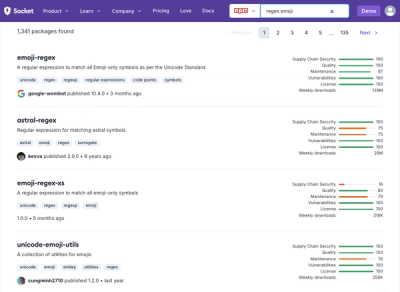
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
@unumid/library-crypto
Advanced tools
A helper library for common Unum ID cryptographic functions in TypeScript.
This library is available from NPM, Github packages or the repository itself.
Releases and publishing to NPM is automated via Github Actions CI job. In order to trigger a release one should push a git tag with a preceding v
with semver notation, ie v1.1.1
, to the main
branch. This will trigger the CI job to bump the package version, generate typedocs, publish to NPM, make a release commit, and make a Github Release. The contents of the Github Release are autogenerated based on pull requests with commits associated with the release, so please use PRs to makes changes to main
. The message of the git tag will be the commit message for the release so please make it meaningful. For example, git tag v1.1.1 -m "Updated the SDK with a new CI job" && push origin v1.1.1
.
This readme and the auto generated typedocs serve as the official documentation.
This latest version of the crypto library only interfaces with byte arrays, specifically Uint8Array's, to remove the string encoding unknowns when dealing with cryptographic outputs from multiple platforms, (i.e. Android, Web, etc).
In order to ensure a deterministic byte array cross platforms Protocol Buffers are highly recommend as the means of going to and from byte arrays. All "protobuf" objects come with built encoding and decoding helpers to assist.
Generates secp256r1
private and public keys.
(encoding: 'base58' | 'pem' = 'pem') => Promise<{ id: string, privateKey: string; publicKey: string}>;
import { generateEccKeyPair } from 'library-crypto-typescript';
// using async/await
const { id, privateKey, publicKey } = await generateEccKeyPair();
// using a promise
generateEccKeyPair().then(({ id, privateKey, publicKey }) => {
// do stuff
});
Generates RSA
private and public keys.
(encoding: 'base58' | 'pem' = 'pem') => Promise<{ id: string, privateKey: string; publicKey: string}>
import { generateRsaKeyPair } from 'library-crypto-typescript';
// using async/await
const { id, privateKey, publicKey } = await generateRsaKeyPair();
// using a promise
generateRsaKeyPair().then(({ id, privateKey, publicKey }) => {
// do stuff
});
Signs bytes with a secp256r1
private key.
(data: Uint8Array, privateKey: string) => string;
import { generateEccKeyPair, signBytes } from 'library-crypto-typescript';
const { privateKey } = await generateEccKeyPair();
const data: UnsignedString = {
data: 'Hello World'
};
const dataBytes = UnsignedString.encode(data).finish();
const signature = signBytes(dataBytes, privateKey);
Verifies a signature with a secp256r1
private key using the corresponding public key.
(signature: string, data: Uint8Array, publicKey: PublicKeyInfo) => boolean;
import { generateEccKeyPair, signBytes, verifyBytes } from 'library-crypto-typescript';
const { privateKey, publicKey } = await generateEccKeyPair();
const data: UnsignedString = {
data: 'Hello World'
};
const dataBytes = UnsignedString.encode(data).finish();
const signature = signBytes(dataBytes, privateKey);
const publicKeyInfo: PublicKeyInfo = {
publicKey,
encoding: 'pem'
}
const isValid = verifyBytes(signature, dataBytes, publicKeyInfo);
Encrypts data with a single-use AES key. Returns an object contianing the encrypted data encoded as a base64 string along with information about the AES key, encrypted with an RSA public key and encoded as base64 strings
(
did: string,
publicKeyInfo: PublicKeyInfo,
data: Uint8Array
) => { data: string, key: { iv: string, key: string, algorithm: string, did: string } };
iv
, key
, and algorithm
import { generateRsaKeyPair, encryptBytes } from 'library-crypto-typescript';
const { publicKey } = await generateRsaKeyPair();
const publicKeyInfo: PublicKeyInfo = {
publicKey,
encoding: 'pem'
}
const data: UnsignedString = {
data: 'Hello World'
};
const dataBytes = UnsignedString.encode(data).finish();
const encryptedData = encryptBytes(did, dataBytes, publicKeyInfo);
Decrypts data encrypted with an RSA
public key using the corresponding private key.
(
privateKey: string,
encryptedData: { data: string, key: { iv: string, key: string, algorithm: string, did: string } }
) => any;
encryptedData
import { generateRsaKeyPair, encryptBytes, decryptBytes } from 'library-crypto-typescript';
const { privateKey, publicKey } = await generateRsaKeyPair();
const publicKeyInfo: PublicKeyInfo = {
publicKey,
encoding: 'pem'
}
const data: UnsignedString = {
data: 'Hello World'
};
const dataBytes = UnsignedString.encode(data).finish();
const encryptedData = encryptBytes(did, publicKeyInfo, data);
const decryptedData = decryptBytes(privateKey, encryptedData);
FAQs
A TypeScript library for Unum ID crypto operations
We found that @unumid/library-crypto demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.