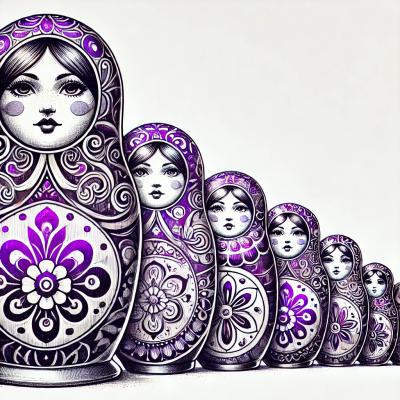
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
@vercel/speed-insights
Advanced tools
Speed Insights is a tool for measuring web performance and providing suggestions for improvement.
@vercel/speed-insights is an npm package designed to provide insights into the performance of web applications. It leverages Google's Lighthouse to analyze and generate reports on various performance metrics, helping developers optimize their applications for better speed and user experience.
Generate Performance Report
This feature allows you to generate a performance report for a given URL. The `getSpeedInsights` function fetches the performance metrics and returns a detailed report.
const { getSpeedInsights } = require('@vercel/speed-insights');
async function generateReport(url) {
const report = await getSpeedInsights({ url });
console.log(report);
}
generateReport('https://example.com');
Custom Configuration
This feature allows you to customize the performance report by specifying options such as the strategy (mobile or desktop) and the categories of metrics to include in the report.
const { getSpeedInsights } = require('@vercel/speed-insights');
async function generateCustomReport(url) {
const options = {
strategy: 'mobile',
categories: ['performance', 'accessibility']
};
const report = await getSpeedInsights({ url, options });
console.log(report);
}
generateCustomReport('https://example.com');
Save Report to File
This feature allows you to save the generated performance report to a file. The `fs` module is used to write the report data to a specified file path.
const { getSpeedInsights } = require('@vercel/speed-insights');
const fs = require('fs');
async function saveReportToFile(url, filePath) {
const report = await getSpeedInsights({ url });
fs.writeFileSync(filePath, JSON.stringify(report, null, 2));
console.log(`Report saved to ${filePath}`);
}
saveReportToFile('https://example.com', './report.json');
Lighthouse is an open-source, automated tool for improving the quality of web pages. It can be run as a Chrome Extension or from the command line. It audits performance, accessibility, progressive web apps, SEO, and more. Compared to @vercel/speed-insights, Lighthouse offers a broader range of audits and can be integrated into various CI/CD pipelines.
psi (PageSpeed Insights) is a Node.js module for Google's PageSpeed Insights API. It provides performance reports and suggestions for improving the speed of web pages. While @vercel/speed-insights focuses on Lighthouse metrics, psi directly interfaces with the PageSpeed Insights API, offering a different set of performance insights.
web-vitals is a small, modular library for measuring all the Web Vitals metrics on real users. It is designed to be used in production environments to capture real user performance data. Unlike @vercel/speed-insights, which generates synthetic performance reports, web-vitals focuses on real user monitoring (RUM) to provide insights based on actual user interactions.
@vercel/speed-insights
automatically tracks web vitals and other performance metrics for your website.
This package does not track data in development mode.
It has 1st class integration with:
Framework | Package |
---|---|
Next.js | @vercel/speed-insights/next |
Nuxt | @vercel/speed-insights/nuxt |
Sveltekit | @vercel/speed-insights/sveltekit |
React | @vercel/speed-insights/react |
Astro | @vercel/speed-insights/astro |
Vue | @vercel/speed-insights/vue |
It also supports other frameworks, vanilla JS and static websites.
Enable Vercel Speed Insights for a project in the Vercel Dashboard.
Add the @vercel/speed-insights
package to your project
Inject Speed Insights to your app
<SpeedInsights />
component to inject the script into your app.injectSpeedInsights()
function @vercel/speed-insights/sveltekit
in your top-level +layout.js/ts
file.inject
function add the tracking script to your app.Deploy your app to Vercel and see data flowing in.
Find more details about this package in our documentation.
FAQs
Speed Insights is a tool for measuring web performance and providing suggestions for improvement.
The npm package @vercel/speed-insights receives a total of 270,586 weekly downloads. As such, @vercel/speed-insights popularity was classified as popular.
We found that @vercel/speed-insights demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.