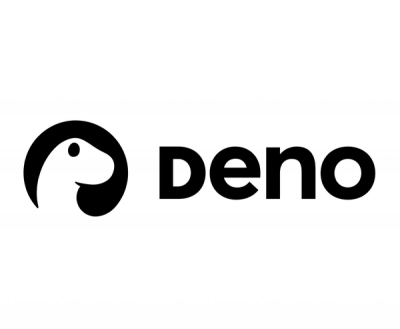
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@unleash/nextjs
Advanced tools
🏗️ Early version
We need your feedback. Share your comments in 🗣️ GitHub Discussions or on Unleash community 💬 Slack server.
This package allows easy integration of Unleash feature flags in a Next.js application.
To install, simply run:
npm install @unleash/nextjs
# or
yarn add @unleash/nextjs
# or
pnpm add @unleash/nextjs
This package will attempt to load configuration from Next.js Environment variables.
Prefixable | Variable | Default | Used in |
---|---|---|---|
NEXT_PUBLIC_ | UNLEASH_BASE_URL | http://localhost:4242/api | FlagProvider , getFrontendFlags |
No | UNLEASH_API_TOKEN | default:development.unleash-insecure-api-token | getDefinitions |
NEXT_PUBLIC_ | UNLEASH_FRONTEND_API_TOKEN | default:development.unleash-insecure-frontend-api-token | FlagProvider , getFrontendFlags |
NEXT_PUBLIC_ | UNLEASH_APP_NAME | nextjs | FlagProvider , getFrontendFlags , getDefinitions |
If you plan to use configuration in the browser, add NEXT_PUBLIC_
prefix.
If both are defined and available, private variable takes priority.
You can use both to have different values on client-side and server-side.
Fastest way to get started is to connect frontend directly to Unleash. You can find out more about direct Front-end API access in our documentation, including a guide on how to setup a client-side SDK key.
import type { AppProps } from "next/app";
import { FlagProvider } from "@unleash/nextjs";
export default function App({ Component, pageProps }: AppProps) {
return (
<FlagProvider
// config prop is optional if environment variables are set
config={{
url: "http://localhost:4242/api", // this will override NEXT_PUBLIC_UNLEASH_BASE_URL
clientKey: "<Frontend_API_token>", // NEXT_PUBLIC_UNLEASH_FRONTEND_API_TOKEN
appName: "nextjs", // NEXT_PUBLIC_UNLEASH_APP_NAME
refreshInterval: 15, // additional client configuration
}}
>
<Component {...pageProps} />
</FlagProvider>
);
}
With <FlagProvider />
in place you can now use hooks like: useFlag
, useVariant
, or useFlagsStatus
to block rendering until flags are ready.
import { useFlag } from "@unleash/nextjs";
const YourComponent = () => {
const isEnabled = useFlag("nextjs-poc");
return <>{isEnabled ? "ENABLED" : "DISABLED"}</>;
};
If you only plan to use Unleash client-side React SDK now also works with Next.js. Check documentation there for more examples.
With same access as in the client-side example above you can resolve Unleash feature flags when building static pages.
Use getFrontendFlags
to load
import { flagsClient, getFrontendFlags, type IVariant } from "@unleash/nextjs";
import type { GetStaticProps, NextPage } from "next";
type Data = {
isEnabled: boolean;
variant: IVariant;
};
const ExamplePage: NextPage<Data> = ({ isEnabled, variant }) => (
<>
Flag status: {isEnabled ? "ENABLED" : "DISABLED"}
<br />
Variant: {variant.name}
</>
);
export const getStaticProps: GetStaticProps<Data> = async (_ctx) => {
/* Using server-side SDK: */
const definitions = await getDefinitions();
const { toggles } = evaluateFlags(definitions);
/* Or with Proxy/Frontend API */
// const { toggles } = await getFrontendFlags();
const flags = flagsClient(toggles);
return {
props: {
isEnabled: flags.isEnabled("nextjs-poc"),
variant: flags.getVariant("nextjs-poc"),
},
};
};
export default ExamplePage;
The same approach will work for ISR (Incremental Static Regeneration).
import {
flagsClient,
evaluateFlags,
getDefinitions,
type IVariant,
} from "@unleash/nextjs";
import type { GetServerSideProps, NextPage } from "next";
type Data = {
isEnabled: boolean;
};
const ExamplePage: NextPage<Data> = ({ isEnabled }) => (
<>Flag status: {isEnabled ? "ENABLED" : "DISABLED"}</>
);
export const getServerSideProps: GetServerSideProps<Data> = async (ctx) => {
const sessionId =
ctx.req.cookies["unleash-session-id"] ||
`${Math.floor(Math.random() * 1_000_000_000)}`;
ctx.res.setHeader("set-cookie", `unleash-session-id=${sessionId}; path=/;`);
const context = {
sessionId, // needed for stickiness
// userId: "123" // etc
};
const { toggles } = await getFrontendFlags(); // Use Proxy/Frontend API
const flags = flagsClient(toggles);
return {
props: {
isEnabled: flags.isEnabled("nextjs-poc"),
},
};
};
export default ExamplePage;
You can bootstrap Unleash React SDK to have values loaded from the start. Initial value can be customized server-side.
import App, { AppContext, type AppProps } from "next/app";
import {
FlagProvider,
getFrontendFlags,
type IMutableContext,
type IToggle,
} from "@unleash/nextjs";
type Data = {
toggles: IToggle[];
context: IMutableContext;
};
export default function CustomApp({
Component,
pageProps,
toggles,
context,
}: AppProps & Data) {
return (
<FlagProvider
config={{
bootstrap: toggles,
context,
}}
>
<Component {...pageProps} />
</FlagProvider>
);
}
CustomApp.getInitialProps = async (ctx: AppContext) => {
const context = {
userId: "123",
};
const { toggles } = await getFrontendFlags(); // use Unleash Proxy
return {
...(await App.getInitialProps(ctx)),
bootstrap: toggles,
context, // pass context along so client can refetch correct values
};
};
Unleash Next.js SDK can run on Edge Runtime and in Middleware. We are also interested in providing an example with App Directory.
FAQs
Unleash SDK for Next.js
The npm package @unleash/nextjs receives a total of 34,502 weekly downloads. As such, @unleash/nextjs popularity was classified as popular.
We found that @unleash/nextjs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.