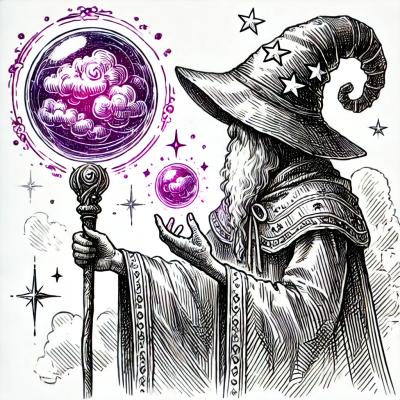
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@valerii-zinchenko/observer
Advanced tools
Simple observer to work with events. It can be used as a stand-alone observer or can be mixed in in some other object/function/class to directly reuse the functionality of an observer there.
All attached event handlers are stored in FIFO order. They are stored as is, i.e. if binding to some context is required then it should be done manually.
It is written on vanilla JavaScript :)
It does not require any additional libraries.
$ npm install valerii-zinchenko/observer --save
$ grunt build
Available library files:
dest/observer.js
- not minified librarydest/observer.min.js
- minified libraryThe destination library files are surrounded with the universal module definition. So it can be loaded
Observer
Simple event with property
// start listen 'hi' event by constructing a new instance
var observer = new Observer('hi', function(name) {
console.log('hi ' + name);
});
// start listen 'hi' event with a new handler function
observer.listen('hi', function(name) {
console.log('hi ' + name + ' again');
});
observer.trigger('hi', 'world');
// The followwing lines will be logged in the console.
// 'hi world'
// 'hi world again'
Example of encapsulating of Observer functionality into a class, by using a class-wrapper.
// Define classes
// --------------------
// Human class, that can call a cat and receive an answer
var Human = Class(function() {
// listen an "answer" event, i.e. an answer from someone
this.listen('answer', this.onAnswer);
}, {
Encapsulate: Observer,
// call a cat
callCat: function(cat) {
cat.trigger('call', this);
},
// handle an answer from a cat
onAnswer: function(answer) {
console.log(answer);
}
});
// Cat class, that listens any calls and answers to the caller
var Cat = Class(function() {
// listen "call" event, i.e. someone will call a cat
this.listen('call', this.onCall);
}, {
Encapsulate: Observer,
// handle a "call" event
onCall: function(initiator) {
// answer to an initiator
initiator.trigger('answer', 'Meow! :P');
}
});
// --------------------
// Create instances
// --------------------
var human = new Human();
var cat = new Cat();
// --------------------
// some human calls some cat
human.callCat(cat);
// The following line will be logged into the console
// 'Meow! :P'
FAQs
Simple tool to work with events.
The npm package @valerii-zinchenko/observer receives a total of 0 weekly downloads. As such, @valerii-zinchenko/observer popularity was classified as not popular.
We found that @valerii-zinchenko/observer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.