use of the namespace keyword instead of the module keyword to declare custom TypeScript modules | :heavy_check_mark: | :wrench: | |
@typescript-eslint/prefer-nullish-coalescing | Enforce the usage of the nullish coalescing operator instead of logical chaining | :wrench: | :thought_balloon: | |
@typescript-eslint/prefer-optional-chain | Prefer using concise optional chain expressions instead of chained logical ands | :wrench: | ||
@typescript-eslint/prefer-readonly | Requires that private members are marked as readonly if they're never modified outside of the constructor | :wrench: | :thought_balloon: | |
@typescript-eslint/prefer-regexp-exec | Prefer RegExp#exec() over String#match() if no global flag is provided | :heavy_check_mark: | :thought_balloon: | |
@typescript-eslint/prefer-string-starts-ends-with | Enforce the use of String#startsWith and String#endsWith instead of other equivalent methods of checking substrings | :heavy_check_mark: | :wrench: | :thought_balloon: |
@typescript-eslint/promise-function-async | Requires any function or method that returns a Promise to be marked async | :thought_balloon: | ||
@typescript-eslint/quotes | Enforce the consistent use of either backticks, double, or single quotes | :wrench: | ||
@typescript-eslint/require-array-sort-compare | Enforce giving compare argument to Array#sort | :thought_balloon: | ||
@typescript-eslint/require-await | Disallow async functions which have no await expression | :heavy_check_mark: | :thought_balloon: | |
@typescript-eslint/restrict-plus-operands | When adding two variables, operands must both be of type number or of type string | :thought_balloon: | ||
@typescript-eslint/restrict-template-expressions | Enforce template literal expressions to be of string type | :thought_balloon: | ||
@typescript-eslint/return-await | Rules for awaiting returned promises | :thought_balloon: | ||
@typescript-eslint/semi | Require or disallow semicolons instead of ASI | :wrench: | ||
@typescript-eslint/space-before-function-paren | enforce consistent spacing before function definition opening parenthesis | :wrench: | ||
@typescript-eslint/strict-boolean-expressions | Restricts the types allowed in boolean expressions | :thought_balloon: | ||
@typescript-eslint/triple-slash-reference | Sets preference level for triple slash directives versus ES6-style import declarations | :heavy_check_mark: | ||
@typescript-eslint/type-annotation-spacing | Require consistent spacing around type annotations | :heavy_check_mark: | :wrench: | |
@typescript-eslint/typedef | Requires type annotations to exist | |||
@typescript-eslint/unbound-method | Enforces unbound methods are called with their expected scope | :heavy_check_mark: | :thought_balloon: | |
@typescript-eslint/unified-signatures | Warns for any two overloads that could be unified into one by using a union or an optional/rest parameter |
FAQs
TypeScript plugin for ESLint
The npm package @typescript-eslint/eslint-plugin receives a total of 24,887,278 weekly downloads. As such, @typescript-eslint/eslint-plugin popularity was classified as popular.
We found that @typescript-eslint/eslint-plugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.