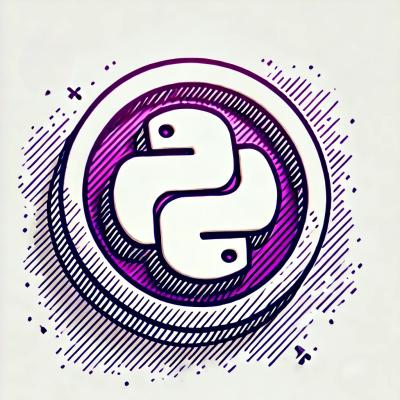
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
@unleash/proxy-client-react
Advanced tools
@unleash/proxy-client-react is a React client for the Unleash feature management system. It allows developers to easily integrate feature toggles into their React applications, enabling dynamic feature management and experimentation.
Initialize Unleash Client
This code initializes the Unleash client with the necessary configuration, including the URL of the Unleash API, the client key, and the application name. The `start` method is called to begin fetching feature toggles.
import { UnleashClient } from '@unleash/proxy-client-react';
const client = new UnleashClient({
url: 'https://app.unleash-hosted.com/demo/api/',
clientKey: 'proxy-client-key',
appName: 'my-react-app'
});
client.start();
Use Feature Toggles in Components
This code demonstrates how to use the `useFlag` hook to check the status of a feature toggle within a React component. Depending on whether the feature toggle is enabled or disabled, different content is rendered.
import { useFlag } from '@unleash/proxy-client-react';
const MyComponent = () => {
const isEnabled = useFlag('my-feature-toggle');
return (
<div>
{isEnabled ? <p>Feature is enabled</p> : <p>Feature is disabled</p>}
</div>
);
};
Custom Context Provider
This code shows how to wrap your application with the `UnleashProvider` to provide the Unleash client configuration context to all components within the application. This makes it easier to use feature toggles throughout the app.
import { UnleashProvider } from '@unleash/proxy-client-react';
const App = () => (
<UnleashProvider
config={{
url: 'https://app.unleash-hosted.com/demo/api/',
clientKey: 'proxy-client-key',
appName: 'my-react-app'
}}
>
<MyComponent />
</UnleashProvider>
);
react-feature-toggles is a lightweight library for managing feature toggles in React applications. It provides a simple API for defining and using feature toggles, but it does not offer the same level of integration with a feature management system like Unleash.
launchdarkly-react-client-sdk is the official React SDK for LaunchDarkly, a feature management platform. It offers similar functionality to @unleash/proxy-client-react, including feature flag evaluation and context management, but it is tied to the LaunchDarkly service.
react-ab-test is a library for A/B testing in React applications. It allows developers to define experiments and variants, and track user interactions. While it focuses on A/B testing rather than feature toggles, it provides similar capabilities for experimentation.
This library is meant to be used with the unleash-proxy. The proxy application layer will sit between your unleash instance and your client applications, and provides performance and security benefits. DO NOT TRY to connect this library directly to the unleash instance, as the datasets follow different formats because the proxy only returns evaluated toggle information.
npm install @unleash/proxy-client-react
// or
yarn add @unleash/proxy-client-react
Import the provider like this in your entrypoint file (typically index.js/ts):
import FlagProvider from '@unleash/proxy-client-react';
const config = {
url: 'https://HOSTNAME/api/proxy',
clientKey: 'PROXYKEY',
refreshInterval: 15,
appName: 'your-app-name',
environment: 'dev',
};
ReactDOM.render(
<React.StrictMode>
<FlagProvider config={config}>
<App />
</FlagProvider>
</React.StrictMode>,
document.getElementById('root')
);
To check if a feature is enabled:
import { useFlag } from '@unleash/proxy-client-react';
const TestComponent = () => {
const enabled = useFlag('travel.landing');
if (enabled) {
return <SomeComponent />
}
return <AnotherComponent />
};
export default TestComponent;
To check variants:
import { useVariant } from '@unleash/proxy-client-react';
const TestComponent = () => {
const variant = useVariant('travel.landing');
if (variant.enabled && variant.name === "SomeComponent") {
return <SomeComponent />
} else if (variant.enabled && variant.name === "AnotherComponent") {
return <AnotherComponent />
}
return <DefaultComponent />
};
export default TestComponent;
Follow the following steps in order to update the unleash context:
import { useUnleashContext, useFlag } from '@unleash/proxy-client-react'
const MyComponent = ({ userId }) => {
const variant = useFlag("my-toggle");
const updateContext = useUnleashContext();
useEffect(() => {
// context is updated with userId
updateContext({ userId })
}, [])
}
FAQs
React interface for working with unleash
The npm package @unleash/proxy-client-react receives a total of 98,909 weekly downloads. As such, @unleash/proxy-client-react popularity was classified as popular.
We found that @unleash/proxy-client-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.